将Python中的Excel转换为JSON
有很多方法可以将Excel文件转换为JSON数据。在本教程中,我们将介绍两个Python模块来将Excel文件转换为JSON。
- Excel2JSON-3是Pandas的一个工具。
使用excel2json-3模块将Excel文件转换为JSON文件。
这是一个非常简单的模块,用于将Excel文件转换为JSON文件。Excel表格中的内容被转换为JSON字符串并保存在文件中。文件的名称是根据Excel表格的名称生成的。因此,如果有两个名称为“Numbers”和“Cars”的表格,那么生成的JSON文件将分别被命名为Numbers.json和Cars.json。该模块支持将.xls和.xlsx文件格式进行转换。我们可以从文件系统和URL中读取Excel文件。我们可以使用PIP命令安装该模块。
$ pip install excel2json-3
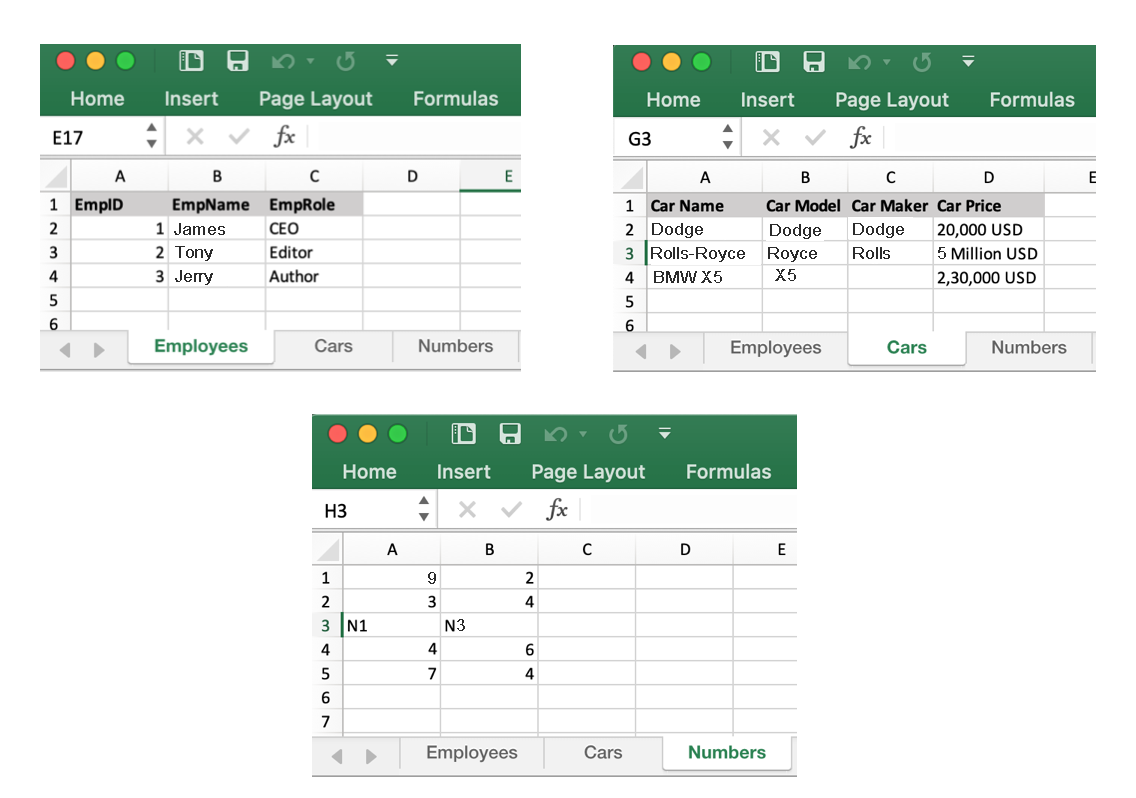
import excel2json
excel2json.convert_from_file('records.xlsx')
脚本会生成三个JSON文件,分别是Employees.json。
[
{
"EmpID": 1.0,
"EmpName": "Pankaj",
"EmpRole": "CEO"
},
{
"EmpID": 2.0,
"EmpName": "David Lee",
"EmpRole": "Editor"
},
{
"EmpID": 3.0,
"EmpName": "Lisa Ray",
"EmpRole": "Author"
}
]
Car.json
[
{
"Car Name": "Honda City",
"Car Model": "City",
"Car Maker": "Honda",
"Car Price": "20,000 USD"
},
{
"Car Name": "Bugatti Chiron",
"Car Model": "Chiron",
"Car Maker": "Bugatti",
"Car Price": "3 Million USD"
},
{
"Car Name": "Ferrari 458",
"Car Model": 458.0,
"Car Maker": "Ferrari",
"Car Price": "2,30,000 USD"
}
]
Number.json
[
{
"1.0": 3.0,
"2.0": 4.0
},
{
"1.0": "N1",
"2.0": "N2"
},
{
"1.0": 5.0,
"2.0": 6.0
},
{
"1.0": 7.0,
"2.0": 8.0
}
]
如果您需要从URL中读取Excel文件,请使用convert_from_url()函数。
excel2json-3 模块的限制
- The plugin has very limited features.
- There are no options to skip any sheet, rows, and columns. This makes it hard to use with bigger excel files.
- The JSON is saved into files. Most of the times, we want to convert to JSON and use it in our program rather than saving it as a file.
- The integers are getting converted to the floating point numbers.
使用Pandas模块将Excel表格转换为JSON字符串。
Pandas模块提供了将Excel表格读取为DataFrame对象的函数。有许多选项可以指定标题、读取特定列、跳过行等。您可以在Pandas read_excel() – Python中读取Excel文件中了解更多。我们可以使用to_json()函数将DataFrame对象转换为JSON字符串。让我们看一个简单的示例,读取“员工”表并将其转换为JSON字符串。
import pandas
excel_data_df = pandas.read_excel('records.xlsx', sheet_name='Employees')
json_str = excel_data_df.to_json()
print('Excel Sheet to JSON:\n', json_str)
输出:
Excel Sheet to JSON:
{"EmpID":{"0":1,"1":2,"2":3},"EmpName":{"0":"Pankaj","1":"David Lee","2":"Lisa Ray"},"EmpRole":{"0":"CEO","1":"Editor","2":"Author"}}
因此,JSON数据是按列生成的。如果您想按行生成JSON字符串,请将“orient”参数值设置为“records”。
json_str = excel_data_df.to_json(orient='records')
输出:
Excel Sheet to JSON:
[{"EmpID":1,"EmpName":"Pankaj","EmpRole":"CEO"},{"EmpID":2,"EmpName":"David Lee","EmpRole":"Editor"},{"EmpID":3,"EmpName":"Lisa Ray","EmpRole":"Author"}]
结论
如果您有一个简单且结构良好的Excel文件,并且希望将其转换为JSON文件,请使用excel2json-3模块。但是,如果您希望对Excel数据的读取和转换为JSON字符串有更多控制权,请使用pandas模块。
参考文献
- excel2json-3 PyPI.org Page
- Pandas DataFrame to_json() API Doc