Kotlinを使用したAndroidのスピナー
このチュートリアルでは、Kotlinを使用してAndroidアプリケーションでスピナーを説明し、実装します。Androidのスピナーは、画面上にドロップダウンリストを作成するために使用されます。
何を学びますか?
- Creating Spinners through XML and Programmatically
- Setting a prompt on the Spinner.
- Creating a custom layout for the Spinner.
- Handling Click Listeners and Display a Toast message.
- Preventing the Click Listener from being fired automatically for the first time.
アンドロイドのスピナーは何ですか? (Andoroido no Supinā wa nan desu ka?)
スピナーは、選択できる項目のリストを含むドロップダウンメニューのようなものです。値が選択されると、スピナーは選択された値を持ったデフォルトの状態に戻ります。Android 3.0以降、スピナーのデフォルト状態としてプロンプトを表示することはできません。代わりに、最初の項目が表示されます。スピナー内のデータはアダプターを使用してロードされます。以下のシナリオを考えてみてください:電話を充電する必要があるとします。そのためには、アダプターを使って電話の充電器を電気ボードに接続する必要があります。そしてアダプターは電話に電気を供給します。Androidでは、スピナーはアダプターを利用してデータが読み込まれる電話のようなものです。アダプターはスピナーに読み込む項目のデータだけでなく、レイアウトも設定します。
スピナーコールバックイベント
Spinnerのクリックイベントコールバックをトリガーするために、AdapterView.onItemSelectedListener インターフェースが使用されます。このインターフェースには、2つのメソッドが含まれています。
- onItemSelected
- onNothingSelected
次のセクションでは、新しいAndroid Studioプロジェクトを作成し、アプリケーションでスピナーを実装します。レイアウトをカスタマイズして、さまざまなシナリオに対処する方法を学びます。
アンドロイド スピナー コトリン プロジェクト
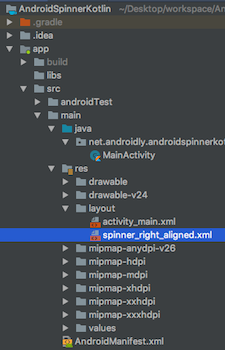
1. XMLレイアウトのコード
以下にactivity_main.xml レイアウトファイルのコードが記載されています。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@+id/linearLayout"
android:gravity="center"
tools:context=".MainActivity">
<Spinner
android:id="@+id/mySpinner"
android:layout_width="match_parent"
android:spinnerMode="dialog"
android:layout_height="wrap_content" />
</LinearLayout>
現在、Spinnerは1つのみホストしており、android:spinnerModeはダイアログまたはドロップダウンのどちらかになります。
プロンプトを表示するためには、spinnerModeの値としてdialogを使用する必要があります。
2. スピナーのXMLコード
以下にspinner_right_aligned.xmlのコードが示されています。
<?xml version="1.0" encoding="utf-8"?>
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="end"
android:padding="15dp"
android:textAlignment="gravity"
android:textColor="@color/colorPrimary"
android:textSize="16sp"
/>
3. メインアクティビティのKotlinコード
以下にMainActivity.ktクラスのコードが示されています。
package net.androidly.androidspinnerkotlin
import android.content.Context
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.view.Gravity
import android.view.View
import android.widget.*
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity(), AdapterView.OnItemSelectedListener {
var languages = arrayOf("Java", "PHP", "Kotlin", "Javascript", "Python", "Swift")
val NEW_SPINNER_ID = 1
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
var aa = ArrayAdapter(this, android.R.layout.simple_spinner_item, languages)
aa.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item)
with(mySpinner)
{
adapter = aa
setSelection(0, false)
onItemSelectedListener = this@MainActivity
prompt = "Select your favourite language"
gravity = Gravity.CENTER
}
val spinner = Spinner(this)
spinner.id = NEW_SPINNER_ID
val ll = LinearLayout.LayoutParams(LinearLayout.LayoutParams.MATCH_PARENT, LinearLayout.LayoutParams.WRAP_CONTENT)
