MongoDB批量插入 – MongoDB insertMany
今天我们将研究MongoDB批量插入。在MongoDB中,可以使用批量插入操作一次插入多个文档,其中将文档数组作为参数传递给插入方法。
MongoDB 批量插入
默认情况下,MongoDB批量插入按顺序进行插入。如果在某一点的插入过程中发生错误,则不会继续插入剩余的文档。让我们通过命令行来看一个使用MongoDB批量插入来插入多个文档的示例。
MongoDB批量插入多个文档
> db.car.insert(
... [
... { _id:1,name:"Audi",color:"Red",cno:"H101",mfdcountry:"Germany",speed:75 },
... { _id:2,name:"Swift",color:"Black",cno:"H102",mfdcountry:"Italy",speed:60 },
... { _id:3,name:"Maruthi800",color:"Blue",cno:"H103",mfdcountry:"India",speed:70 },
... { _id:4,name:"Polo",color:"White",cno:"H104",mfdcountry:"Japan",speed:65 },
... { _id:5,name:"Volkswagen",color:"JetBlue",cno:"H105",mfdcountry:"Rome",speed:80 }
... ]
... )
BulkWriteResult({
"writeErrors" : [ ],
"writeConcernErrors" : [ ],
"nInserted" : 5,
"nUpserted" : 0,
"nMatched" : 0,
"nModified" : 0,
"nRemoved" : 0,
"upserted" : [ ]
})
执行了这个操作后,共插入了五个文档。如果用户在查询中没有指定id字段,MongoDB将自动创建该字段。”nInserted”列告诉用户被插入的文档数量。要查看已插入的文档,请执行如下所示的查询。
> db.car.find()
{ "_id" : 1, "name" : "Audi", "color" : "Red", "cno" : "H101", "mfdcountry" : "Germany", "speed" : 75 }
{ "_id" : 2, "name" : "Swift", "color" : "Black", "cno" : "H102", "mfdcountry" : "Italy", "speed" : 60 }
{ "_id" : 3, "name" : "Maruthi800", "color" : "Blue", "cno" : "H103", "mfdcountry" : "India", "speed" : 70 }
{ "_id" : 4, "name" : "Polo", "color" : "White", "cno" : "H104", "mfdcountry" : "Japan", "speed" : 65 }
{ "_id" : 5, "name" : "Volkswagen", "color" : "JetBlue", "cno" : "H105", "mfdcountry" : "Rome", "speed" : 80 }
>
阅读有关 MongoDB 的查找和插入操作的更多信息。在插入时,用户不必在查询中提供所有字段。现在让我们看看当未指定一些字段时插入是如何工作的。
使用MongoDB批量插入文档时,可以指定部分字段。
> db.car.insert(
... [
... { _id:6,name:"HondaCity",color:"Grey",cno:"H106",mfdcountry:"Sweden",speed:45 },
... {name:"Santro",color:"Pale Blue",cno:"H107",mfdcountry:"Denmark",speed:55 },
... { _id:8,name:"Zen",speed:54 }
... ]
... )
BulkWriteResult({
"writeErrors" : [ ],
"writeConcernErrors" : [ ],
"nInserted" : 3,
"nUpserted" : 0,
"nMatched" : 0,
"nModified" : 0,
"nRemoved" : 0,
"upserted" : [ ]
})
>
在这个例子中,对于第二个文档,用户没有指定id字段,而对于第三个文档,查询中只提供了id、name和speed字段。尽管第二个和第三个文档中缺少一些字段,但查询成功插入了文档。nInserted列显示插入了三个文档。调用find方法并检查插入的文档。
> db.car.find()
{ "_id" : 1, "name" : "Audi", "color" : "Red", "cno" : "H101", "mfdcountry" : "Germany", "speed" : 75 }
{ "_id" : 2, "name" : "Swift", "color" : "Black", "cno" : "H102", "mfdcountry" : "Italy", "speed" : 60 }
{ "_id" : 3, "name" : "Maruthi800", "color" : "Blue", "cno" : "H103", "mfdcountry" : "India", "speed" : 70 }
{ "_id" : 4, "name" : "Polo", "color" : "White", "cno" : "H104", "mfdcountry" : "Japan", "speed" : 65 }
{ "_id" : 5, "name" : "Volkswagen", "color" : "JetBlue", "cno" : "H105", "mfdcountry" : "Rome", "speed" : 80 }
{ "_id" : 6, "name" : "HondaCity", "color" : "Grey", "cno" : "H106", "mfdcountry" : "Sweden", "speed" : 45 }
{ "_id" : ObjectId("54885b8e61307aec89441a0b"), "name" : "Santro", "color" : "Pale Blue", "cno" : "H107", "mfdcountry" : "Denmark", "speed" : 55 }
