Android Material Components – 材料AlertDialog
Material Design 2.0 已经发布,我们迫不及待地想要使用 Dialogs。在本教程中,我们将在我们的 Android 应用程序中使用 Material 主题来自定义 Dialogs。
材料组件 – 对话框
警告对话框是应用程序的重要部分。通常用于引起用户对重要事项的注意。多亏了Material Design 2.0,现在我们有了更强大的对话框。首先,您需要添加材料组件的依赖项。
implementation 'com.google.android.material:material:1.1.0-alpha09'
在创建活动主题时,不要忘记继承MaterialComponent Theme或其后代。
基本实施
现在让我们使用建造者模式来创建一个基本的MaterialAlertDialog:
new MaterialAlertDialogBuilder(MainActivity.this)
.setTitle("Title")
.setMessage("Your message goes here. Keep it short but clear.")
.setPositiveButton("GOT IT", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
}
})
.setNegativeButton("CANCEL", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
}
})
.show();
在屏幕上看起来是这样的。
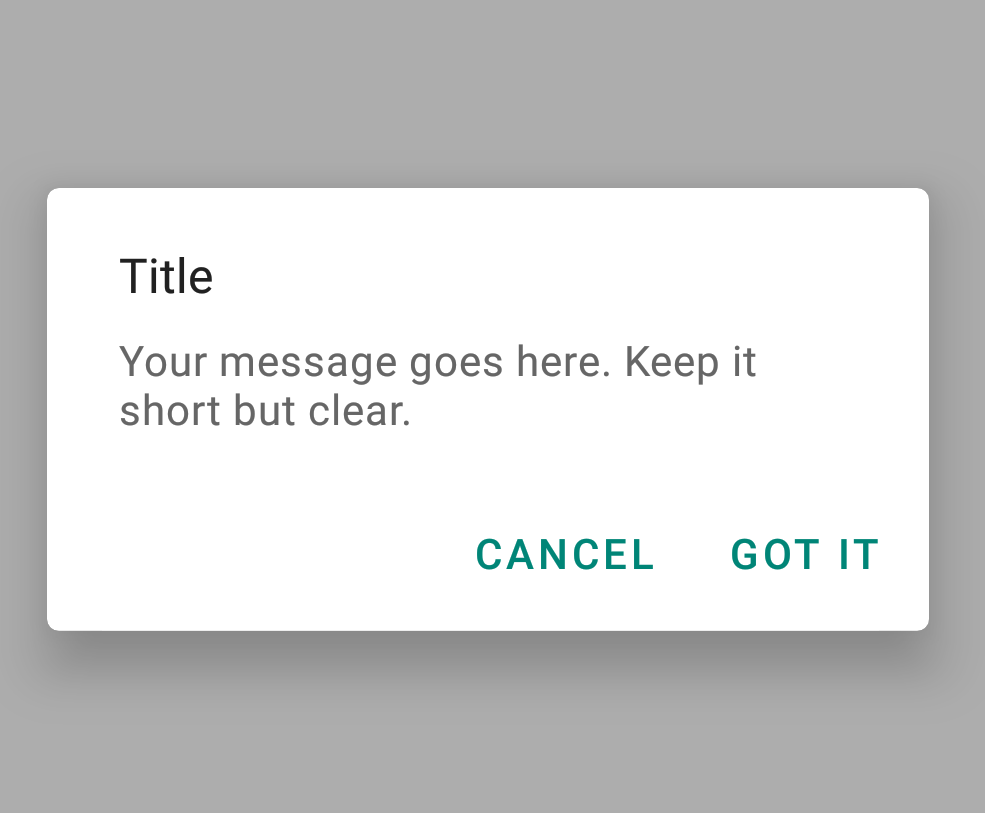
让我们与旧的警告对话框进行比较。
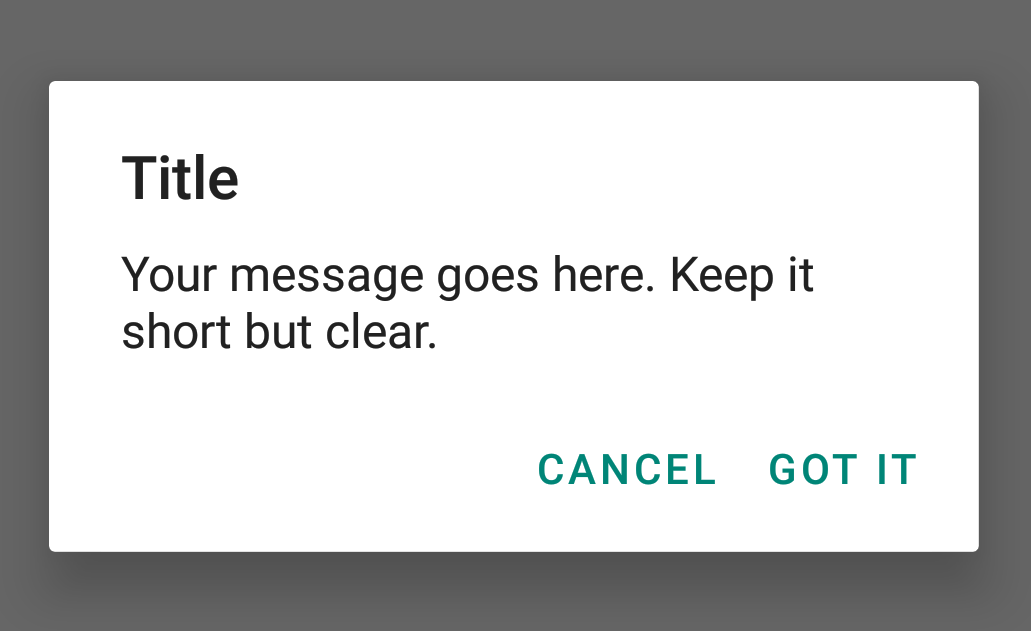
毫不疑问,新的MaterialAlertDialog看起来更好。
AlertDialog在配置更改时会丢失其内容。因此建议使用AppCompatDialogFragment。但为了简化本教程,我们将继续使用MaterialAlertDialog。
按钮样式
由于MaterialAlertDialog中的按钮只是MaterialButtons,因此我们可以对其进行样式设置。可以设置轮廓按钮/无边框按钮、水波纹效果等。让我们来看一个例子:
<style name="AlertDialogTheme">
<item name="buttonBarPositiveButtonStyle">@style/Alert.Button.Positive</item>
<item name="buttonBarNeutralButtonStyle">@style/Alert.Button.Neutral</item>
</style>
<style name="Alert.Button.Positive" parent="Widget.MaterialComponents.Button.TextButton">
<item name="backgroundTint">@color/colorPrimaryDark</item>
<item name="rippleColor">@color/colorAccent</item>
<item name="android:textColor">@android:color/white</item>
<item name="android:textSize">14sp</item>
<item name="android:textAllCaps">false</item>
</style>
<style name="Alert.Button.Neutral" parent="Widget.MaterialComponents.Button.TextButton">
<item name="backgroundTint">@android:color/transparent</item>
<item name="rippleColor">@color/colorAccent</item>
<item name="android:textColor">@android:color/darker_gray</item>
<item name="android:textSize">14sp</item>
</style>
new MaterialAlertDialogBuilder(MainActivity.this, R.style.AlertDialogTheme)
.setTitle("Title")
.setMessage("Your message goes here. Keep it short but clear.")
