breakpoint function in Python
Python breakpoint() is a newly added function in Python 3.7 that aims to alleviate the challenges faced during code debugging. Traditionally, debugging Python code has been cumbersome due to the tight integration between the actual code and the debugging module code. For instance, using the pdb debugger would entail calling pdb.set_trace() within the program code. Conversely, if you opt for a different debugger like web-pdb, you would need to eliminate all PDB-related code and incorporate the web_pdb.set_trace() method. This process adds significant complexity to using the Python debugger and makes the code difficult to debug and maintain. To combat this issue, Python 3.7 introduced the breakpoint() method, which enables the creation of loosely coupled debugging code.
One potential paraphrase could be “Create a breakpoint in Python using the breakpoint() function.”
The breakpoint() function in Python calls the sys.breakpointhook() function, which by default calls pdb.set_trace() function. Thus, using breakpoint() simplifies the use of a debugger as we don’t need to import the pdb module explicitly. To illustrate the usage of the breakpoint() function, let’s consider the following code snippet in the python_breakpoint_examples.py script.
x = 10
y = 'Hi'
z = 'Hello'
print(y)
breakpoint()
print(z)
The PDB debugger console automatically opens when we run this script.
$python3.7 python_breakpoint_examples.py
Hi
> /Users/scdev/Documents/PycharmProjects/BasicPython/basic_examples/python_breakpoint_examples.py(8)()
-> print(z)
(Pdb) c
Hello
$

Stop debugging using the Python breakpoint() function.
The Python sys.breakpointhook() function relies on the environment variable PYTHONBREAKPOINT to customize the debugger. In case this variable is not specified, the default PDB debugger is utilized. If the variable is set to “0”, the function will promptly terminate and no debugging will occur. This feature is particularly useful when we need to execute our code without any debug assistance.
$PYTHONBREAKPOINT=0 python3.7 python_breakpoint_examples.py
Hi
Hello
$

The Debugger Module can be modified by using the Python breakpoint() function.
The PYTHONBREAKPOINT environment variable allows us to specify the debugger method to be used with the breakpoint() function. This is beneficial as it enables us to switch debugger modules effortlessly without altering any code. For instance, if we desire to utilize the web-pdb debugger, we can seamlessly integrate it into our program by setting PYTHONBREAKPOINT=web_pdb.set_trace. Before implementing this, ensure that web-pdb is installed by running the command pip3.7 install web-pdb.
The web-pdb documentation states that it is in line with the newly added breakpoint() function in Python 3.7.
$PYTHONBREAKPOINT=web_pdb.set_trace python3.7 python_breakpoint_examples.py
Hi
2018-08-10 12:49:54,339: root - web_console:110 - CRITICAL - Web-PDB: starting web-server on scdev:5555...
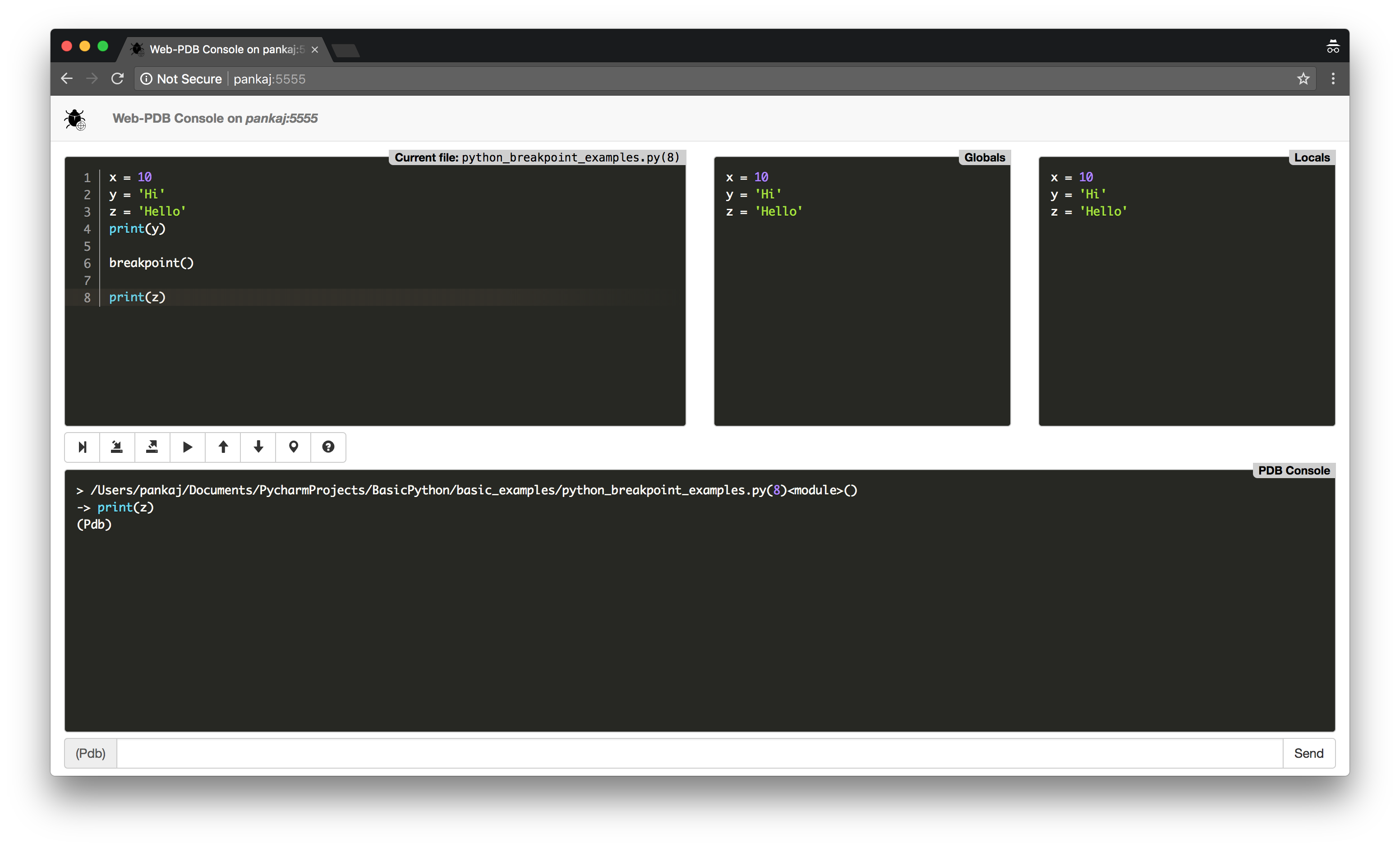
Can you please provide the specific content or sentence that needs to be paraphrased?
The Python breakpoint() function is a valuable addition to Python’s debugging capabilities. It is advisable to utilize this function when debugging as it allows for seamless integration of other third-party debuggers. Additionally, it offers a convenient way to deactivate the debugger and execute the program without interruption.
You have the option to explore additional Python examples on our GitHub Repository.
Source: The Offical Documentation
other Python tutorials
The Python functions ord() and chr()(Opens in a new browser tab)
Python Substring refers to extracting a smaller portion of a string.(Opens in a new browser tab)