{ "_id" : 8, "name" : "Zen", "speed" : 54 }
>
请注意,对于汽车“Santro”,id是由MongoDB自动生成的。对于id 8 – 只有名称和速度字段被插入。
插入无序的文件
在执行无序插入时,如果在某个点发生错误,MongoDB将继续插入数组中剩余的文档。例如;
> db.car.insert(
... [
... { _id:9,name:"SwiftDezire",color:"Maroon",cno:"H108",mfdcountry:"New York",speed:40 },
... { name:"Punto",color:"Wine Red",cno:"H109",mfdcountry:"Paris",speed:45 },
... ],
... { ordered: false }
... )
BulkWriteResult({
"writeErrors" : [ ],
"writeConcernErrors" : [ ],
"nInserted" : 2,
"nUpserted" : 0,
"nMatched" : 0,
"nModified" : 0,
"nRemoved" : 0,
"upserted" : [ ]
})
>
执行 db.car.find() 查询指定了有序的假数据,表明这是一个无序集合。
{ "_id" : 1, "name" : "Audi", "color" : "Red", "cno" : "H101", "mfdcountry" : "Germany", "speed" : 75 }
{ "_id" : 2, "name" : "Swift", "color" : "Black", "cno" : "H102", "mfdcountry" : "Italy", "speed" : 60 }
{ "_id" : 3, "name" : "Maruthi800", "color" : "Blue", "cno" : "H103", "mfdcountry" : "India", "speed" : 70 }
{ "_id" : 4, "name" : "Polo", "color" : "White", "cno" : "H104", "mfdcountry" : "Japan", "speed" : 65 }
{ "_id" : 5, "name" : "Volkswagen", "color" : "JetBlue", "cno" : "H105", "mfdcountry" : "Rome", "speed" : 80 }
{ "_id" : 6, "name" : "HondaCity", "color" : "Grey", "cno" : "H106", "mfdcountry" : "Sweden", "speed" : 45 }
{ "_id" : ObjectId("54746407d785e3a05a1808a6"), "name" : "Santro", "color" : "Pale Blue", "cno" : "H107", "mfdcountry" : "Denmark", "speed" : 55 }
{ "_id" : 8, "name" : "Zen", "speed" : 54 }
{ "_id" : 9, "name" : "SwiftDezire", "color" : "Maroon", "cno" : "H108", "mfdcountry" : "New York", "speed" : 40 }
{ "_id" : ObjectId("5474642dd785e3a05a1808a7"), "name" : "Punto", "color" : "Wine Red", "cno" : "H109", "mfdcountry" : "Paris", "speed" : 45 }
文件已插入,如您所见,这是一个无序插入。如果插入方法遇到错误,结果将包括“WriteResult.writeErrors”字段,指示导致失败的错误消息。
插入重复的id值 (Chārù chóngfù de id zhí)
> db.car.insert({_id:6,name:"Innova"})
WriteResult({
"nInserted" : 0,
"writeError" : {
"code" : 11000,
"errmsg" : "insertDocument :: caused by :: 11000 E11000 duplicate key error index: scdev.car.$_id_ dup key: { : 6.0 }"
}
})
>
错误表明我们正在插入一个已经包含文档的id为6的文档,因此对值为6的id抛出了重复键错误。
MongoDB的Bulk.insert()方法
这种方法可以批量进行插入操作。它从2.6版本开始引入。语法如下:Bulk.insert(
大批量无序插入
> var carbulk = db.car.initializeUnorderedBulkOp();
> carbulk.insert({ name:"Ritz", color:"Grey",cno:"H109",mfdcountry:"Mexico",speed:62});
> carbulk.insert({ name:"Versa", color:"Magenta",cno:"H110",mfdcountry:"France",speed:68});
> carbulk.insert({ name:"Innova", color:"JetRed",cno:"H111",mfdcountry:"Dubai",speed:72});
> carbulk.execute();
BulkWriteResult({
"writeErrors" : [ ],
"writeConcernErrors" : [ ],
"nInserted" : 3,
"nUpserted" : 0,
"nMatched" : 0,
"nModified" : 0,
"nRemoved" : 0,
"upserted" : [ ]
})
>
创建了一个名为carbulk的无序列表,并指定了要插入的字段和值的插入查询。请注意,在最后一个插入语句之后需要调用execute()方法,以确保数据实际被插入到数据库中。
MongoDB批量有序插入
这类似于无序批量插入,但我们使用 initializeOrderedBulkOp 调用。
>var car1bulk = db.car.initializeOrderedBulkOp();
