Python中的逻辑门 – 一份适合初学者的指南
本文全面介绍了Python中的不同逻辑门。逻辑门是实现数字元件的最基本材料。逻辑门的应用范围从计算机架构扩展到电子领域。
这些门处理二进制值,即0或1。不同类型的门需要不同数量的输入,但它们都提供一个单一的输出。当这些逻辑门组合在一起时,形成了复杂的电路。
让我们尝试在Python语言中实现逻辑门。
在Python中的基本逻辑门
电路开发中有三种最基本的逻辑门。
或门
这个门当两个输入中的任意一个为1时提供输出为1。它与二进制数的“加法”操作类似。
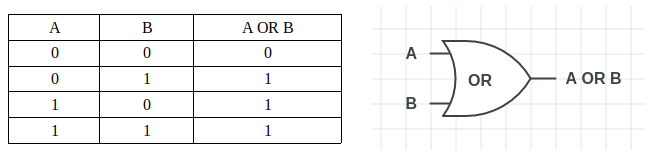
上方展示的是真值表,用于展示“或”门输入值的所有组合。表格旁边的图示代表一个“或”门。
在Python中实现。
# Function to simulate OR Gate
def OR(A, B):
return A | B
print("Output of 0 OR 0 is", OR(0, 0))
print("Output of 0 OR 1 is", OR(0, 1))
print("Output of 1 OR 0 is", OR(1, 0))
print("Output of 1 OR 1 is", OR(1, 1))
我们得到了如下的输出。
Output of 0 OR 0 is 0
Output of 0 OR 1 is 1
Output of 1 OR 0 is 1
Output of 1 OR 1 is 1
与门
这个门的输出为0,只要其中一个输入为0。在二进制数字中,这个操作被视为乘法。
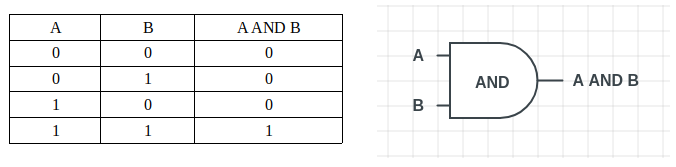
在真值表中可以看到,只要两个输入中的任一输入为0,输出也是0。旁边的图表示了“与门”。
Python 实施方法:
# Function to simulate AND Gate
def AND(A, B):
return A & B
print("Output of 0 AND 0 is", AND(0, 0))
print("Output of 0 AND 1 is", AND(0, 1))
print("Output of 1 AND 0 is", AND(1, 0))
print("Output of 1 AND 1 is", AND(1, 1))
輸出:
Output of 0 AND 0 is 0
Output of 0 AND 1 is 0
Output of 1 AND 0 is 0
Output of 1 AND 1 is 1
非门
这个门提供了输入的否定。这个门只支持一个输入。

上述表格清楚地显示了位的反转。相邻的图形代表了“非”门。
二进制非门的python实现。
# Function to simulate NOT Gate
def NOT(A):
return ~A+2
print("Output of NOT 0 is", NOT(0))
print("Output of NOT 1 is", NOT(1))
输出:
Output of NOT 0 is 1
Output of NOT 1 is 0
注意:’NOT()’函数对于位值0和1提供准确的结果。
Python中的通用逻辑门
存在两个通用逻辑门,即“与非”门和“或非”门。它们被称为通用逻辑门,因为任何布尔电路都可以仅使用这些门来实现。
非门
“NAND” 门是由 “AND”门和 “NOT” 门组合而成的。与 “AND” 门相反,当两个位都设置时,它只提供输出为0,否则为1。
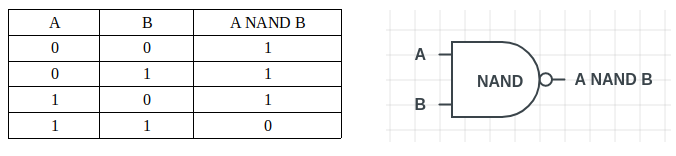
在Python中,可以使用之前创建的’AND()’和’OR()’函数来实现’NAND()’函数。
# Function to simulate AND Gate
def AND(A, B):
return A & B;
# Function to simulate NOT Gate
def NOT(A):
return ~A+2
# Function to simulate NAND Gate
def NAND(A, B):
return NOT(AND(A, B))
print("Output of 0 NAND 0 is", NAND(0, 0))
print("Output of 0 NAND 1 is", NAND(0, 1))
print("Output of 1 NAND 0 is", NAND(1, 0))
print("Output of 1 NAND 1 is", NAND(1, 1))
