BroadcastReceiver Example Tutorial on Android
Today, we will be talking about and utilizing the Android BroadcastReceiver, which plays a crucial role in the Android Framework.
A native paraphrase of “Android BroadcastReceiver” could be “Receiver in Android for handling broadcast events.”
An Android BroadcastReceiver is a passive element of the Android system that monitors system-wide broadcast events or intents. Once any of these events take place, it triggers an action in the application, such as displaying a notification on the status bar or performing a specific task. Unlike activities, Android BroadcastReceiver does not include any graphical user interface. Generally, the broadcast receiver is used to assign tasks to services based on the type of intent data received. The subsequent intents listed are a few examples of significant system-wide generated intents.
-
- android.intent.action.BATTERY_LOW: Indicates that the device’s battery is running low.
-
- android.intent.action.BOOT_COMPLETED: Broadcasted once after the system has completed booting up.
-
- android.intent.action.CALL: Initiates a phone call to the specified recipient.
-
- android.intent.action.DATE_CHANGED: Indicates that the date has been changed.
-
- android.intent.action.REBOOT: Initiates a system reboot on the device.
- android.net.conn.CONNECTIVITY_CHANGE: Indicates a change or reset in the mobile network or Wi-Fi connection.
Android’s Broadcast Receiver
To configure a Broadcast Receiver in an android application, there are two essential steps that must be undertaken.
-
- Writing a BroadcastReceiver
- Enabling a BroadcastReceiver
Designing a BroadcastReceiver
Let us swiftly execute a personalized BroadcastReceiver as depicted beneath.
public class MyReceiver extends BroadcastReceiver {
public MyReceiver() {
}
@Override
public void onReceive(Context context, Intent intent) {
Toast.makeText(context, "Action: " + intent.getAction(), Toast.LENGTH_SHORT).show();
}
}
A BroadcastReceiver is a class that has an abstract method called onReceiver(). This method is invoked on registered Broadcast Receivers when an event takes place. Along with the additional data, an intent object is passed. A Context object is also accessible, which can be used to initiate an activity or service using context.startActivity(myIntent); or context.startService(myService); respectively.
One possible paraphrase:
Adding the BroadcastReceiver to the android application.
There are two methods to register a BroadcastReceiver.
- By specifying it in the AndroidManifest.xml file in the manner demonstrated below.
<receiver android:name=".ConnectionReceiver" >
<intent-filter>
<action android:name="android.net.conn.CONNECTIVITY_CHANGE" />
</intent-filter>
</receiver>
By specifying intent filters, we instruct the system to send any intent that matches our subelements to the designated broadcast receiver. An example of registering a broadcast receiver programmatically is demonstrated in the provided code snippet.
IntentFilter filter = new IntentFilter();
intentFilter.addAction(getPackageName() + "android.net.conn.CONNECTIVITY_CHANGE");
MyReceiver myReceiver = new MyReceiver();
registerReceiver(myReceiver, filter);
To remove a broadcast receiver from onStop() or onPause() of the activity, you can utilize the following code snippet.
@Override
protected void onPause() {
unregisterReceiver(myReceiver);
super.onPause();
}
One possible paraphrased option could be: “The Activity is responsible for transmitting Broadcast intents.”
This code snippet is utilized for dispatching an intention to all the associated BroadcastReceivers.
Intent intent = new Intent();
intent.setAction("com.scdev.CUSTOM_INTENT");
sendBroadcast(intent);
Make sure to include the mentioned action in the intent filter section of the manifest or programmatically. We will create an app that detects network changes and custom intents, and then handles the data accordingly.
In the structure of an Android project, the BroadcastReceiver is present.