>car1bulk.insert({ name:"Ertiga", color:"Red",cno:"H112",mfdcountry:"America",speed:65});
>car1bulk.insert({ name:"Quanta", color:"Maroon",cno:"H113",mfdcountry:"Rome",speed:78});
>car1bulk.execute();
BulkWriteResult({
"writeErrors" : [ ],
"writeConcernErrors" : [ ],
"nInserted" : 2,
"nUpserted" : 0,
"nMatched" : 0,
"nModified" : 0,
"nRemoved" : 0,
"upserted" : [ ]
})
首先,我们创建一个名为carbulk1的有序车辆集合列表,然后通过调用execute()方法插入文档。
MongoDB批量插入Java程序
让我们看一个使用Java程序来执行不同的批量操作的示例,这些操作我们之前使用Shell命令来完成。以下是使用MongoDB Java驱动程序版本2.x进行批量插入的Java程序。
package com.Olivia.mongodb;
import com.mongodb.BasicDBObject;
import com.mongodb.BulkWriteOperation;
import com.mongodb.BulkWriteResult;
import com.mongodb.DB;
import com.mongodb.DBCollection;
import com.mongodb.DBCursor;
import com.mongodb.DBObject;
import com.mongodb.MongoClient;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.List;
public class MongoDBBulkInsert {
//method that inserts all the documents
public static void insertmultipledocs() throws UnknownHostException{
//Get a new connection to the db assuming that it is running
MongoClient mongoClient = new MongoClient("localhost");
////use test as a datbase,use your database here
DB db=mongoClient.getDB("test");
////fetch the collection object ,car is used here,use your own
DBCollection coll = db.getCollection("car");
//create a new object
DBObject d1 = new BasicDBObject();
//data for object d1
d1.put("_id", 11);
d1.put("name","WagonR");
d1.put("color", "MetallicSilver");
d1.put("cno", "H141");
d1.put("mfdcountry","Australia");
d1.put("speed",66);
DBObject d2 = new BasicDBObject();
//data for object d2
d2.put("_id", 12);
d2.put("name","Xylo");
d2.put("color", "JetBlue");
d2.put("cno", "H142");
d2.put("mfdcountry","Europe");
d2.put("speed",69);
DBObject d3 = new BasicDBObject();
//data for object d3
d3.put("_id", 13);
d3.put("name","Alto800");
d3.put("color", "JetGrey");
d3.put("cno", "H143");
d3.put("mfdcountry","Austria");
d3.put("speed",74);
//create a new list
List<DBObject> docs = new ArrayList<>();
//add d1,d2 and d3 to list docs
docs.add(d1);
docs.add(d2);
docs.add(d3);
//insert list docs to collection
coll.insert(docs);
//stores the result in cursor
DBCursor carmuldocs = coll.find();
//print the contents of the cursor
try {
while(carmuldocs.hasNext()) {
System.out.println(carmuldocs.next());
}
} finally {
carmuldocs.close();//close the cursor
}
}
//method that inserts documents with some fields
public static void insertsomefieldsformultipledocs() throws UnknownHostException{
//Get a new connection to the db assuming that it is running
MongoClient mongoClient = new MongoClient("localhost");
////use test as a datbase,use your database here
DB db=mongoClient.getDB("test");
////fetch the collection object ,car is used here,use your own
DBCollection coll = db.getCollection("car");
//create object d1
DBObject d1 = new BasicDBObject();
//insert data for name,color and speed
d1.put("name","Indica");
