在Android中使用JSONObject解析JSON数据
Android中的JSONObject用于在android应用中进行JSON解析。在本教程中,我们将讨论并在我们的android应用中实现一个JSONObject来解析JSON数据。JSON代表JavaScript对象表示法。
JSON是什么?
JSON用于服务器之间的数据交换(发布和检索)。因此,了解其语法和可用性非常重要。JSON是XML的最佳替代方案,而且更易读。JSON是与语言无关的。由于具有语言无关性,我们可以使用任何语言(如Java/C/C++)来编程JSON。服务器返回的JSON响应由许多字段组成。下面是一个示例JSON响应/数据。我们将把它用作参考并在我们的应用程序中实现。
{
"title":"JSONParserTutorial",
"array":[
{
"company":"Google"
},
{
"company":"Facebook"
},
{
"company":"LinkedIn"
},
{
"company" : "Microsoft"
},
{
"company": "Apple"
}
],
"nested":{
"flag": true,
"random_number":1
}
}
我们从这个页面生成了一个随机的JSON数据字符串。它非常方便用于编辑JSON数据。一个JSON数据由下面列出的4个主要组成部分构成。
- 数组:一个JSONArray被括在方括号([)中。它包含一组对象。
对象:被大括号({)括起来的数据是一个单独的JSONObject。嵌套的JSON对象是可能的,并且非常常用。
键:每个JSONObject都有一个包含特定值的键字符串。
值:每个键都有一个单一的值,可以是任意类型的字符串、双精度浮点数、整数、布尔值等等。
安卓的 JSONObject
我们将从上述给定的静态JSON数据字符串中创建一个JSONObject,并在ListView中显示JSONArray。我们将把应用程序名称更改为JSON数据中的标题字符串。
在安卓中的JSON解析示例
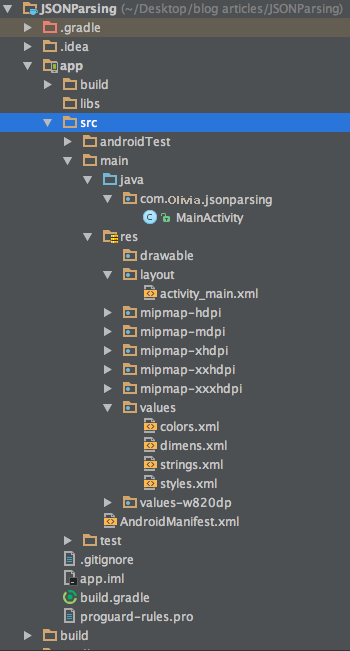
Android JSON解析代码
以下是activity_main.xml文件的内容。
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:tools="https://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.Olivia.jsonparsing.MainActivity">
<ListView
android:layout_width="wrap_content"
android:id="@+id/list_view"
android:layout_height="match_parent"/>
</RelativeLayout>
下面是MainActivity.java的代码。
package com.Olivia.jsonparsing;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
String json_string = "{\n" +
" \"title\":\"JSONParserTutorial\",\n" +
" \"array\":[\n" +
" {\n" +
" \"company\":\"Google\"\n" +
" },\n" +
" {\n" +
" \"company\":\"Facebook\"\n" +
" },\n" +
" {\n" +
" \"company\":\"LinkedIn\"\n" +
" },\n" +
" {\n" +
" \"company\" : \"Microsoft\"\n" +
" },\n" +
" {\n" +
" \"company\": \"Apple\"\n" +
" }\n" +
" ],\n" +
" \"nested\":{\n" +
" \"flag\": true,\n" +
" \"random_number\":1\n" +
" }\n" +
"}";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
try {
ListView listView = (ListView) findViewById(R.id.list_view);
List<String> items = new ArrayList<>();
JSONObject root = new JSONObject(json_string);
JSONArray array= root.getJSONArray("array");
this.setTitle(root.getString("title"));
for(int i=0;i<array.length();i++)
{
JSONObject object= array.getJSONObject(i);
items.add(object.getString("company"));
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1, items);
if (listView != null) {
listView.setAdapter(adapter);
}
JSONObject nested= root.getJSONObject("nested");
Log.d("TAG","flag value "+nested.getBoolean("flag"));
} catch (JSONException e) {
e.printStackTrace();
}
}
}
我们已经遍历了JSONArray对象,并提取了每个子JSONObject中存在的字符串,并将它们添加到一个ArrayList中,该ArrayList在ListView中显示。使用以下方式更改应用程序名称:
this.setTitle();
Android中JSONObject示例输出
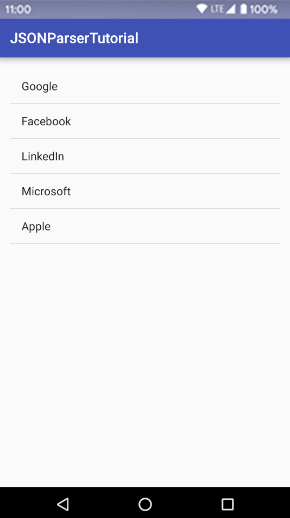