Set in Python
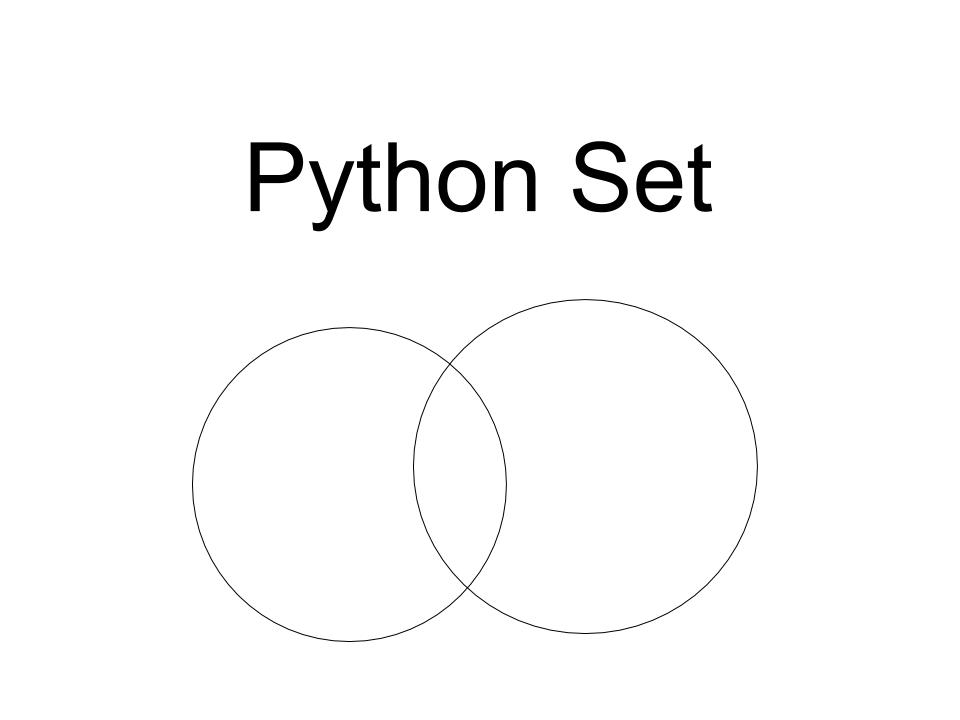
Set in Python
A Python Set is a collection of distinct elements that is not ordered. If you have a list and you only want the unique items from it, you can utilize a Python Set. Likewise, if you require only unique items from an input, a Python set can assist in accomplishing that. It is possible to add or remove items from the set. To initialize a set, you can enclose the elements within curly braces. Similar to other sequences, a set can contain elements of different data types. Additionally, you can convert a list into a set by using the set() function. The following example provides an illustration of how to initialize a set.
#set containing single data-type
set1 = {1, 2, 3, 4, 2, 3, 1}
print(set1)
#set containing multiple data-type
set2 = {1, 2, 3, (1, 2, 3), 2.45, "Python", 2, 3}
print(set2)
#creating a set from a list
theList = [1, 2, 3, 4, 2, 3, 1]
theSet = set(theList)
print(theSet)
The result will be.
================== RESTART: /home/imtiaz/set1.py ==================
set([1, 2, 3, 4])
set([1, 2, 3, 2.45, 'Python', (1, 2, 3)])
set([1, 2, 3, 4])
>>>
Including items in a Python set
In the previous example, we learned the direct initialization of a Python set. If we want to add an element to the set, we can achieve this by using the add() function. However, this function can only add one element at a time. If we want to add iterable elements such as a list or set, we can use the update() function instead. The following example will provide a better understanding of this concept.
#initialize an empty set
theSet = set()
#add a single element using add() function
theSet.add(1)
theSet.add(2)
theSet.add(3)
theSet.add(2)
#add another data-type
theSet.add('hello')
#add iterable elements using update() function
theSet.update([1,2,4,'hello','world']) #list as iterable element
theSet.update({1,2,5}) #set as iterable element
print(theSet)
The result of the given code will be.
================== RESTART: /home/imtiaz/set_new.py ==================
set([1, 2, 3, 4, 5, 'world', 'hello'])
>>>
Take out items from a Python set.
There are two ways to eliminate elements from a Python Set. One of them is the remove() function, and the other is the discard() function. If the element you are attempting to remove is not present in the set, the remove() function will cause an exception. However, the discard() function will not throw any exception in such cases. The code below will demonstrate these functions.
theSet = {1,2,3,4,5,6}
#remove 3 using discard() function
theSet.discard(3)
print(theSet)
#call discard() function again to remove 3
theSet.discard(3) #This won't raise any exception
print(theSet)
#call remove() function to remove 5
theSet.remove(5)
print(theSet)
#call remove() function to remove 5 again
theSet.remove(5) #this would raise exception
print(theSet) #this won't be printed
You will discover that the result appears similar.
================== RESTART: /home/imtiaz/set_del.py ==================
set([1, 2, 4, 5, 6])
set([1, 2, 4, 5, 6])
set([1, 2, 4, 6])
Traceback (most recent call last):
File "/home/imtiaz/set_del.py", line 16, in
theSet.remove(5) #this would raise exception
KeyError: 5
>>>
Python operations involving sets.
You may already have knowledge of mathematical set operations such as union, intersection, and difference. These operations can also be performed using Python sets. In this tutorial, we will acquire the skills to do so.
Union of Python Sets
The union operation combines two sets, creating a new set that includes all distinct elements from both sets. For instance, if we have two sets of {1, 2, 3, 4} and {2, 3, 5, 7}, performing the union operation would result in a new set of {1, 2, 3, 4, 5, 7}. This can be achieved by utilizing the union() function.
Python Set Intersection can be rephrased as finding the common elements between two sets in Python.
To obtain the shared distinct elements from two sets, we use the intersection operation. For instance, if we have sets {1, 2, 3, 4} and {2, 3, 5, 7}, their intersection would be {2, 3}. This intersection can be carried out using the intersection() function.
Difference of Sets in Python
The difference operation in Python compares two sets, A and B, and creates a new set that contains the items from set A which are not present in set B. For example, if we have set A = {1, 2, 3, 4} and set B = {2, 3, 5, 7}, the A – B operation will result in {1, 4}, while the B – A operation will result in {5, 7}. The difference operation can be performed using the difference() function in Python. The code below provides an example of how to perform these set operations in Python programming.
A = {1, 2, 3, 4} #initializing set A
B = {2, 3, 5, 7} #initializing set B
union_operation = A.union(B)
print("A union B :")
print(union_operation)
intersection_operation = A.intersection(B)
print("A intersection B :")
print(intersection_operation)
difference_operation = A.difference(B)
print("A-B :")
print(difference_operation)
difference_operation = B.difference(A)
print("B-A :")
print(difference_operation)
You will receive a result in this format.
================== RESTART: /home/imtiaz/set_op.py ==================
A union B :
set([1, 2, 3, 4, 5, 7])
A intersection B :
set([2, 3])
A-B :
set([1, 4])
B-A :
set([5, 7])
>>>
That concludes our discussion on Python Sets. We hope you have gained a good understanding. If you have any additional questions or need further assistance, please feel free to leave a comment. We will be glad to help. Source: Official Documentation
Other Python tutorials
breakpoint function in Python(Opens in a new browser tab)
The Python functions ord() and chr()(Opens in a new browser tab)
Python Substring refers to extracting a smaller portion of a string.(Opens in a new browser tab)