C++において文字列を逆さまにする
様々な状況で、C++プログラミングにおいて文字列を反転させる必要があることがあります。それは、反転した文字列を単に出力するだけの場合もありますし、場合によっては文字列を永続的にアドレスで反転させる必要があるかもしれません。
このチュートリアルでは、異なる予め定義された関数やユーザー定義の関数を使って、両方のタスクをどのように達成するかを学びます。
C++で文字列を逆さまにする。
文字列の逆転とは、その文字列の文字の順序を逆にする操作を指します。例えば、文字列「JournalDev」が「str」に含まれている場合、これを逆転させるということです。
文字列「str」に逆転操作が実行された後、内容は逆になります。したがって、今、「str」には文字列「veDlanruoJ」が含まれるべきです。
今度は、さまざまなテクニックを使ってC++の文字列に逆操作を行う方法を見てみましょう。
C++でreverse()関数を使う
ビルトインのリバース関数reverse()はC++で、文字列を直接逆さにします。双方向の開始イテレータと終了イテレータの両方が引数として渡されます。
この機能はアルゴリズムヘッダーファイルで定義されています。以下のコードはreverse()関数の使用方法を説明しています。
#include <algorithm>
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str = "Journal Dev reverse example";
reverse(str.begin(), str.end());
cout<<"\n"<<str;
return 0;
}
出力:最適な解だけを日本語で言い換えてください。
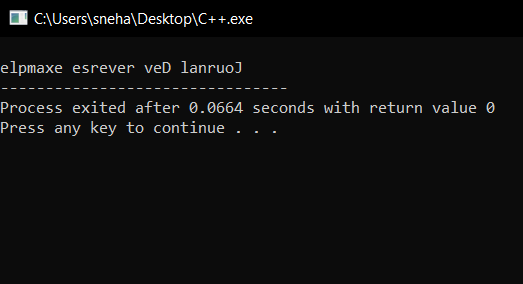
strrev()を使用して
strrev()は、cstring.hのヘッダーファイル内に定義された、C++の事前定義関数です。これは、任意のC文字列(文字配列)を逆順にするのに広く使用できます。
さらに、strrev()関数は引数として文字列のベースアドレスのみを必要とし、文字列を逆にします。C++でstrrev()関数を使用して文字列を逆にする方法を見てみましょう。
#include<iostream>
#include<cstring>
using namespace std;
int main()
{
char str[] ="Journal Dev reverse example";
strrev(str);
cout<<"\n"<<str;
return 0;
}
出力:
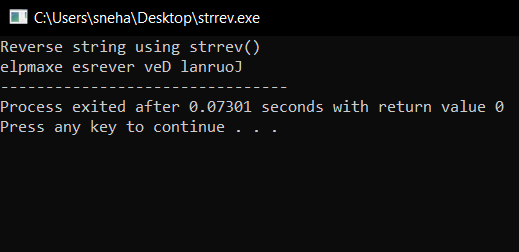
上記のコードは、関数strrev()の動作を非常によく示しています。文字列「str」に対して、関数は出力結果自体で見ることができるように、それを成功裏に逆さにします。
文字列を逆に表示する
特定の場合において、文字列を変更する必要はなく、逆順で印刷するだけで十分な場合があります。これは変更できない定数文字列の場合に起こります。ループを使用することで、任意の文字列を逆順に印刷することができます。では、具体的な方法を見てみましょう。
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str="Journal Dev reverse example";
int i;
cout<<"Printing string in reverse\n";
for(i = str.length() - 1; i >= 0; i--)
{
cout<<str[i];
}
return 0;
}
出力:
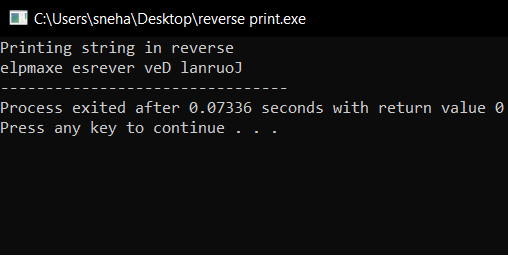
- In the above-given code, we have firstly initialized a string ‘str’.
- Inside the for loop, while printing the string, note that we have initialized the iterator ‘i’ with a value str.length()-1. This means that we print the string character by character but, starting from the last index.
- Note: length() returns the length of a string. So, for printing a string in reverse we should consider the last index which should be length()-1, since indexing starts from ‘0’ in a character array.
自分自身で文字列を逆さにする関数、My_rev() を作成する。
これまで、私たちはさまざまな事前定義された関数を使用して文字列を逆に出力したり、文字列自体を逆にする方法を学びました。次に、与えられた文字列を逆にするための独自の関数である” My_rev()”を作成または定義しましょう。
#include<iostream>
#include<string>
#include<cstring>
using namespace std;
char *My_rev(char *str)
{
int i,len=0,n;
char temp;
len=strlen(str);
n=len-1;
for(i = 0; i <=(len/2); i++)
{
temp=str[i];
str[i]=str[n];
str[n]=temp;
n--;
}
return str;
}
int main()
{
char My_string[]="Journal Dev reverse example";
cout<<"Reverse string using My_rev()...\n";
My_rev(My_string);
cout<<My_string;
return 0;
}
出力:
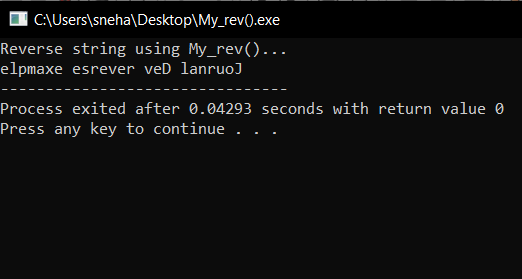
- In the code above, My_rev() is a function that reverses a string, given the base address of the string is passed as an argument.
- Inside the My_rev() function, *str is a pointer that stores the base address of the provided string. In our case, str points to the first element of the string My_string.
- len stores the length of the string. Whereas, n is the index of the last element.
- In the function, we try to swap individual characters of the string, from both ends. That means we go on swapping elements from the 0th and nth index until we get to the (len/2)th position. In the code above, the for loop does this swapping for us which technically reverses the string.
- In the end, we return the base address str to the main() function. Where the string is printed using the cout function.
参考文献
- https://stackoverflow.com/questions/198199/how-do-you-reverse-a-string-in-place-in-c-or-c
- /community/tutorials/string-length-in-c-plus-plus