EasyMock void method to have its last call.
Mocking void methods using the EasyMock expect() method is not possible. However, we can instead use expectLastCall() in conjunction with andAnswer() to successfully mock void methods.
Create a void method in EasyMock.
If we utilize expectLastCall() along with andAnswer() for mocking void methods, we can utilize getCurrentArguments() to retrieve the arguments passed to the method and carry out certain actions on it. Ultimately, we need to return null since we are simulating a void method. Suppose we possess a utility class like the following:
package com.scdev.utils;
public class StringUtils {
public void print(String s) {
System.out.println(s);
}
}
EasyMock provides the code to mimic the print() function of a void method.
package com.scdev.easymock;
import static org.easymock.EasyMock.*;
import org.junit.jupiter.api.Test;
import com.scdev.utils.StringUtils;
public class EasyMockVoidMethodExample {
@Test
public void test() {
StringUtils mock = mock(StringUtils.class);
mock.print(anyString());
expectLastCall().andAnswer(() -> {
System.out.println("Mock Argument = "
+getCurrentArguments()[0]);
return null;
}).times(2);
replay(mock);
mock.print("Java");
mock.print("Python");
verify(mock);
}
}
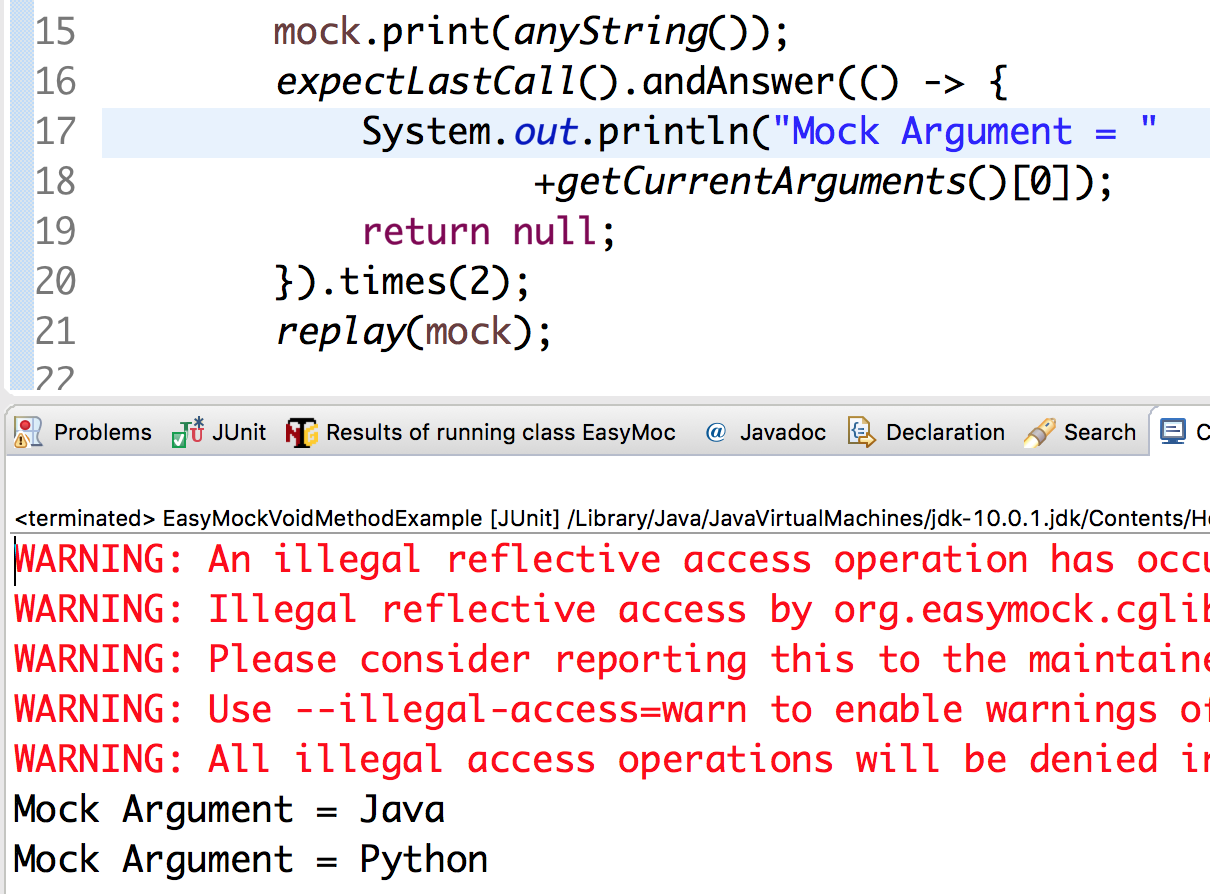
We anticipate the last call to be void.
For mocking a void method without executing any logic, we can utilize expectLastCall().andVoid() immediately after invoking the void method on the mocked object.
Our GitHub Repository contains a comprehensive project and additional EasyMock examples that you can explore and access.
more tutorials
Java String substring() method(Opens in a new browser tab)
Spring MVC HandlerInterceptorAdapter and HandlerInterceptor.(Opens in a new browser tab)
BroadcastReceiver Example Tutorial on Android(Opens in a new browser tab)