.setPositiveButton("GOT IT", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
}
})
.setNeutralButton("LATER", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
}
})
.show();
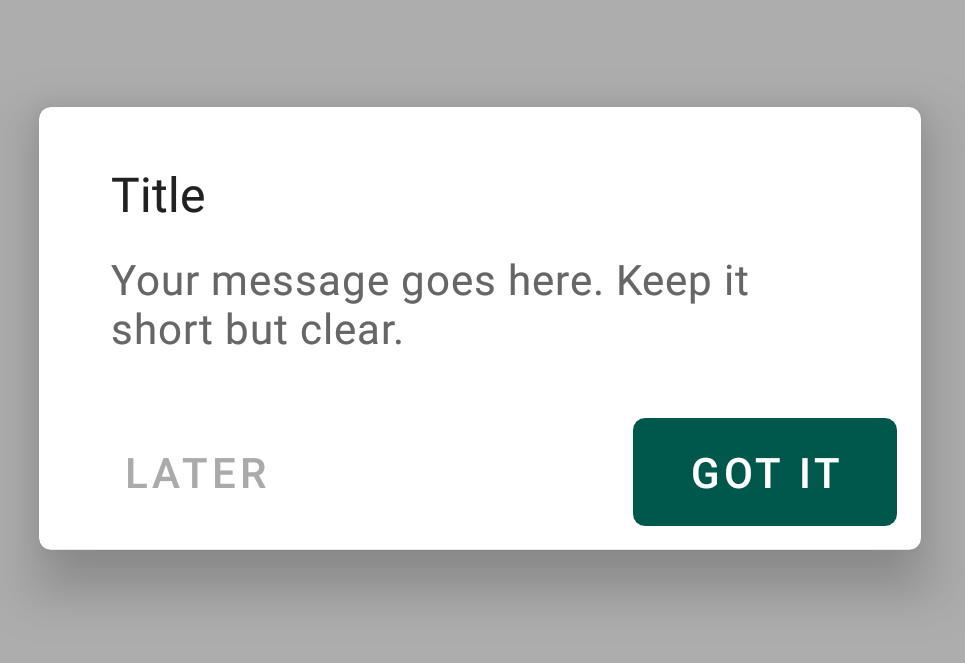
剪形对话
现在我们可以在Material对话框上设置形状了!让我们通过继承ShapeAppearance样式,将其中一个样式设置成剪裁形状。
<style name="CutShapeTheme" parent="ThemeOverlay.MaterialComponents.Dialog.Alert">
<item name="shapeAppearanceMediumComponent">@style/CutShapeAppearance</item>
</style>
<style name="CutShapeAppearance" parent="ShapeAppearance.MaterialComponents.MediumComponent">
<item name="cornerFamily">cut</item>
<item name="cornerSize">10dp</item>
</style>
现在只需在构建器构造函数中设置样式。
new MaterialAlertDialogBuilder(MainActivity.this, R.style.CutShapeTheme)
.setTitle("Title")
.setMessage("Your message goes here. Keep it short but clear.")
.setPositiveButton("GOT IT", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
