Pythonのunittestでのユニットテストの例
今日は、Pythonのunittestについて学び、Pythonのユニットテストの実例プログラムを見ていきましょう。前のチュートリアルでは、Pythonのzip関数について学びました。
Pythonのunittest
Pythonのunittestモジュールはソースコードの単位をテストするために使用されます。例えば、プロジェクトをテストする必要があるとします。関数がどのようなデータを返すかはわかっています。大量のコードを書いた後、出力が正しいかどうかを確認する必要があります。通常、私たちがするのは出力を表示し、参照の出力ファイルと一致させるか、出力を手動で確認することです。この手間を省くために、Pythonではunittestモジュールが導入されています。このモジュールを使用すると、簡単なコードで関数の出力をチェックすることができます。このチュートリアルでは、Pythonのunittestモジュールの基本的な使い方とクラスの関数をテストするためのいくつかのPythonユニットテストケースについて説明します。
Pythonのユニットテストの例のソースコード。
まず最初に、それらをユニットテストするためのいくつかのコードを書く必要があります。Pythonのクラスを作成します。クラスの主な目的は、人物の名前を保存および取得することです。したがって、データを保存するためのset_name()関数と、クラスから名前を取得するためのget_name()関数を書きます。
class Person:
name = []
def set_name(self, user_name):
self.name.append(user_name)
return len(self.name) - 1
def get_name(self, user_id):
if user_id >= len(self.name):
return 'There is no such user'
else:
return self.name[user_id]
if __name__ == '__main__':
person = Person()
print('User Abbas has been added with id ', person.set_name('Abbas'))
print('User associated with id 0 is ', person.get_name(0))
私たちはクラスファイルをPerson.pyと名付けました。また、上記のコードの出力は以下のようになります。
$ python3.6 Person.py
User Abbas has been added with id 0
User associated with id 0 is Abbas
$
Pythonのunittestの構造
さあ、ユニットテストのためのコーディング方法を学びましょう。単体テストケースは、unittest.TestCaseをサブクラス化することで作成されます。適切な関数を上書きまたは追加することで、テストのためのロジックを追加することができます。次のコードは、aがbと等しい場合に成功します。
import unittest
class Testing(unittest.TestCase):
def test_string(self):
a = 'some'
b = 'some'
self.assertEqual(a, b)
def test_boolean(self):
a = True
b = True
self.assertEqual(a, b)
if __name__ == '__main__':
unittest.main()
Pythonのunittestモジュールの実行方法はどうすればいいですか。

Pythonのユニットテスト結果と基本的な機能
このユニットテストには3つの可能な結果があります。以下にそれらを示します。
-
- OK: もし全てのテストケースが合格した場合、出力はOKと表示します。
-
- Failure: もしテストケースのいずれかが失敗しAssertionError例外が発生した場合
- Error: もしAssertionError例外以外の例外が発生した場合。
unittestモジュールには、いくつかの関数があります。下にリストされています。
Method | Checks that |
---|---|
assertEqual(a,b) | a==b |
assertNotEqual(a,b) | a != b |
assertTrue(x) | bool(x) is True |
assertFalse(x) | bool(x) is False |
assertIs(a,b) | a is b |
assertIs(a,b) | a is b |
assertIsNot(a, b) | a is not b |
assertIsNone(x) | x is None |
assertIsNotNone(x) | x is not None |
assertIn(a, b) | a in b |
assertNotIn(a, b) | a not in b |
assertIsInstance(a, b) | isinstance(a, b) |
assertNotIsInstance(a, b) | not isinstance(a, b) |
Pythonのユニットテストの例を一つ挙げてください。
今、私たちのソースクラス「Person」のユニットテストを書く時間です。このクラスでは、「get_name()」と「set_name()」という2つの関数を実装しています。今回は、unittestを使ってこれらの関数をテストします。したがって、これらの2つの関数のために2つのテストケースを設計しました。以下のコードを見ていただければ、簡単に理解できると思います。
import unittest
# This is the class we want to test. So, we need to import it
import Person as PersonClass
class Test(unittest.TestCase):
"""
The basic class that inherits unittest.TestCase
"""
person = PersonClass.Person() # instantiate the Person Class
user_id = [] # variable that stores obtained user_id
user_name = [] # variable that stores person name
# test case function to check the Person.set_name function
def test_0_set_name(self):
print("Start set_name test\n")
"""
Any method which starts with ``test_`` will considered as a test case.
"""
for i in range(4):
# initialize a name
name = 'name' + str(i)
# store the name into the list variable
self.user_name.append(name)
# get the user id obtained from the function
user_id = self.person.set_name(name)
# check if the obtained user id is null or not
self.assertIsNotNone(user_id) # null user id will fail the test
# store the user id to the list
self.user_id.append(user_id)
print("user_id length = ", len(self.user_id))
print(self.user_id)
print("user_name length = ", len(self.user_name))
print(self.user_name)
print("\nFinish set_name test\n")
# test case function to check the Person.get_name function
def test_1_get_name(self):
print("\nStart get_name test\n")
"""
Any method that starts with ``test_`` will be considered as a test case.
