Java ListIterator – Java中的列表迭代器
我们知道Java有四个游标:Enumeration,Iterator,ListIterator和Spliterator。我们已经在之前的帖子中讨论了Enumeration和Iterator游标。在阅读本帖子之前,请先阅读我的上一篇帖子:Java Iterator。在本文中,我们将讨论第三个Java游标:ListIterator。
Java的ListIterator
和Iterator一样,ListIterator是Java中的一个迭代器,用于逐个访问实现了List接口的对象中的元素。
- It is available since Java 1.2.
- It extends Iterator interface.
- It is useful only for List implemented classes.
- Unlike Iterator, It supports all four operations: CRUD (CREATE, READ, UPDATE and DELETE).
- Unlike Iterator, It supports both Forward Direction and Backward Direction iterations.
- It is a Bi-directional Iterator.
- It has no current element; its cursor position always lies between the element that would be returned by a call to previous() and the element that would be returned by a call to next().
请解释集合API中的CRUD操作是什么?
- CREATE: Adding new elements to Collection object.
- READ: Retrieving elements from Collection object.
- UPDATE: Updating or setting existing elements in Collection object.
- DELETE: Removing elements from Collection object.
Java ListIterator 类的类图
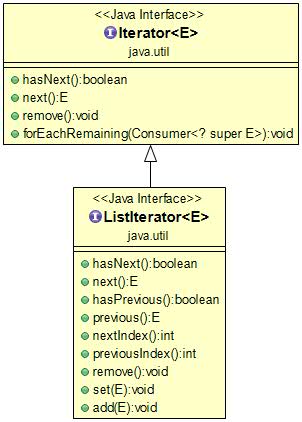
Java ListIterator 方法
Java ListIterator 接口具有以下方法。
- void add(E e): Inserts the specified element into the list.
- boolean hasNext(): Returns true if this list iterator has more elements when traversing the list in the forward direction.
- boolean hasPrevious(): Returns true if this list iterator has more elements when traversing the list in the reverse direction.
- E next(): Returns the next element in the list and advances the cursor position.
- int nextIndex(): Returns the index of the element that would be returned by a subsequent call to next().
- E previous(): Returns the previous element in the list and moves the cursor position backwards.
- int previousIndex(): Returns the index of the element that would be returned by a subsequent call to previous().
- void remove(): Removes from the list the last element that was returned by next() or previous().
- void set(E e): Replaces the last element returned by next() or previous() with the specified element.
在接下来的部分中,我们将一一探讨这些方法,并举出有用的例子。
Java ListIterator 基本示例
在本节中,我们将讨论一些带有示例的ListIterator方法。首先,我们需要了解如何获取此迭代器对象。怎么获取ListIterator呢?
ListIterator<E> listIterator()
这将返回一个列表迭代器,用于遍历此列表中的各个元素。例如:
import java.util.*;
public class ListIteratorDemo
{
public static void main(String[] args)
{
List<String> names = new LinkedList<>();
names.add("Rams");
names.add("Posa");
names.add("Chinni");
// Getting ListIterator
ListIterator<String> namesIterator = names.listIterator();
// Traversing elements
while(namesIterator.hasNext()){
System.out.println(namesIterator.next());
}
// Enhanced for loop creates Internal Iterator here.
for(String name: names){
System.out.println(name);
}
}
}
产出:
Rams
Posa
Chinni
双向迭代示例:ListIterator
在本节中,我们将探索ListIterator的方法如何用于执行正向迭代和反向迭代。
import java.util.*;
public class BiDirectinalListIteratorDemo
{
public static void main(String[] args)
{
List<String> names = new LinkedListt<>();
names.add("Rams");
names.add("Posa");
names.add("Chinni");
// Getting ListIterator
ListIterator<String> listIterator = names.listIterator();
// Traversing elements
System.out.println("Forward Direction Iteration:");
while(listIterator.hasNext()){
System.out.println(listIterator.next());
}
// Traversing elements, the iterator is at the end at this point
System.out.println("Backward Direction Iteration:");
while(listIterator.hasPrevious()){
System.out.println(listIterator.previous());
}
}
}
输出:
Forward Direction Iteration:
Rams
Posa
Chinni
Backward Direction Iteration:
Chinni
Posa
Rams
Java 迭代器的类型
正如我们所知,Java有四个游标:Enumeration、Iterator、ListIterator和Spliterator。我们可以将它们分为两种主要类型,如下所示:
- Uni-Directional Iterators
They are Cursors which supports only Forward Direction iterations. For instance, Enumeration, Iterator, etc. are Uni-Directional Iterators.- Bi-Directional Iterators
They are Cursors which supports Both Forward Direction and Backward Direction iterations. For instance, ListIterator is Bi-Directional Iterator.