}
})
.setNeutralButton("LATER", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
}
})
.show();
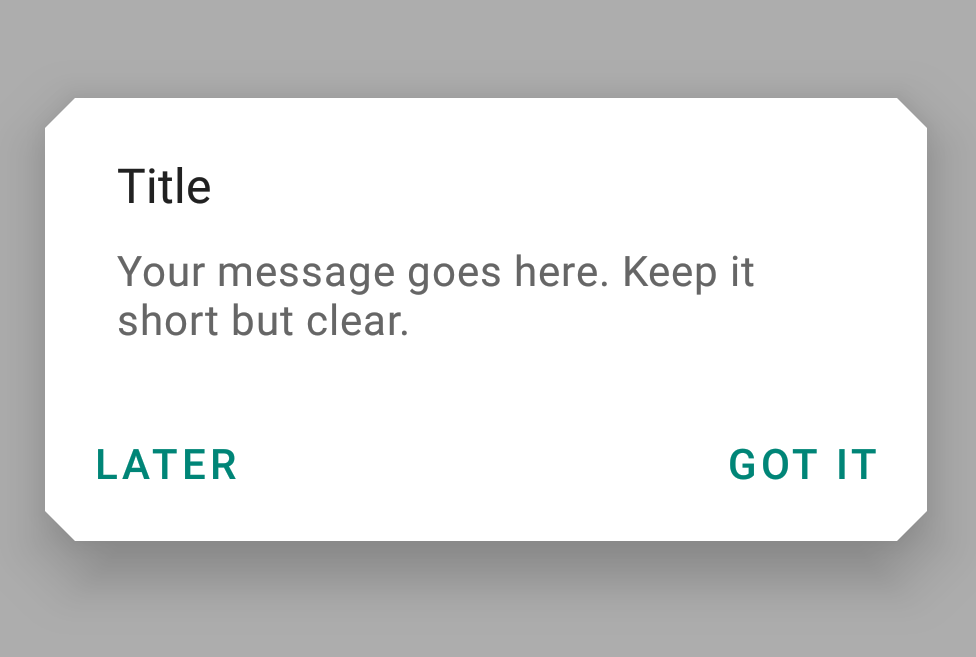
圆形的对话
<style name="RoundShapeTheme" parent="ThemeOverlay.MaterialComponents.Dialog.Alert">
<item name="shapeAppearanceMediumComponent">@style/RoundShapeAppearance</item>
</style>
<style name="RoundShapeAppearance" parent="ShapeAppearance.MaterialComponents.MediumComponent">
<item name="cornerFamily">rounded</item>
<item name="cornerSize">16dp</item>
</style>
将上面的样式设置在上一个代码片段的Dialog Builder构造函数中,我们得到了以下结果:
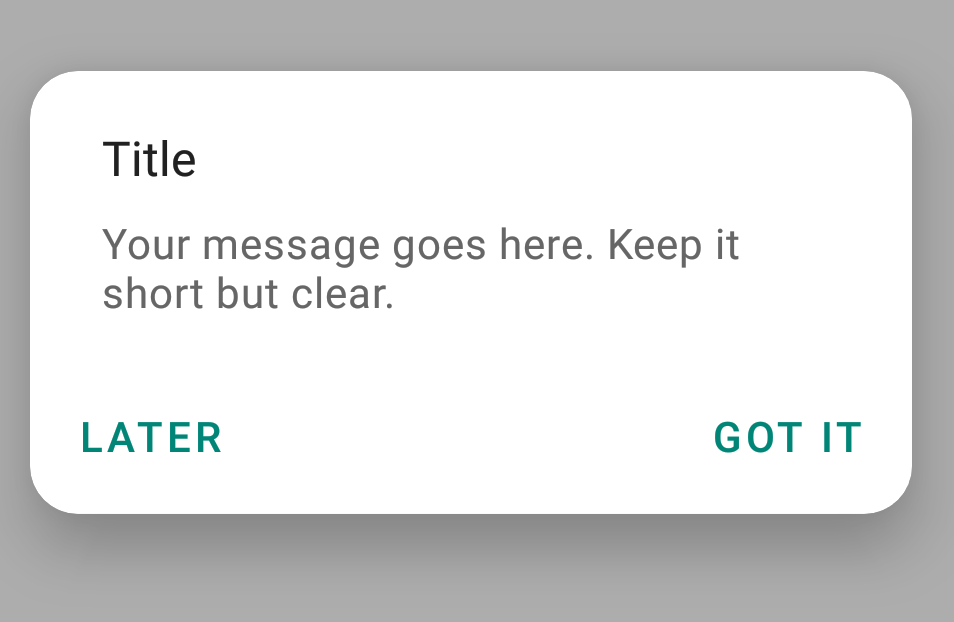
这些设计真的太让人流口水了!
自定义字体对话框
最后,我们可以像下面显示的那样为按钮和标题设置自定义字体系列。
<style name="CustomFont" parent="ThemeOverlay.MaterialComponents.Dialog.Alert">
<item name="fontFamily">@font/amatic</item>
<item name="android:textAllCaps">false</item>
</style>
这为我们呈现了一个全新的对话界面,如下所示:
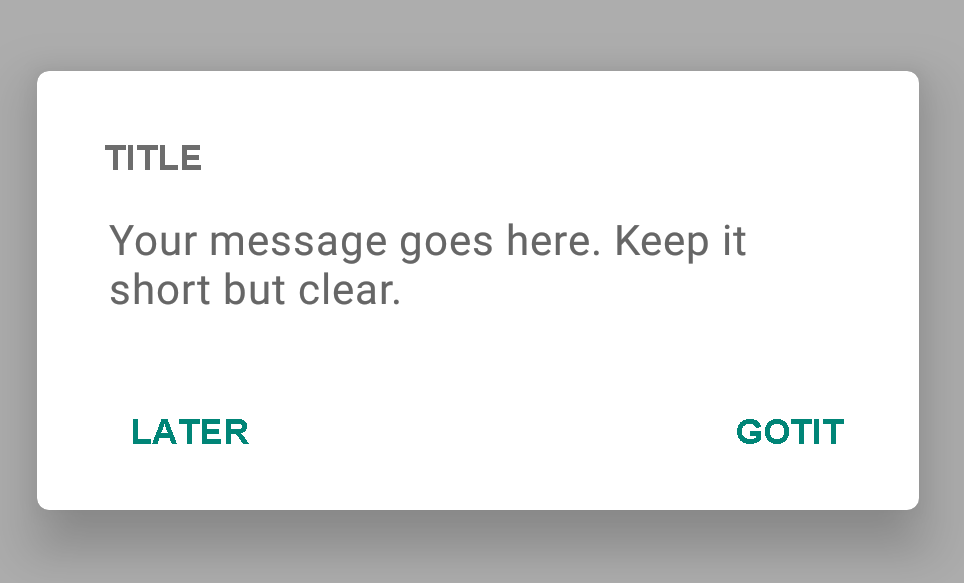