A class in Kotlin that is sealed.
In this tutorial, we will explore Kotlin Sealed Class, its definition, and its purpose. All of these aspects will be discussed in the following sections.
A Kotlin Sealed Class
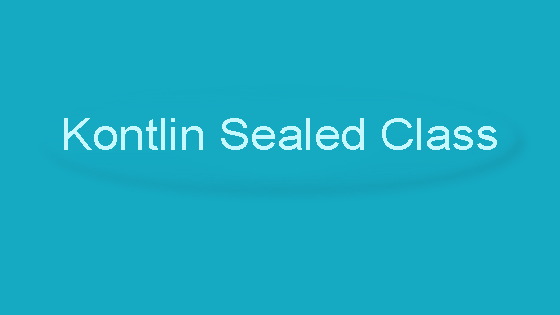
Utilizing Kotlin Sealed Classes in practice.
In Kotlin, the implementation of sealed classes is done as follows.
sealed class A{
class B : A()
class C : A()
}
In order to define a sealed class, the sealed modifier must be added. Sealed classes cannot be created or instantiated, thus making them implicitly abstract. The following example will not function as intended.
fun main(args: Array<String>)
{
var a = A() //compiler error. Class A cannot be instantiated.
}
By default, the constructors of a sealed class are private. All subclasses of a sealed class need to be declared in the same file. Sealed classes are crucial for maintaining type safety as they limit the available types during compile-time exclusively.
sealed class A{
class B : A()
{
class E : A() //this works.
}
class C : A()
init {
println("sealed class A")
}
}
class D : A() //this works
{
class F: A() //This won't work. Since sealed class is defined in another scope.
}
Building a sealed class by incorporating constructors.
sealed class A(var name: String){
class B : A("B")
class C : A("C")
}
class D : A("D")
fun main(args: Array<String>) {
var b = A.B()
var d = D()
}
One possible paraphrase could be:
Incorporating Data Class and Object within a sealed class.
fun main(args: Array<String>) {
val e = A.E("Anupam")
println(e) //prints E(name=Anupam)
var d = A.D
d.name() //prints Object D
}
sealed class A{
class B : A()
class C : A()
object D : A()
{
fun name()
{
println("Object D")
}
}
data class E(var name: String) : A()
}
What is the distinction between enum and sealed classes?
Sealed classes in Kotlin can be thought of as enhanced versions of Enum classes. Unlike Enums, which only allow us to use the same type for all enum constants, sealed classes enable us to create instances with different types. Thus, the capability described above is not achievable with Enum classes.
enum class Months(string: String){
January("Jan"), February(2),
}
Sealed classes come to our aid in situations where we need multiple instances, unlike enum classes which only allow a single type for all constants.
sealed class Months {
class January(var shortHand: String) : Months()
class February(var number: Int) : Months()
class March(var shortHand: String, var number: Int) : Months()
}
In your Projects, how can you incorporate this Sealed classes feature? For example, in an application similar to a newsfeed, you can generate three distinct class categories for posts: Status, Image, and Video.
sealed class Post
{
class Status(var text: String) : Post()
class Image(var url: String, var caption: String) : Post()
class Video(var url: String, var timeDuration: Int, var encoding: String): Post()
}
It is not feasible to accomplish this using Enum classes.
Sealed classes and when statements are the same.
When using sealed classes, it is typical to incorporate them alongside when statements as each subclass and their respective types function as cases. Additionally, the use of sealed classes restricts the available types. As a result, removing the else portion of the when statement becomes a straightforward task. The following example illustrates this concept.
sealed class Shape{
class Circle(var radius: Float): Shape()
class Square(var length: Int): Shape()
class Rectangle(var length: Int, var breadth: Int): Shape()
}
fun eval(e: Shape) =
when (e) {
is Shape.Circle -> println("Circle area is ${3.14*e.radius*e.radius}")
is Shape.Square -> println("Square area is ${e.length*e.length}")
is Shape.Rectangle -> println("Rectagle area is ${e.length*e.breadth}")
}
We need to execute the eval function within our main function as demonstrated below.
fun main(args: Array<String>) {
var circle = Shape.Circle(4.5f)
var square = Shape.Square(4)
var rectangle = Shape.Rectangle(4,5)
eval(circle)
eval(square)
eval(rectangle)
//eval(x) //compile-time error.
}
//Following is printed on the console:
//Circle area is 63.585
//Square area is 16
//Rectangle area is 20
Please note that the “is” modifier is used to check if a class belongs to a specific type. However, this modifier is only necessary for classes and not for Kotlin objects, as demonstrated in the example below.
sealed class Shape{
class Circle(var radius: Float): Shape()
class Square(var length: Int): Shape()
object Rectangle: Shape()
{
var length: Int = 0
var breadth : Int = 0
}
}
fun eval(e: Shape) =
when (e) {
is Shape.Circle -> println("Circle area is ${3.14*e.radius*e.radius}")
is Shape.Square -> println("Square area is ${e.length*e.length}")
Shape.Rectangle -> println("Rectangle area is ${Shape.Rectangle.length*Shape.Rectangle.breadth}")
}
The Kotlin sealed class tutorial has reached its conclusion. Check out the Kotlin Docs for further references.
more tutorials
get pandas DataFrame from an API endpoint that lacks order?(Opens in a new browser tab)
init or Swift initialization in Swift(Opens in a new browser tab)
The Command design pattern.(Opens in a new browser tab)
Spring Component annotation(Opens in a new browser tab)
Progress Bar iOS also known as Progress View(Opens in a new browser tab)