d1.put("color", "Silver");
d1.put("cno", "H154");
DBObject d2 = new BasicDBObject();
//insert data for id,name and speed
d2.put("_id", 43);
d2.put("name","Astar");
d2.put("speed",79);
List<DBObject> docs = new ArrayList<>();
docs.add(d1);
docs.add(d2);
coll.insert(docs);
DBCursor carmuldocs = coll.find();
System.out.println("-----------------------------------------------");
try {
while(carmuldocs.hasNext()) {
System.out.println(carmuldocs.next());
}
} finally {
carmuldocs.close();//close the cursor
}
}
//method that checks for duplicate documents
public static void insertduplicatedocs() throws UnknownHostException{
//Get a new connection to the db assuming that it is running
MongoClient mongoClient = new MongoClient("localhost");
////use test as a datbase,use your database here
DB db=mongoClient.getDB("test");
////fetch the collection object ,car is used here,use your own
DBCollection coll = db.getCollection("car");
DBObject d1 = new BasicDBObject();
//insert duplicate data of id11
d1.put("_id", 11);
d1.put("name","WagonR-Lxi");
coll.insert(d1);
DBCursor carmuldocs = coll.find();
System.out.println("-----------------------------------------------");
try {
while(carmuldocs.hasNext()) {
System.out.println(carmuldocs.next());
}
} finally {
carmuldocs.close();//close the cursor
}
}
//method to perform bulk unordered list
public static void insertbulkunordereddocs() throws UnknownHostException{
//Get a new connection to the db assuming that it is running
MongoClient mongoClient = new MongoClient("localhost");
////use test as a datbase,use your database here
DB db=mongoClient.getDB("test");
////fetch the collection object ,car is used here,use your own
DBCollection coll = db.getCollection("car");
DBObject d1 = new BasicDBObject();
d1.put("name","Suzuki S-4");
d1.put("color", "Yellow");
d1.put("cno", "H167");
d1.put("mfdcountry","Italy");
d1.put("speed",54);
DBObject d2 = new BasicDBObject();
d2.put("name","Santro-Xing");
d2.put("color", "Cyan");
d2.put("cno", "H164");
d2.put("mfdcountry","Holand");
d2.put("speed",76);
//intialize and create a unordered bulk
BulkWriteOperation b1 = coll.initializeUnorderedBulkOperation();
//insert d1 and d2 to bulk b1
b1.insert(d1);
b1.insert(d2);
//execute the bulk
BulkWriteResult r1 = b1.execute();
DBCursor carmuldocs = coll.find();
System.out.println("-----------------------------------------------");
try {
while(carmuldocs.hasNext()) {
System.out.println(carmuldocs.next());
}
} finally {
carmuldocs.close();//close the cursor
}
}
//method that performs bulk insert for ordered list
public static void insertbulkordereddocs() throws UnknownHostException{
//Get a new connection to the db assuming that it is running
MongoClient mongoClient = new MongoClient("localhost");
////use test as a datbase,use your database here
DB db=mongoClient.getDB("test");
////fetch the collection object ,car is used here,use your own
DBCollection coll = db.getCollection("car");
