Python Substring refers to extracting a smaller portion of a string.
Python Substring is a section of a string. Python string offers different methods for generating a substring, verifying whether it contains a substring, finding the index of a substring, and more. Throughout this tutorial, we will explore different operations pertaining to substrings.
Python substring using a string
Firstly, let us examine two distinct methods of generating a substring.
Make a smaller part of a string.
One option for paraphrasing the given sentence natively could be:
String slicing enables us to generate a subset of characters from a string. By utilizing the split() function, we can create a collection of substrings by specifying a delimiter.
s = 'My Name is Pankaj'
# create substring using slice
name = s[11:]
print(name)
# list of substrings using split
l1 = s.split()
print(l1)
The result is as follows:
Pankaj
['My', 'Name', 'is', 'Pankaj']
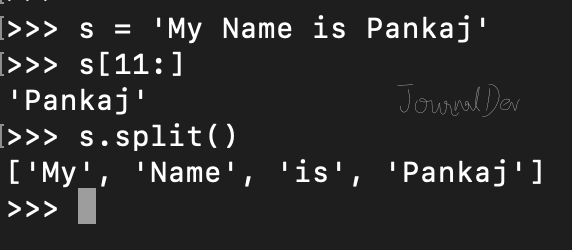
Verifying the presence of a substring.
To determine if a substring exists within a string, we have the option of using either the in operator or the find() function.
s = 'My Name is Pankaj'
if 'Name' in s:
print('Substring found')
if s.find('Name') != -1:
print('Substring found')
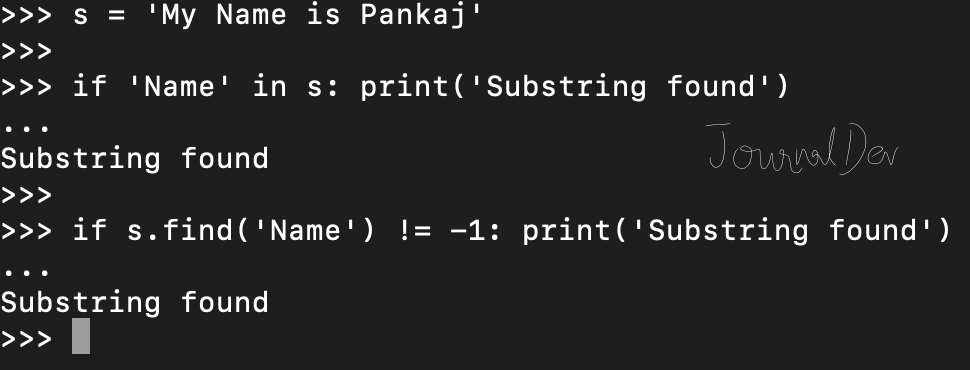
Number of times a substring appears
To determine the amount of times a substring appears within a string, we can make use of the count() function.
s = 'My Name is Pankaj'
print('Substring count =', s.count('a'))
s = 'This Is The Best Theorem'
print('Substring count =', s.count('Th'))
Provide one option for paraphrasing the following sentence natively:
“The result obtained or produced.”
Substring count = 3
Substring count = 3
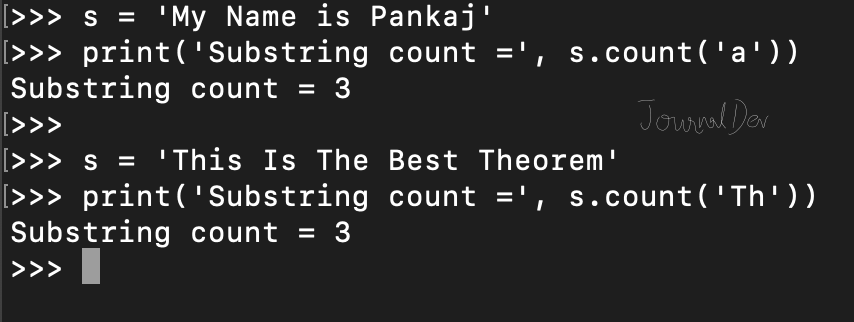
Locate every occurrence of the substring in the source string.
Although there is no predefined function to retrieve all the indexes for a given substring, we can create our own custom function by utilizing the find() function.
def find_all_indexes(input_str, substring):
l2 = []
length = len(input_str)
index = 0
while index < length:
i = input_str.find(substring, index)
if i == -1:
return l2
l2.append(i)
index = i + 1
return l2
s = 'This Is The Best Theorem'
print(find_all_indexes(s, 'Th'))
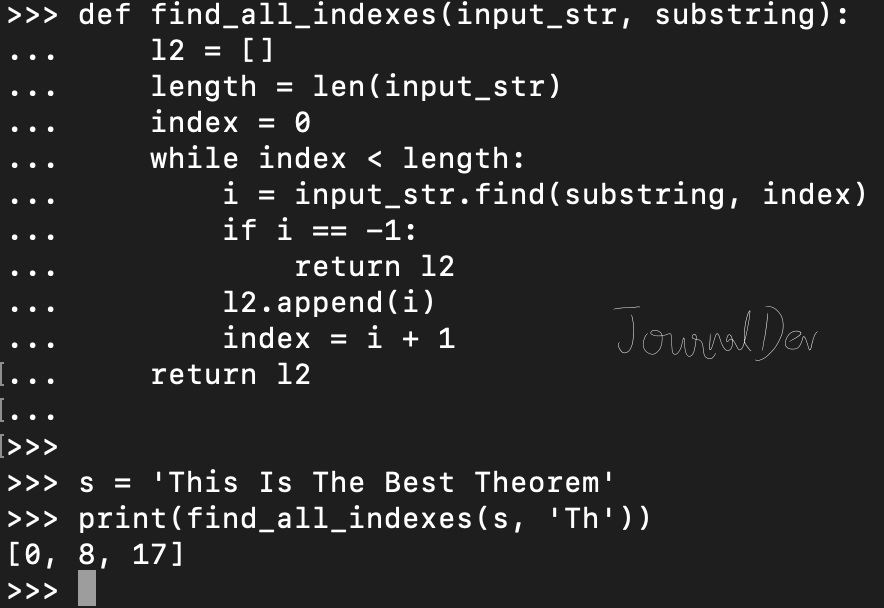
You have the option to access the entire Python script and more Python examples directly from our GitHub Repository.
More tutor about Python
The Python functions ord() and chr()(Opens in a new browser tab)