我们得到了以下输出。
Output of 0 NAND 0 is 1
Output of 0 NAND 1 is 1
Output of 1 NAND 0 is 1
Output of 1 NAND 1 is 0
与门 (Yǔ mén)
“NOR”门是通过级联“OR”门和“NOT”门得到的结果。与“OR”门相反,当所有输入都为0时,它输出为1。
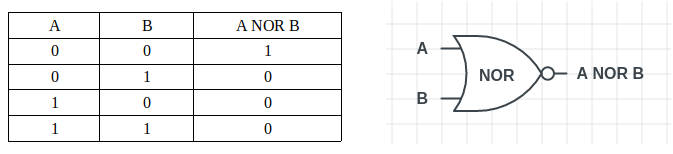
类似于”NAND()”函数,可以使用已经创建的函数来实现”NOR()”。
# Function to calculate OR Gate
def OR(A, B):
return A | B;
# Function to simulate NOT Gate
def NOT(A):
return ~A+2
# Function to simulate NOR Gate
def NOR(A, B):
return NOT(OR(A, B))
print("Output of 0 NOR 0 is", NOR(0, 0))
print("Output of 0 NOR 1 is", NOR(0, 1))
print("Output of 1 NOR 0 is", NOR(1, 0))
print("Output of 1 NOR 1 is", NOR(1, 1))
输出结果:
Output of 0 NOR 0 is 1
Output of 0 NOR 1 is 0
Output of 1 NOR 0 is 0
Output of 1 NOR 1 is 0
Python中的独家逻辑门
有两种特殊类型的逻辑门,XOR门和XNOR门,它们关注的是输入为0或1的数量,而不是各个值。
异或门
独占或门(’XOR’)代表Exclusive-OR的首字母缩写,在输入中的1的数量为奇数时提供一个输出为1。
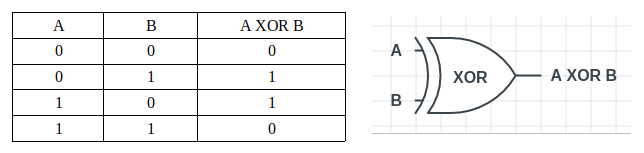
我们可以清楚地看到上表中异或门的输出。当输入中的1的数量为1时(即奇数),它会提供一个输出为1。
我们可以轻松地在Python中实现’XOR()’函数,方法如下:
# Function to simulate XOR Gate
def XOR(A, B):
return A ^ B
print("Output of 0 XOR 0 is", XOR(0, 0))
print("Output of 0 XOR 1 is", XOR(0, 1))
print("Output of 1 XOR 0 is", XOR(1, 0))
print("Output of 1 XOR 1 is", XOR(1, 1))
我们获得了如下的输出结果。
Output of 0 XOR 0 is 0
Output of 0 XOR 1 is 1
Output of 1 XOR 0 is 1
Output of 1 XOR 1 is 0
XNOR门
它是由“XOR”门和“NOT”门的组合而形成的。与“XOR”相反,在输入中的1的数量为偶数时,它提供1的输出。
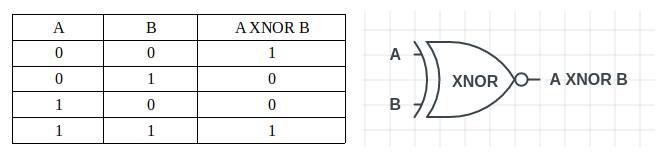
在Python中,可以通过使用’XOR()’和’NOT()’函数来实现’XNOR()’函数。
# Function to simulate XOR Gate
def XOR(A, B):
return A ^ B
# Function to simulate NOT Gate
def NOT(A):
return ~A+2
# Function to simulate XNOR Gate
def XNOR(A, B):
return NOT(XOR(A, B))
print("Output of 0 XNOR 0 is", XNOR(0, 0))
print("Output of 0 XNOR 1 is", XNOR(0, 1))
print("Output of 1 XNOR 0 is", XNOR(1, 0))
print("Output of 1 XNOR 1 is", XNOR(1, 1))
输出:
Output of 0 XNOR 0 is 1
Output of 0 XNOR 1 is 0
Output of 1 XNOR 0 is 0
Output of 1 XNOR 1 is 1
结论
使用Python实现逻辑门非常容易。作为一名程序员,你需要了解Python中的逻辑门和运算符。我们希望这篇文章能让读者对Python中逻辑门的基础知识和执行方法有更清晰的认识。
如需进一步阅读,请查阅我们的其他Python教程。