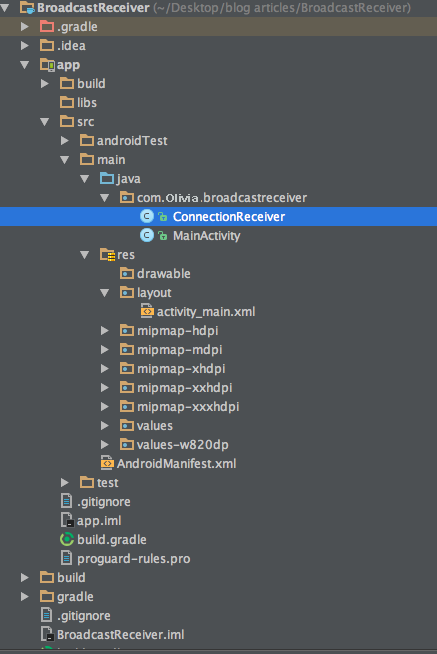
One potential option for paraphrasing this phrase natively could be:
Code for Android BroadcastReceiver
In the center of the activity_main.xml, there is a button that is responsible for sending a broadcast intention.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:tools="https://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.scdev.broadcastreceiver.MainActivity">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/button"
android:text="Send Broadcast"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
</RelativeLayout>
Here is the code for MainActivity.java.
package com.scdev.broadcastreceiver;
import android.content.Intent;
import android.content.IntentFilter;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import butterknife.ButterKnife;
import butterknife.InjectView;
import butterknife.OnClick;
public class MainActivity extends AppCompatActivity {
ConnectionReceiver receiver;
IntentFilter intentFilter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.inject(this);
receiver = new ConnectionReceiver();
intentFilter = new IntentFilter("com.scdev.broadcastreceiver.SOME_ACTION");
}
@Override
protected void onResume() {
super.onResume();
registerReceiver(receiver, intentFilter);
}
@Override
protected void onDestroy() {
super.onDestroy();
unregisterReceiver(receiver);
}
@OnClick(R.id.button)
void someMethod() {
Intent intent = new Intent("com.scdev.broadcastreceiver.SOME_ACTION");
sendBroadcast(intent);
}
}
We have programmatically added another custom action in the code mentioned above. The definition of the ConnectionReceiver can be found in the AndroidManifest.xml file, as stated below.
<?xml version="1.0" encoding="utf-8"?>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="https://schemas.android.com/apk/res/android"
package="com.scdev.broadcastreceiver">
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<receiver android:name=".ConnectionReceiver">
<intent-filter>
<action android:name="android.net.conn.CONNECTIVITY_CHANGE" />
</intent-filter>
</receiver>
</application>
</manifest>
Below is the definition of the class ConnectionReceiver in the Java file ConnectionReceiver.java.
public class ConnectionReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
Log.d("API123",""+intent.getAction());
if(intent.getAction().equals("com.scdev.broadcastreceiver.SOME_ACTION"))
Toast.makeText(context, "SOME_ACTION is received", Toast.LENGTH_LONG).show();
else {
ConnectivityManager cm =
(ConnectivityManager) context.getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo activeNetwork = cm.getActiveNetworkInfo();
boolean isConnected = activeNetwork != null &&
activeNetwork.isConnectedOrConnecting();
if (isConnected) {
try {
Toast.makeText(context, "Network is connected", Toast.LENGTH_LONG).show();
} catch (Exception e) {
e.printStackTrace();
}
} else {
Toast.makeText(context, "Network is changed or reconnected", Toast.LENGTH_LONG).show();
}
}
}
}
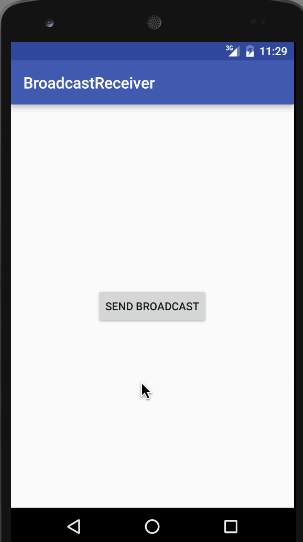
Get the Android BroadcastReceiver Project for download.
Read more tutor from us:
convert string to character array in Java.(Opens in a new browser tab)
Tutorial on how to set up a Hibernate Tomcat JNDI DataSource.(Opens in a new browser tab)