ll.setMargins(10, 40, 10, 10)
linearLayout.addView(spinner)
aa = ArrayAdapter(this, R.layout.spinner_right_aligned, languages)
aa.setDropDownViewResource(R.layout.spinner_right_aligned)
with(spinner)
{
adapter = aa
setSelection(0, false)
onItemSelectedListener = this@MainActivity
layoutParams = ll
prompt = "Select your favourite language"
setPopupBackgroundResource(R.color.material_grey_600)
}
}
override fun onNothingSelected(parent: AdapterView<*>?) {
showToast(message = "Nothing selected")
}
override fun onItemSelected(parent: AdapterView<*>?, view: View?, position: Int, id: Long) {
when (view?.id) {
1 -> showToast(message = "Spinner 2 Position:${position} and language: ${languages[position]}")
else -> {
showToast(message = "Spinner 1 Position:${position} and language: ${languages[position]}")
}
}
}
private fun showToast(context: Context = applicationContext, message: String, duration: Int = Toast.LENGTH_LONG) {
Toast.makeText(context, message, duration).show()
}
}
重要なポイント:
1. Please submit your report by the deadline.
締め切りまでにレポートを提出してください。
2. It is crucial to prioritize customer satisfaction.
顧客満足を優先することが重要です。
3. We need to address this issue promptly.
この問題には迅速に対処する必要があります。
4. Teamwork plays a vital role in achieving success.
チームワークは成功を達成するために重要な役割を果たします。
5. Please bring any concerns or issues to our attention.
何か懸念事項や問題があれば、お知らせください。
6. It is essential to follow company policies and procedures.
会社の規則や手順に従うことが重要です。
7. Continuous improvement is key to staying competitive in the market.
競争力を維持するためには、継続的な改善が重要です。
8. Your input and feedback are highly valued.
貴重なご意見やフィードバックを大切にしています。
9. Effective communication is essential for a productive work environment.
生産的な職場環境を作るためには、効果的なコミュニケーションが重要です。
10. Investing in employee training and development leads to long-term success.
従業員の研修と成長に投資することが長期的な成功につながります。
- Thanks to Kotlin Android extensions, the XML Spinner widget is automatically available in our Kotlin Activity class.
- We’ve created an arrayOf strings that consist of programming languages. These are filled in the adapter using the ArrayAdapter.
- The setDropDownViewResource is used to set the layout for the selected state and the spinner list rows.
- The android.R.layout.simple_spinner_item is used to set the default android SDK layout. By default, the TextView is left aligned in this type of layout.
私たちは、spinner_right_aligned.xml ファイルからレイアウトを読み込むため、プログラムで2番目のスピナーを作成しました。
Activityが作成されたときに、SpinnerのOnItemSelectedメソッドが発動するのを防ぐために、setSelection(0、false)が使用されます。
「それはどのように機能しますか? setSelection()メソッドは、最初のスピナーアイテムがすでに選択されたことをアクティビティに伝えます。このステートメントは、onItemSelectedListener = thisの前に配置する必要があります。setPopupBackgroundResourceは、ドロップダウンリストの背景色を設定するために使用されます。onItemSelected関数の中で、respective Spinnerアイテムに対してToastをトリガーするためにwhenステートメントを使用します。Kotlinとデフォルト値を持つ関数のおかげで、Toastの冗長な呼び出しを削減しています。」
4. スピナーコトリンアプリの出力
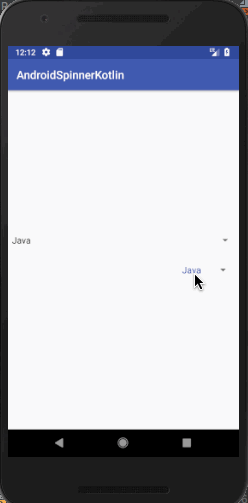