DBObject d1 = new BasicDBObject();
d1.put("name","Palio");
d1.put("color", "Purple");
d1.put("cno", "H183");
d1.put("mfdcountry","Venice");
d1.put("speed",82);
DBObject d2 = new BasicDBObject();
d2.put("name","Micra");
d2.put("color", "Lime");
d2.put("cno", "H186");
d2.put("mfdcountry","Ethopia");
d2.put("speed",84);
//initialize and create ordered bulk
BulkWriteOperation b1 = coll.initializeOrderedBulkOperation();
b1.insert(d1);
b1.insert(d2);
//invoking execute
BulkWriteResult r1 = b1.execute();
DBCursor carmuldocs = coll.find();
System.out.println("-----------------------------------");
try {
while(carmuldocs.hasNext()) {
System.out.println(carmuldocs.next());
}
} finally {
carmuldocs.close();//close the cursor
}
}
public static void main(String[] args) throws UnknownHostException{
//invoke all the methods to perform insert operation
insertmultipledocs();
insertsomefieldsformultipledocs();
insertbulkunordereddocs();
insertbulkordereddocs();
insertduplicatedocs();
}
}
以上是上述程序的输出结果。
{ "_id" : 11 , "name" : "WagonR" , "color" : "MetallicSilver" , "cno" : "H141" , "mfdcountry" : "Australia" , "speed" : 66}
{ "_id" : 12 , "name" : "Xylo" , "color" : "JetBlue" , "cno" : "H142" , "mfdcountry" : "Europe" , "speed" : 69}
{ "_id" : 13 , "name" : "Alto800" , "color" : "JetGrey" , "cno" : "H143" , "mfdcountry" : "Austria" , "speed" : 74}
-----------------------------------------------
{ "_id" : 11 , "name" : "WagonR" , "color" : "MetallicSilver" , "cno" : "H141" , "mfdcountry" : "Australia" , "speed" : 66}
{ "_id" : 12 , "name" : "Xylo" , "color" : "JetBlue" , "cno" : "H142" , "mfdcountry" : "Europe" , "speed" : 69}
{ "_id" : 13 , "name" : "Alto800" , "color" : "JetGrey" , "cno" : "H143" , "mfdcountry" : "Austria" , "speed" : 74}
{ "_id" : { "$oid" : "548860e803649b8efac5a1d7"} , "name" : "Indica" , "color" : "Silver" , "cno" : "H154"}
{ "_id" : 43 , "name" : "Astar" , "speed" : 79}
-----------------------------------------------
{ "_id" : 11 , "name" : "WagonR" , "color" : "MetallicSilver" , "cno" : "H141" , "mfdcountry" : "Australia" , "speed" : 66}
{ "_id" : 12 , "name" : "Xylo" , "color" : "JetBlue" , "cno" : "H142" , "mfdcountry" : "Europe" , "speed" : 69}
{ "_id" : 13 , "name" : "Alto800" , "color" : "JetGrey" , "cno" : "H143" , "mfdcountry" : "Austria" , "speed" : 74}
{ "_id" : { "$oid" : "548860e803649b8efac5a1d7"} , "name" : "Indica" , "color" : "Silver" , "cno" : "H154"}
{ "_id" : 43 , "name" : "Astar" , "speed" : 79}
{ "_id" : { "$oid" : "548860e803649b8efac5a1d8"} , "name" : "Suzuki S-4" , "color" : "Yellow" , "cno" : "H167" , "mfdcountry" : "Italy" , "speed" : 54}
{ "_id" : { "$oid" : "548860e803649b8efac5a1d9"} , "name" : "Santro-Xing" , "color" : "Cyan" , "cno" : "H164" , "mfdcountry" : "Holand" , "speed" : 76}
-----------------------------------
{ "_id" : 11 , "name" : "WagonR" , "color" : "MetallicSilver" , "cno" : "H141" , "mfdcountry" : "Australia" , "speed" : 66}