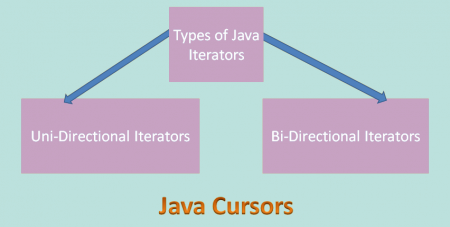
Java ListIterator的内部工作原理是什么?
正如我们所知,Java的ListIterator既可以在正向方向上工作,也可以在反向方向上工作。它是一个双向迭代器。为了支持这个功能,它有两组方法。
- Forward Direction Iteration methods
We need to use the following methods to support Forward Direction Iteration:
- hasNext() – 是否有下一个元素
next() – 返回下一个元素
nextIndex() – 返回下一个元素的索引
- Backward Direction Iteration methods
We need to use the following methods to support Backward Direction Iteration:
- 有前一个吗?(hasPrevious())
前一个 (previous())
前一个索引 (previousIndex())
在我之前的帖子中,我们已经讨论过如何在向前的方向上内部运作的迭代器,即“Java迭代器的内部工作原理”部分。即使ListIterator也是以相同的方式工作。如果您想查看我之前的帖子,请点击这里:Java迭代器。在这一部分,我们将讨论ListIterator在向后方向上的工作原理。让我们拿以下的LinkedList对象来理解这个功能。
List<String> names = new LinkedList<>();
names.add("E-1");
names.add("E-2");
names.add("E-3");
.
.
.
names.add("E-n");
现在在LinkedList上创建一个ListIterator对象,如下所示:
ListIterator<String> namesIterator = names.listLterator();
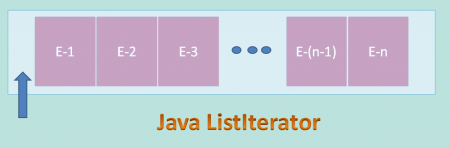
namesIterator.hasNext();
namesIterator.next();
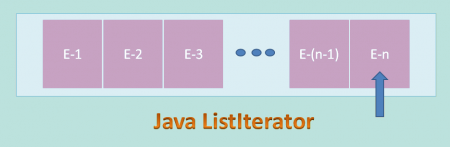
namesIterator.hasPrevious();
namesIterator.previous();
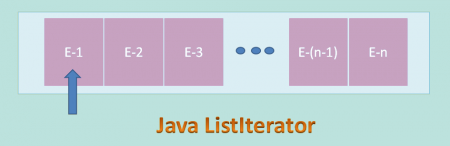
namesIterator.hasPrevious();
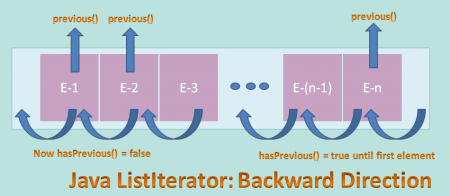
ListIterator的优势
与迭代器不同,列表迭代器具有以下优点:
- Like Iterator, it supports READ and DELETE operations.
- It supports CREATE and UPDATE operations too.
- That means, it supports CRUD operations: CREATE, READ, UPDATE and DELETE operations.
- It supports both Forward direction and Backward direction iteration. That means it’s a Bi-Directional Java Cursor.
- Method names are simple and easy to use them.
ListIterator的限制
与迭代器相比,Java的ListIterator具有许多优点。然而,它仍然有以下一些限制。
- It is an Iterator only List implementation classes.
- Unlike Iterator, it is not applicable for whole Collection API.
- It is not a Universal Java Cursor.
- Compare to Spliterator, it does NOT support Parallel iteration of elements.
- Compare to Spliterator, it does NOT support better performance to iterate large volume of data.
迭代器和列表迭代器之间的相似之处
在这一部分中,我们将讨论Java两个游标之间的相似之处:迭代器和列表迭代器。
- Bother are introduced in Java 1.2.
- Both are Iterators used to iterate Collection or List elements.
- Both supports READ and DELETE operations.
- Both supports Forward Direction iteration.
- Both are not Legacy interfaces.
迭代器和列表迭代器之间的区别
在这一部分中,我们将讨论Java两种迭代器:Iterator和ListIterator之间的差异。
Iterator | ListIterator |
---|---|
Introduced in Java 1.2. | Introduced in Java 1.2. |
It is an Iterator for whole Collection API. | It is an Iterator for only List implemented classes. |
It is an Universal Iterator. | It is NOT an Universal Iterator. |
It supports only Forward Direction Iteration. | It supports both Forward and Backward Direction iterations. |
It’s a Uni-Directional Iterator. | It’s a Bi-Directional Iterator. |
It supports only READ and DELETE operations. | It supports all CRUD operations. |
We can get Iterator by using iterator() method. | We can ListIterator object using listIterator() method. |
这就是关于Java中ListIterator的全部内容。希望这些Java ListIterator的理论和例子能帮助你开始ListIterator编程。参考文献:ListIterator API文档。