Using Kotlin Android SharedPreferences
In this tutorial, we will explore how to use SharedPreferences in our Android app by employing Kotlin.
What does Android SharedPreferences refer to?
SharedPreferences has been a part of the Android API since API level 1. It serves as an interface for storing, modifying, and deleting data locally. Usually, it is employed for caching user-specific data like login forms. This data is stored in a key-value format and it is possible to have several files for holding SharedPreferences data.
Option 1:
Methods of SharedPreferences
Let us examine some crucial techniques for SharedPreferences.
- getSharedPreferences(String, int) method is used to retrieve an instance of the SharedPreferences. Here String is the name of the SharedPreferences file and int is the Context passed.
- The SharedPreferences.Editor() is used to edit values in the SharedPreferences.
- We can call commit() or apply() to save the values in the SharedPreferences file. The commit() saves the values immediately whereas apply() saves the values asynchronously.
Using Kotlin, the values of SharedPreferences can be set and retrieved.
We have the ability to assign values to our SharedPreference instance using Kotlin by following this approach.
val sharedPreference = getSharedPreferences("PREFERENCE_NAME",Context.MODE_PRIVATE)
var editor = sharedPreference.edit()
editor.putString("username","Anupam")
editor.putLong("l",100L)
editor.commit()
When it comes to obtaining a value:
sharedPreference.getString("username","defaultName")
sharedPreference.getLong("l",1L)
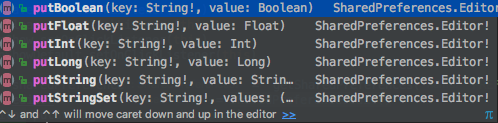
One option for paraphrasing the given sentence natively could be:
Kotlin code that can be used to clear and delete the stored records in SharedPreferences.
By using the clear() and remove(String key) methods, we have the option to either remove a specific value or clear all the values.
editor.clear()
editor.remove("username")
The modifications made to the editor after the commit or apply are not taken into account. The method described above for saving and retrieving values from a SharedPreference is very similar to what we do in Java. So, where does Kotlin offer its unique benefits? We will explore this by looking at an example Android application.
The project structure of an Android Kotlin project that utilizes SharedPreferences.
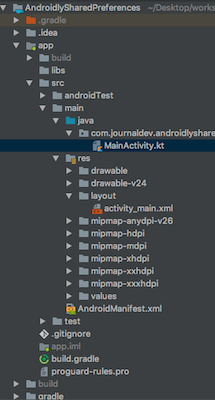
1. Code for the design structure.
Here is the code for the activity_main.xml layout file.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/inUserId"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:hint="User ID"
android:inputType="number" />
<EditText
android:id="@+id/inPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/inUserId"
android:hint="Password"
android:inputType="textPassword" />
<Button
android:id="@+id/btnSave"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/inPassword"
android:text="SAVE USER DATA" />
<Button
android:id="@+id/btnClear"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_below="@+id/btnSave"
android:text="CLEAR USER DATA" />
<Button
android:id="@+id/btnShow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_below="@+id/inPassword"
android:text="SHOW" />
<Button
android:id="@+id/btnShowDefault"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:layout_below="@+id/btnSave"
android:text="Show Default" />
</RelativeLayout>
The Kotlin code for the main activity.
Here is the code for the Kotlin class MainActivity.kt.
package com.scdev.androidlysharedpreferences
import android.content.Context
import android.content.SharedPreferences
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.preference.PreferenceManager
import android.view.View
import com.scdev.androidlysharedpreferences.PreferenceHelper.defaultPreference
import com.scdev.androidlysharedpreferences.PreferenceHelper.password
import com.scdev.androidlysharedpreferences.PreferenceHelper.userId
import com.scdev.androidlysharedpreferences.PreferenceHelper.clearValues
import com.scdev.androidlysharedpreferences.PreferenceHelper.customPreference
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity(), View.OnClickListener {
val CUSTOM_PREF_NAME = "User_data"
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
btnSave.setOnClickListener(this)
btnClear.setOnClickListener(this)
btnShow.setOnClickListener(this)
btnShowDefault.setOnClickListener(this)
}
override fun onClick(v: View?) {
val prefs = customPreference(this, CUSTOM_PREF_NAME)
when (v?.id) {
R.id.btnSave -> {
prefs.password = inPassword.text.toString()
prefs.userId = inUserId.text.toString().toInt()
}
R.id.btnClear -> {
prefs.clearValues
}
R.id.btnShow -> {
inUserId.setText(prefs.userId.toString())
inPassword.setText(prefs.password)
}
R.id.btnShowDefault -> {
val defaultPrefs = defaultPreference(this)
inUserId.setText(defaultPrefs.userId.toString())
inPassword.setText(defaultPrefs.password)
}
}
}
}
object PreferenceHelper {
val USER_ID = "USER_ID"
val USER_PASSWORD = "PASSWORD"
fun defaultPreference(context: Context): SharedPreferences = PreferenceManager.getDefaultSharedPreferences(context)
fun customPreference(context: Context, name: String): SharedPreferences = context.getSharedPreferences(name, Context.MODE_PRIVATE)
inline fun SharedPreferences.editMe(operation: (SharedPreferences.Editor) -> Unit) {
val editMe = edit()
operation(editMe)
editMe.apply()
}
var SharedPreferences.userId
get() = getInt(USER_ID, 0)
set(value) {
editMe {
it.putInt(USER_ID, value)
}
}
var SharedPreferences.password
get() = getString(USER_PASSWORD, "")
set(value) {
editMe {
it.putString(USER_PASSWORD, value)
}
}
var SharedPreferences.clearValues
get() = { }
set(value) {
editMe {
it.clear()
}
}
}
With the help of Kotlin Android Extensions, we can avoid using findViewById for each XML view. In the provided code, we are generating a singleton class using the object keyword. A higher-order function named editMe() is declared as an inline function, which contains the logic for the edit operation. We have defined individual properties for each value. The use of get and set Kotlin properties allows us to fetch and modify the data in the shared preferences. Kotlin has significantly reduced the verbosity of the code, resulting in a cleaner appearance. Moreover, we can enhance its conciseness by utilizing another Kotlin higher-order function demonstrated below.
fun SharedPreferences.Editor.put(pair: Pair<String, Any>) {
val key = pair.first
val value = pair.second
when(value) {
is String -> putString(key, value)
is Int -> putInt(key, value)
is Boolean -> putBoolean(key, value)
is Long -> putLong(key, value)
is Float -> putFloat(key, value)
else -> error("Only primitive types can be stored in SharedPreferences")
}
And while assigning the values, we carry out the following actions.
var SharedPreferences.password
get() = getString(USER_PASSWORD, "")
set(value) {
editMe {
it.put(USER_PASSWORD to value)
}
}
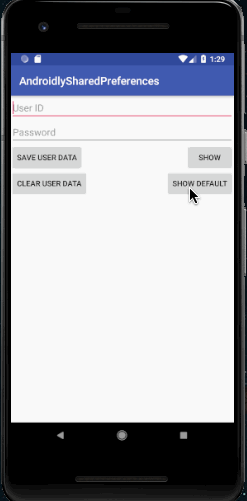
You are able to obtain the source code by clicking on the provided link: AndroidlySharedPreferences.
More tutorials
A class in Kotlin that is sealed.(Opens in a new browser tab)
How can you determine the standard deviation in R?(Opens in a new browser tab)
get pandas DataFrame from an API endpoint that lacks order?(Opens in a new browser tab)
JSON fundamentals(Opens in a new browser tab)
BroadcastReceiver Example Tutorial on Android(Opens in a new browser tab)