{ "_id" : 12 , "name" : "Xylo" , "color" : "JetBlue" , "cno" : "H142" , "mfdcountry" : "Europe" , "speed" : 69}
{ "_id" : 13 , "name" : "Alto800" , "color" : "JetGrey" , "cno" : "H143" , "mfdcountry" : "Austria" , "speed" : 74}
{ "_id" : { "$oid" : "548860e803649b8efac5a1d7"} , "name" : "Indica" , "color" : "Silver" , "cno" : "H154"}
{ "_id" : 43 , "name" : "Astar" , "speed" : 79}
{ "_id" : { "$oid" : "548860e803649b8efac5a1d8"} , "name" : "Suzuki S-4" , "color" : "Yellow" , "cno" : "H167" , "mfdcountry" : "Italy" , "speed" : 54}
{ "_id" : { "$oid" : "548860e803649b8efac5a1d9"} , "name" : "Santro-Xing" , "color" : "Cyan" , "cno" : "H164" , "mfdcountry" : "Holand" , "speed" : 76}
{ "_id" : { "$oid" : "548860e803649b8efac5a1da"} , "name" : "Palio" , "color" : "Purple" , "cno" : "H183" , "mfdcountry" : "Venice" , "speed" : 82}
{ "_id" : { "$oid" : "548860e803649b8efac5a1db"} , "name" : "Micra" , "color" : "Lime" , "cno" : "H186" , "mfdcountry" : "Ethopia" , "speed" : 84}
Exception in thread "main" com.mongodb.MongoException$DuplicateKey: { "serverUsed" : "localhost:27017" , "ok" : 1 , "n" : 0 , "err" : "insertDocument :: caused by :: 11000 E11000 duplicate key error index: test.car.$_id_ dup key: { : 11 }" , "code" : 11000}
at com.mongodb.CommandResult.getWriteException(CommandResult.java:88)
at com.mongodb.CommandResult.getException(CommandResult.java:79)
at com.mongodb.DBCollectionImpl.translateBulkWriteException(DBCollectionImpl.java:314)
at com.mongodb.DBCollectionImpl.insert(DBCollectionImpl.java:189)
at com.mongodb.DBCollectionImpl.insert(DBCollectionImpl.java:165)
at com.mongodb.DBCollection.insert(DBCollection.java:93)
at com.mongodb.DBCollection.insert(DBCollection.java:78)
at com.mongodb.DBCollection.insert(DBCollection.java:120)
at com.Olivia.mongodb.MongoDBBulkInsert.insertduplicatedocs(MongoDBBulkInsert.java:163)
at com.Olivia.mongodb.MongoDBBulkInsert.main(MongoDBBulkInsert.java:304)
如果您使用的是MongoDB Java驱动程序3.x版本,则请使用下面的程序。
package com.Olivia.mongodb.main;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.List;
import org.bson.Document;
import com.mongodb.MongoClient;
import com.mongodb.client.FindIterable;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
public class MongoDBBulkInsert {
public static void main(String[] args) throws UnknownHostException {
// invoke all the methods to perform insert operation
insertmultipledocs();
insertsomefieldsformultipledocs();
insertduplicatedocs();
}
// method that inserts all the documents
public static void insertmultipledocs() throws UnknownHostException {
// Get a new connection to the db assuming that it is running
MongoClient mongoClient = new MongoClient("localhost");
//// use test as a database,use your database here
MongoDatabase db = mongoClient.getDatabase("test");
//// fetch the collection object ,car is used here,use your own
