NumPy 矩阵乘法
NumPy矩阵乘法可以通过以下三种方法进行。
- multiply():逐元素矩阵相乘。
matmul():两个数组的矩阵乘积。
dot():两个数组的点积。
1. NumPy 矩阵逐元素相乘
如果你想进行逐元素矩阵乘法,你可以使用multiply()函数。
import numpy as np
arr1 = np.array([[1, 2],
[3, 4]])
arr2 = np.array([[5, 6],
[7, 8]])
arr_result = np.multiply(arr1, arr2)
print(arr_result)
输出结果:
[[ 5 12]
[21 32]]
下面的图片显示了执行乘法运算以得到结果矩阵。
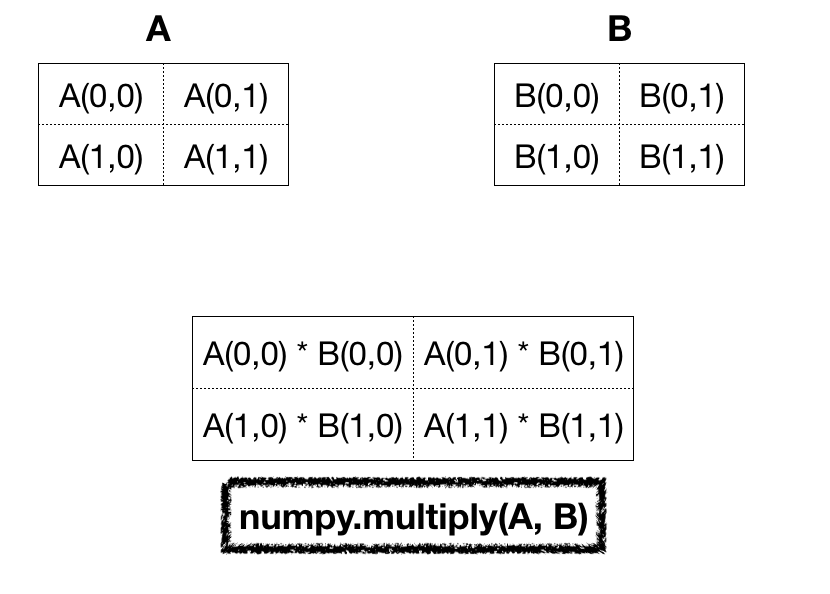
2. 两个NumPy数组的矩阵乘积
如果你想要两个数组的矩阵乘积,请使用matmul()函数。
import numpy as np
arr1 = np.array([[1, 2],
[3, 4]])
arr2 = np.array([[5, 6],
[7, 8]])
arr_result = np.matmul(arr1, arr2)
print(f'Matrix Product of arr1 and arr2 is:\n{arr_result}')
arr_result = np.matmul(arr2, arr1)
print(f'Matrix Product of arr2 and arr1 is:\n{arr_result}')
输出:
Matrix Product of arr1 and arr2 is:
[[19 22]
[43 50]]
Matrix Product of arr2 and arr1 is:
[[23 34]
[31 46]]
下图解释了结果数组中每个索引的矩阵乘法操作。为简单起见,取第一个数组的行和第二个数组的列,然后将对应的元素相乘并相加,得出矩阵乘积的值。
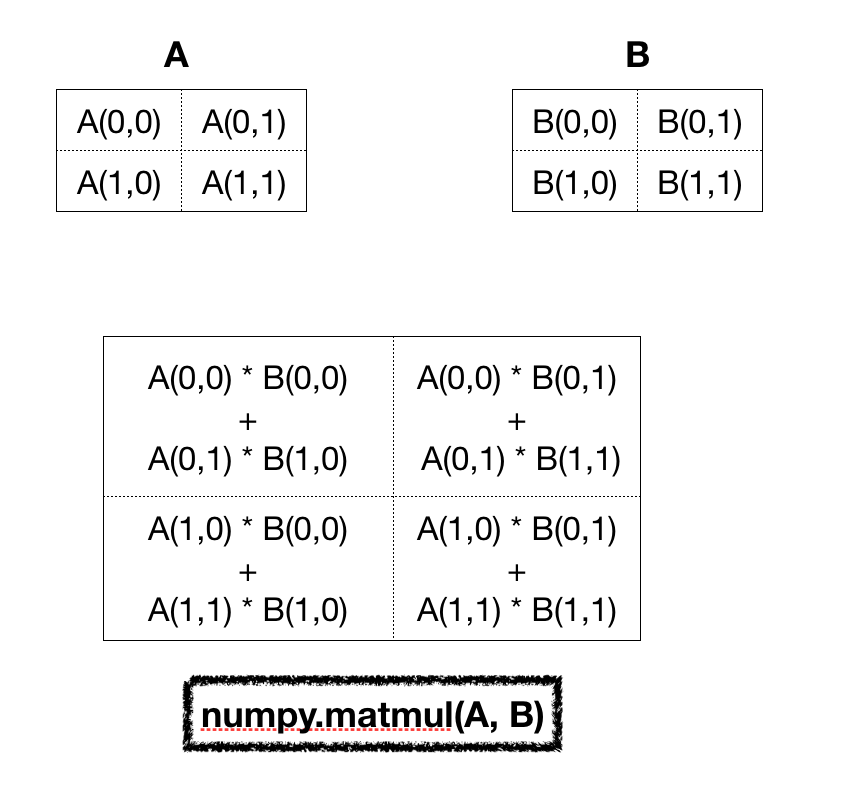
两个数组的矩阵乘积取决于参数的位置。因此,matmul(A, B)可能与matmul(B, A)不同。
3. 两个NumPy数组的点积
numpy的dot()函数返回两个数组的点乘结果。对于一维和二维数组,该结果与matmul()函数相同。
import numpy as np
arr1 = np.array([[1, 2],
[3, 4]])
arr2 = np.array([[5, 6],
[7, 8]])
arr_result = np.dot(arr1, arr2)
print(f'Dot Product of arr1 and arr2 is:\n{arr_result}')
arr_result = np.dot(arr2, arr1)
print(f'Dot Product of arr2 and arr1 is:\n{arr_result}')
arr_result = np.dot([1, 2], [5, 6])
print(f'Dot Product of two 1-D arrays is:\n{arr_result}')
输出:只需要一种选项
Dot Product of arr1 and arr2 is:
[[19 22]
[43 50]]
Dot Product of arr2 and arr1 is:
[[23 34]
[31 46]]
Dot Product of two 1-D arrays is:
17
推荐阅读:
- numpy.square()
- NumPy sqrt() – Square Root of Matrix Elements
- Python NumPy Tutorial
参考文献
- numpy matmul()
- numpy multiply()