Sort the Java Collections using the sort() method.
We will explore the Java Collections sort method today. In Java, when dealing with Collections, it is quite common to require data sorting.
Sort the Java collections using the sort() method.
The Java Collections class offers the Collections.sort() method, making it easy to sort all List implementations such as LinkedList and ArrayList. This method has two overloaded versions available.
-
- sort(List list): Orders the elements of the List in an ascending manner based on their inherent ordering.
- sort(List list, Comparator c): Orders the elements of the list according to the sequence determined by the comparator.
Please be aware that the previously mentioned methods have generics in their signature, but I have excluded them here for the sake of clarity. Let’s now explore separately how and under what circumstances we can utilize these two methods.
Sort the list using Java Collections.
Think about an ArrayList that contains strings.
List<String> fruits = new ArrayList<String>();
fruits.add("Apple");
fruits.add("Orange");
fruits.add("Banana");
fruits.add("Grape");
Next, we will arrange it using Collections.sort().
Collections.sort(fruits);
// Print the sorted list
System.out.println(fruits);
The program will produce the following result.
[Apple, Banana, Grape, Orange]
Therefore, it is evident that the list of Strings has been sorted in lexical order by Collections.sort(). This method does not produce any output. Now, let’s contemplate a scenario where we have a list of custom objects such as Fruit.
package com.scdev.collections;
public class Fruit{
private int id;
private String name;
private String taste;
Fruit(int id, String name, String taste){
this.id=id;
this.name=name;
this.taste=taste;
}
}
Shall we compile a roster of different types of fruits?
List<Fruit> fruitList=new ArrayList<Fruit>();
Fruit apple=new Fruit(1, "Apple", "Sweet");
Fruit orange=new Fruit(2, "Orange", "Sour");
Fruit banana=new Fruit(4, "Banana", "Sweet");
Fruit grape=new Fruit(3, "Grape", "Sweet and Sour");
fruitList.add(apple);
fruitList.add(orange);
fruitList.add(banana);
fruitList.add(grape);
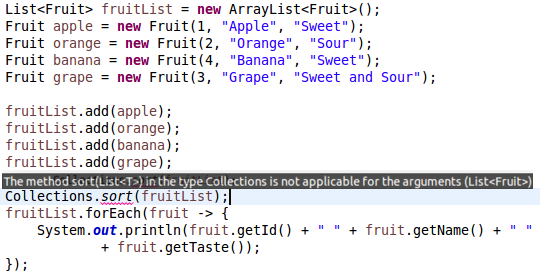
package com.scdev.collections;
public class Fruit implements Comparable<Object>{
private int id;
private String name;
private String taste;
Fruit(int id, String name, String taste){
this.id=id;
this.name=name;
this.taste=taste;
}
@Override
public int compareTo(Object o) {
Fruit f = (Fruit) o;
return this.id - f.id ;
}
}
Since Comparable has been implemented, the list can now be sorted without encountering any errors.
Collections.sort(fruitList);
fruitList.forEach(fruit -> {
System.out.println(fruit.getId() + " " + fruit.getName() + " " +
fruit.getTaste());
});
The result will appear in the following manner:
1 Apple Sweet
2 Orange Sour
3 Grape Sweet and Sour
4 Banana Sweet
Sort a List in Java using the Collections class and a custom Comparator.
To establish a customized sorting method that deviates from the default ordering of the elements, we can employ the java.util.Comparator interface and submit an instance of it as the second parameter of the sort() function. Suppose we want to organize the elements based on the “name” attribute of the Fruit. We implement the Comparator and within its compare() function, we define the comparison algorithm.
package com.scdev.collections;
class SortByName implements Comparator<Fruit> {
@Override
public int compare(Fruit a, Fruit b) {
return a.getName().compareTo(b.getName());
}
}
We can now arrange it by employing this comparator.
Collections.sort(fruitList, new SortByName());
Here is how the output will appear:
1 Apple Sweet
4 Banana Sweet
3 Grape Sweet and Sour
2 Orange Sour
We can provide sorting logic at runtime using lambda functions, eliminating the need to write a new class for the Comparator.
Collections.sort(fruitList, (a, b) -> {
return a.getName().compareTo(b.getName());
});
Reverse order is a feature in Java Collections.
By default, when using Collection.sort, the elements are sorted in ascending order. However, if we want to sort the elements in the opposite direction, there are several methods we can utilize.
-
- reverseOrder(): This method returns a Comparator that applies the opposite ordering of the elements’ natural order in the collection.
- reverseOrder(Comparator cmp): This method returns a Comparator that applies the reverse ordering based on the provided comparator.
Here are the samples for both of these approaches:
Example of using the reverseOrder() method in Java Collections.
Collections.sort(fruits, Collections.reverseOrder());
System.out.println(fruits);
The fruits will be displayed in reverse alphabetical order.
[Orange, Grape, Banana, Apple]
One possible paraphrase could be: “An example of using the Java Collections reverseOrder method with a specified Comparator.”
Collections.sort(fruitList, Collections.reverseOrder(new SortByName()));
fruitList.forEach(fruit -> {
System.out.println(fruit.getId() + " " + fruit.getName() + " " +
fruit.getTaste());
});
Can you provide only one option for paraphrasing the following sentence natively?
“Output:”
2 Orange Sour
3 Grape Sweet and Sour
4 Banana Sweet
1 Apple Sweet
There are no more details to cover regarding the sort() method in Java Collections, along with its examples. Reference: API Documentation.
more tutorials
Convert string to XML document in Java(Opens in a new browser tab)
How to include items to a list in Python(Opens in a new browser tab)
Java String substring() method(Opens in a new browser tab)
Adding a string to a Python variable(Opens in a new browser tab)
EasyMock void method to have its last call.(Opens in a new browser tab)