NumPy の行列の乗算
NumPyの行列の乗算は、以下の3つの方法で行うことができます。
-
- multiply():要素ごとの行列の乗算。
-
- matmul():2つの配列の行列積。
- dot():2つの配列の内積。
1. NumPyの行列の要素ごとの積
要素ごとの行列の掛け算が必要な場合は、multiply() 関数を使うことができます。
import numpy as np
arr1 = np.array([[1, 2],
[3, 4]])
arr2 = np.array([[5, 6],
[7, 8]])
arr_result = np.multiply(arr1, arr2)
print(arr_result)
出力:
[[ 5 12]
[21 32]]
下の画像は、結果行列を得るために実行された乗算操作を示しています。
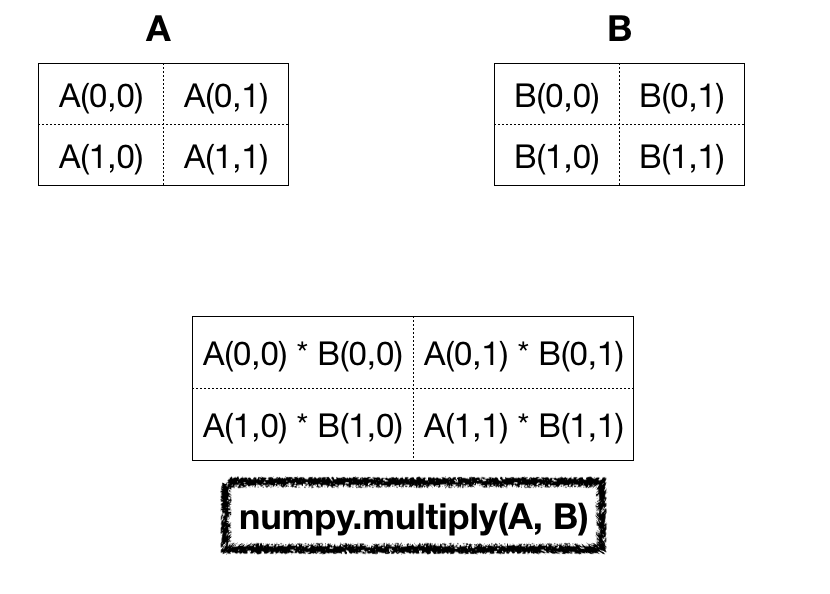
2. 二つのNumPy配列の行列積
もし2つの配列の行列積を取得したい場合は、matmul()関数を使用してください。
import numpy as np
arr1 = np.array([[1, 2],
[3, 4]])
arr2 = np.array([[5, 6],
[7, 8]])
arr_result = np.matmul(arr1, arr2)
print(f'Matrix Product of arr1 and arr2 is:\n{arr_result}')
arr_result = np.matmul(arr2, arr1)
print(f'Matrix Product of arr2 and arr1 is:\n{arr_result}')
以下を日本語で言い換えてください。
「Output:」
Matrix Product of arr1 and arr2 is:
[[19 22]
[43 50]]
Matrix Product of arr2 and arr1 is:
[[23 34]
[31 46]]
下の図は、結果配列の各インデックスに対する行列積演算を説明しています。簡単のため、各インデックスについて、最初の配列から行を取り、2番目の配列から列を取ります。そして、対応する要素を乗算し、それらを足し合わせて行列積の値を得ます。
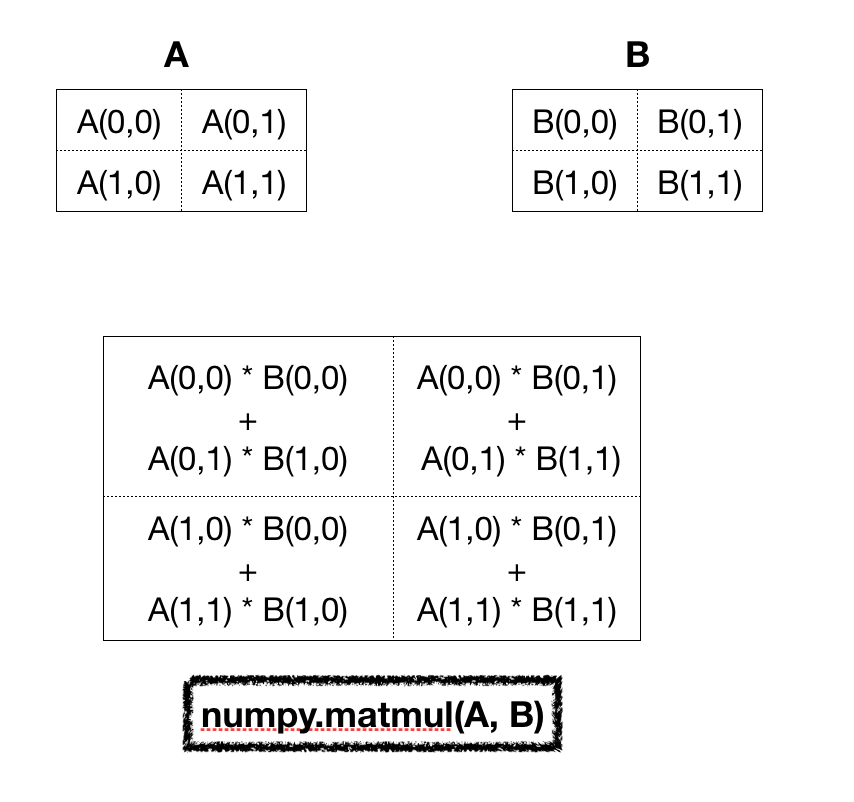
2つの配列の行列積は、引数の位置に依存します。そのため、matmul(A, B)とmatmul(B, A)は異なる場合があります。
3. 2つのNumPy配列のドット積
NumPyのdot()関数は、2つの配列の内積を返します。結果は、1次元および2次元の配列に対するmatmul()関数と同じです。
import numpy as np
arr1 = np.array([[1, 2],
[3, 4]])
arr2 = np.array([[5, 6],
[7, 8]])
arr_result = np.dot(arr1, arr2)
print(f'Dot Product of arr1 and arr2 is:\n{arr_result}')
arr_result = np.dot(arr2, arr1)
print(f'Dot Product of arr2 and arr1 is:\n{arr_result}')
arr_result = np.dot([1, 2], [5, 6])
print(f'Dot Product of two 1-D arrays is:\n{arr_result}')
出力:
Dot Product of arr1 and arr2 is:
[[19 22]
[43 50]]
Dot Product of arr2 and arr1 is:
[[23 34]
[31 46]]
Dot Product of two 1-D arrays is:
17
おすすめの読み物:
- numpy.square()
- NumPy sqrt() – Square Root of Matrix Elements
- Python NumPy Tutorial
引用文献
- numpy matmul()
- numpy multiply()