"""
length = len(self.user_id) # total number of stored user information
print("user_id length = ", length)
print("user_name length = ", len(self.user_name))
for i in range(6):
# if i not exceed total length then verify the returned name
if i < length:
# if the two name not matches it will fail the test case
self.assertEqual(self.user_name[i], self.person.get_name(self.user_id[i]))
else:
print("Testing for get_name no user test")
# if length exceeds then check the 'no such user' type message
self.assertEqual('There is no such user', self.person.get_name(i))
print("\nFinish get_name test\n")
if __name__ == '__main__':
# begin the unittest.main()
unittest.main()
テストケース関数の実行順序は、その名前の順序に従っています。したがって、set_nameテストを最初に実行したい場合、テストケース関数をtest_0_set_nameとtest_1_get_nameという名前にしました。
Pythonのユニットテストの例の出力
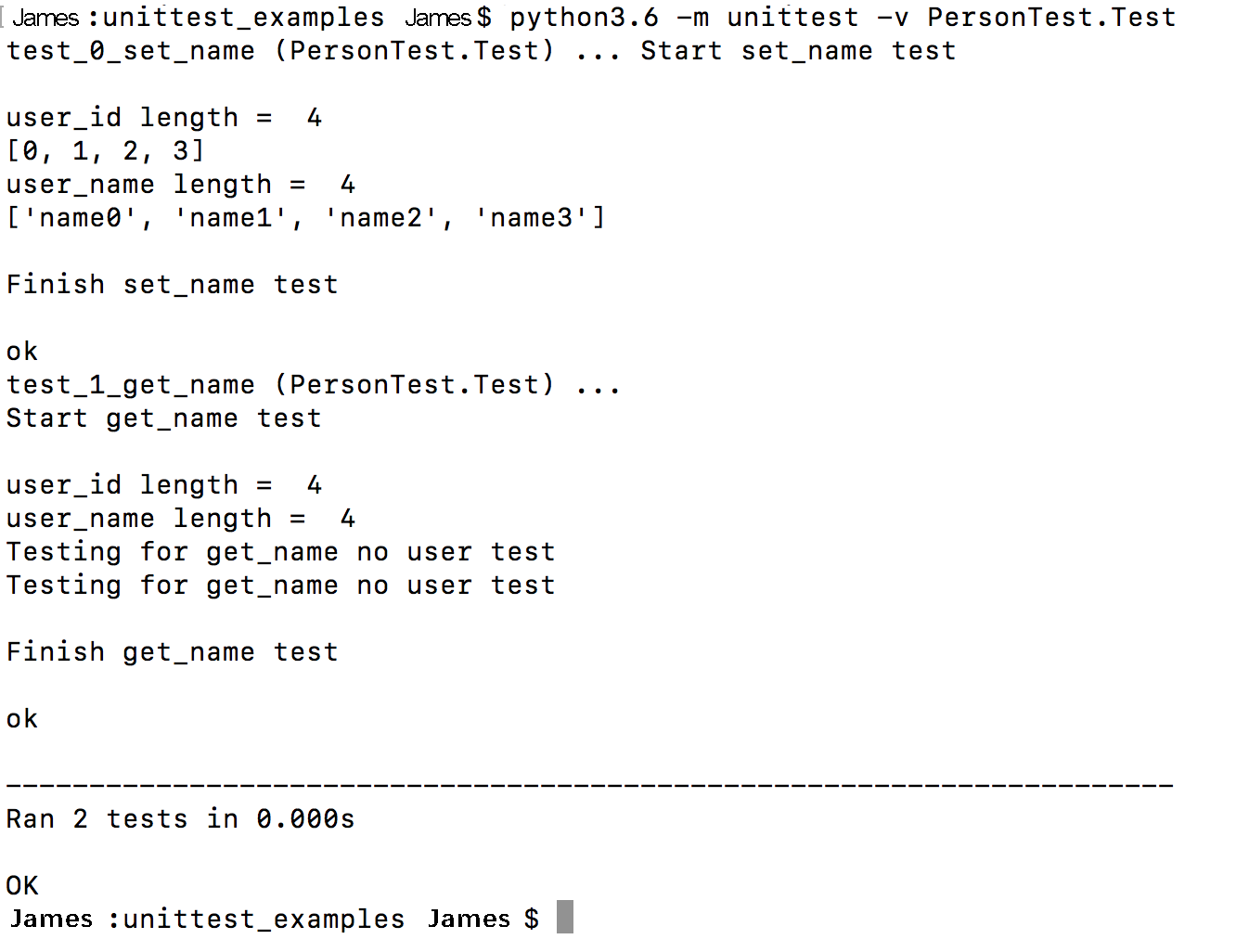
$ python3.6 -m unittest -v PersonTest.Test
test_0_set_name (PersonTest.Test) ... Start set_name test
user_id length = 4
[0, 1, 2, 3]
user_name length = 4
['name0', 'name1', 'name2', 'name3']
Finish set_name test
ok
test_1_get_name (PersonTest.Test) ...
Start get_name test
user_id length = 4
user_name length = 4
Testing for get_name no user test
Testing for get_name no user test
Finish get_name test
ok
----------------------------------------------------------------------
Ran 2 tests in 0.000s
OK
$
これはPythonのunittestチュートリアルについてのすべてです。もっと学びたい場合は、公式ドキュメントを読んでください。さらなる質問にはコメントボックスをご利用ください。 🙂