MongoCollection<Document> coll = db.getCollection("car");
// create a new object
Document d1 = new Document();
// data for object d1
d1.put("_id", 11);
d1.put("name", "WagonR");
d1.put("color", "MetallicSilver");
d1.put("cno", "H141");
d1.put("mfdcountry", "Australia");
d1.put("speed", 66);
Document d2 = new Document();
// data for object d2
d2.put("_id", 12);
d2.put("name", "Xylo");
d2.put("color", "JetBlue");
d2.put("cno", "H142");
d2.put("mfdcountry", "Europe");
d2.put("speed", 69);
Document d3 = new Document();
// data for object d3
d3.put("_id", 13);
d3.put("name", "Alto800");
d3.put("color", "JetGrey");
d3.put("cno", "H143");
d3.put("mfdcountry", "Austria");
d3.put("speed", 74);
// create a new list
List<Document> docs = new ArrayList<>();
// add d1,d2 and d3 to list docs
docs.add(d1);
docs.add(d2);
docs.add(d3);
// insert list docs to collection
coll.insertMany(docs);
// stores the result in cursor
FindIterable<Document> carmuldocs = coll.find();
for (Document d : carmuldocs)
System.out.println(d);
mongoClient.close();
}
// method that inserts documents with some fields
public static void insertsomefieldsformultipledocs() throws UnknownHostException {
// Get a new connection to the db assuming that it is running
MongoClient mongoClient = new MongoClient("localhost");
//// use test as a datbase,use your database here
MongoDatabase db = mongoClient.getDatabase("test");
//// fetch the collection object ,car is used here,use your own
MongoCollection<Document> coll = db.getCollection("car");
// create object d1
Document d1 = new Document();
// insert data for name,color and speed
d1.put("name", "Indica");
d1.put("color", "Silver");
d1.put("cno", "H154");
Document d2 = new Document();
// insert data for id,name and speed
d2.put("_id", 43);
d2.put("name", "Astar");
d2.put("speed", 79);
List<Document> docs = new ArrayList<>();
docs.add(d1);
docs.add(d2);
coll.insertMany(docs);
FindIterable<Document> carmuldocs = coll.find();
System.out.println("-----------------------------------------------");
for (Document d : carmuldocs)
System.out.println(d);
mongoClient.close();
}
// method that checks for duplicate documents
public static void insertduplicatedocs() throws UnknownHostException {
// Get a new connection to the db assuming that it is running
MongoClient mongoClient = new MongoClient("localhost");
//// use test as a database, use your database here
MongoDatabase db = mongoClient.getDatabase("test");
//// fetch the collection object ,car is used here,use your own
MongoCollection<Document> coll = db.getCollection("car");
Document d1 = new Document();
// insert duplicate data of id11
d1.put("_id", 11);
d1.put("name", "WagonR-Lxi");
coll.insertOne(d1);
FindIterable<Document> carmuldocs = coll.find();
System.out.println("-----------------------------------------------");
for (Document d : carmuldocs)
System.out.println(d);
mongoClient.close();
}
}
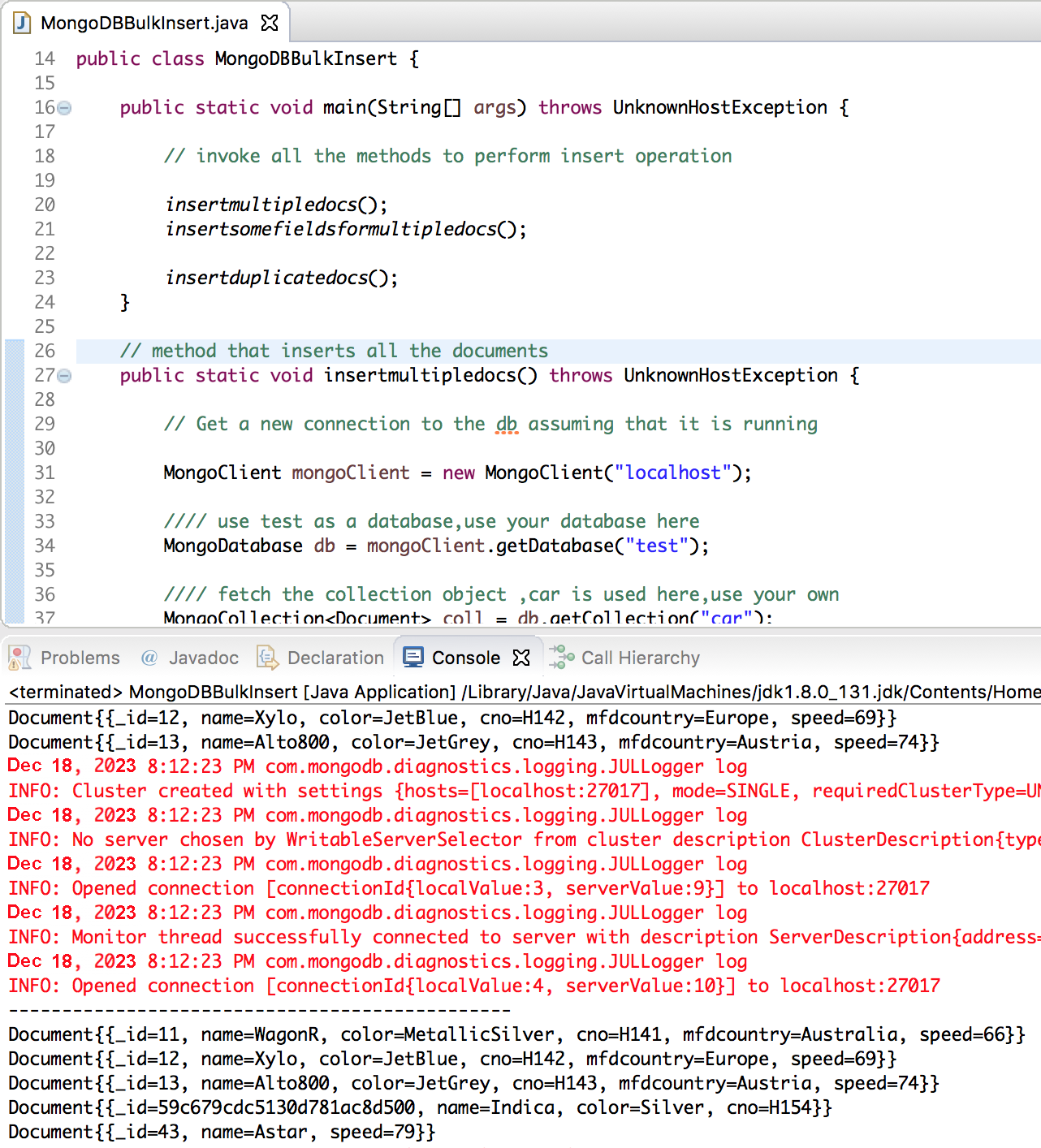