在安卓中自定义进度条
在安卓应用中使用自定义进度条能增加一些个性化。在本教程中,我们将实现在应用中添加一个旋转的标志图标来创建一个自定义进度条。大部分情况下,在数据加载过程中,我们会使用ProgressBar作为加载图标。根据当前趋势,像Reddit、Uber、Foodpanda和Twitter这样的应用已经将普通的进度条替换为他们应用的图标作为加载图标。这为他们的应用和品牌图标增添了一些特色,使它们与众不同。
自定义Android中的进度条
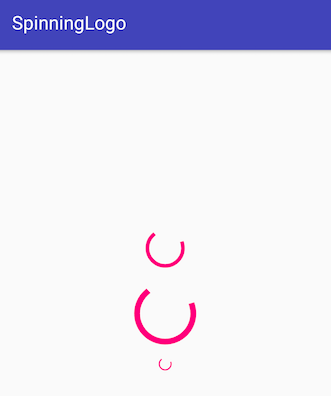
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:app="https://schemas.android.com/apk/res-auto"
xmlns:tools="https://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.Olivia.spinninglogo.MainActivity">
<ProgressBar
android:id="@+id/progressBarLarge"
style="?android:attr/progressBarStyleLarge"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ProgressBar
android:id="@+id/progressBarSmall"
style="?android:attr/progressBarStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@+id/progressBarLarge" />
<ProgressBar
android:id="@+id/progressBarMedium"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="8dp"
app:layout_constraintBottom_toTopOf="@+id/progressBarLarge"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
</android.support.constraint.ConstraintLayout>
我们在上面的布局中设置了三个无限旋转的圆形进度条。现在让我们尝试添加一个可以无限旋转图标的进度条。
自定义进度条的安卓工程结构
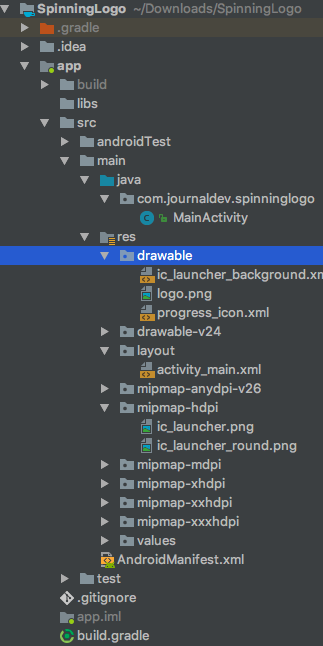
安卓自定义进度条代码
ProgressBar类包含一个indeterminateDrawable属性,该属性用指定的drawable替换默认的指示器。让我们看看在ProgressBar中放置一个图标时会发生什么。activity_main.xml的代码如下所示:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:app="https://schemas.android.com/apk/res-auto"
xmlns:tools="https://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.Olivia.spinninglogo.MainActivity">
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:indeterminateDrawable="@mipmap/ic_launcher"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
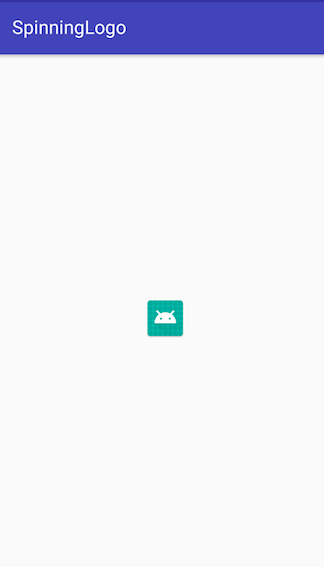
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="https://schemas.android.com/apk/res/android" >
<item>
<rotate
android:drawable="@mipmap/ic_launcher"
android:fillAfter="true"
android:fromDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:toDegrees="360" />
</item>
</layer-list>
属性android:fillAfter表示在动画结束后应用变换。 android:toDegrees的值可以增加或减少以改变旋转速度。通常建议将它设置为360的倍数。让我们在activity_main.xml中的ProgressBar中设置上述可绘制对象。
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:indeterminateDrawable="@drawable/progress_icon"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
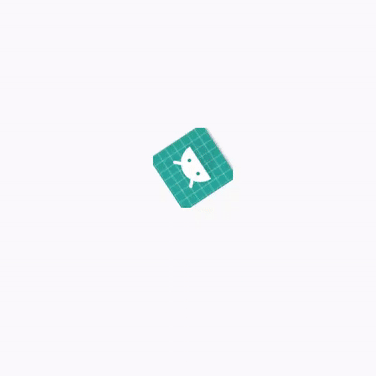
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="https://schemas.android.com/apk/res/android"
xmlns:app="https://schemas.android.com/apk/res-auto"
xmlns:tools="https://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.Olivia.spinninglogo.MainActivity">
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:indeterminateDrawable="@drawable/progress_icon"
android:visibility="gone"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="32dp"
android:text="TAP ME TO GET A RANDOM QUOTE"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="A Greeting Message Awaits You"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
请注意上述代码中对ConstraintLayout的高效使用。下面是MainActivity.java的代码。
package com.Olivia.spinninglogo;
import android.os.Handler;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
TextView textView;
List<String> quotesList;
ProgressBar progressBar;
int i = 0;
Handler handler = new Handler();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
quotesList = new ArrayList<>();
quotesList.add("Hi");
quotesList.add("Happy New Year");
quotesList.add("Hope you have a good day");
quotesList.add("Merry Christmas");
Button btnTap = findViewById(R.id.button);
textView = findViewById(R.id.textView);
progressBar = findViewById(R.id.progressBar);
btnTap.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
progressBar.setVisibility(View.VISIBLE);
textView.setVisibility(View.GONE);
handler.postDelayed(new Runnable() {
@Override
public void run() {
if (i == quotesList.size())
i = 0;
textView.setVisibility(View.VISIBLE);
textView.setText(quotesList.get(i++));
progressBar.setVisibility(View.GONE);
}
}, 3000);
}
});
}
}
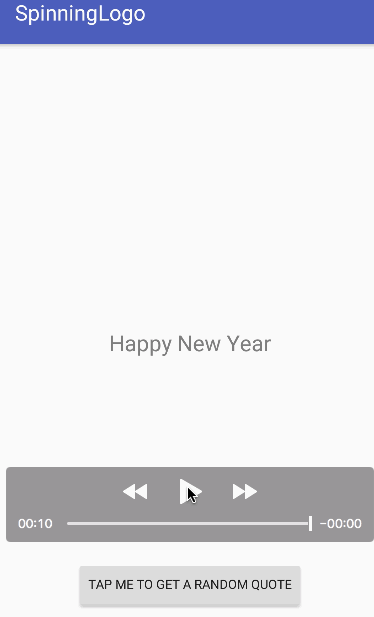
下载Android自定义进度条项目。
参考:API文档