Python HTTP requests such as GET and POST methods.
The Python HTTP module contains classes that handle the client-side of the HTTP and HTTPS protocols. In many programs, it is often combined with the urllib module to manage URL connections and interactions with HTTP requests. In this lesson, we will explore how to utilize a Python HTTP client to send HTTP requests, analyze response status, and retrieve response body data.
Python HTTP Client can be rephrased as “HTTP Client for Python.”
In this blog about the Python HTTP module, we will begin by exploring how to establish connections and make various types of HTTP requests such as GET, POST, and PUT. Let’s dive in.
Establishing HTTP Connections
Let’s begin by exploring the basic functionality of the HTTP module. With this module, establishing HTTP connections becomes effortless. Take a look at this sample program:
import http.client
connection = http.client.HTTPConnection('www.python.org', 80, timeout=10)
print(connection)
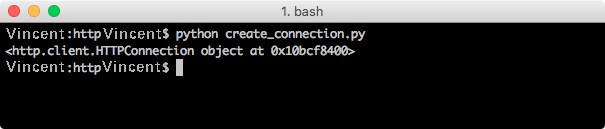
Python’s HTTP GET method
Next, we will utilize the HTTP client to retrieve a response and a status from a given URL. Here is an example of a code snippet that demonstrates this process.
import http.client
connection = http.client.HTTPSConnection("www.scdev.com")
connection.request("GET", "/")
response = connection.getresponse()
print("Status: {} and reason: {}".format(response.status, response.reason))
connection.close()
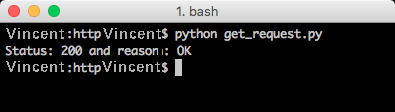
Are you encountering an SSL error code CERTIFICATE_VERIFY_FAILED?
When I initially ran the program, I encountered an error related to SSL certificates.
$ python3.6 http_client.py
Traceback (most recent call last):
File "http_client.py", line 4, in <module>
connection.request("GET", "/")
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/http/client.py", line 1239, in request
self._send_request(method, url, body, headers, encode_chunked)
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/http/client.py", line 1285, in _send_request
self.endheaders(body, encode_chunked=encode_chunked)
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/http/client.py", line 1234, in endheaders
self._send_output(message_body, encode_chunked=encode_chunked)
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/http/client.py", line 1026, in _send_output
self.send(msg)
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/http/client.py", line 964, in send
self.connect()
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/http/client.py", line 1400, in connect
server_hostname=server_hostname)
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/ssl.py", line 401, in wrap_socket
context=self, session=session)
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/ssl.py", line 808, in init
self.do_handshake()
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/ssl.py", line 1061, in do_handshake
self._sslobj.do_handshake()
File "/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6/ssl.py", line 683, in do_handshake
self._sslobj.do_handshake()
ssl.SSLError: [SSL: CERTIFICATE_VERIFY_FAILED] certificate verify failed (_ssl.c:748)
$
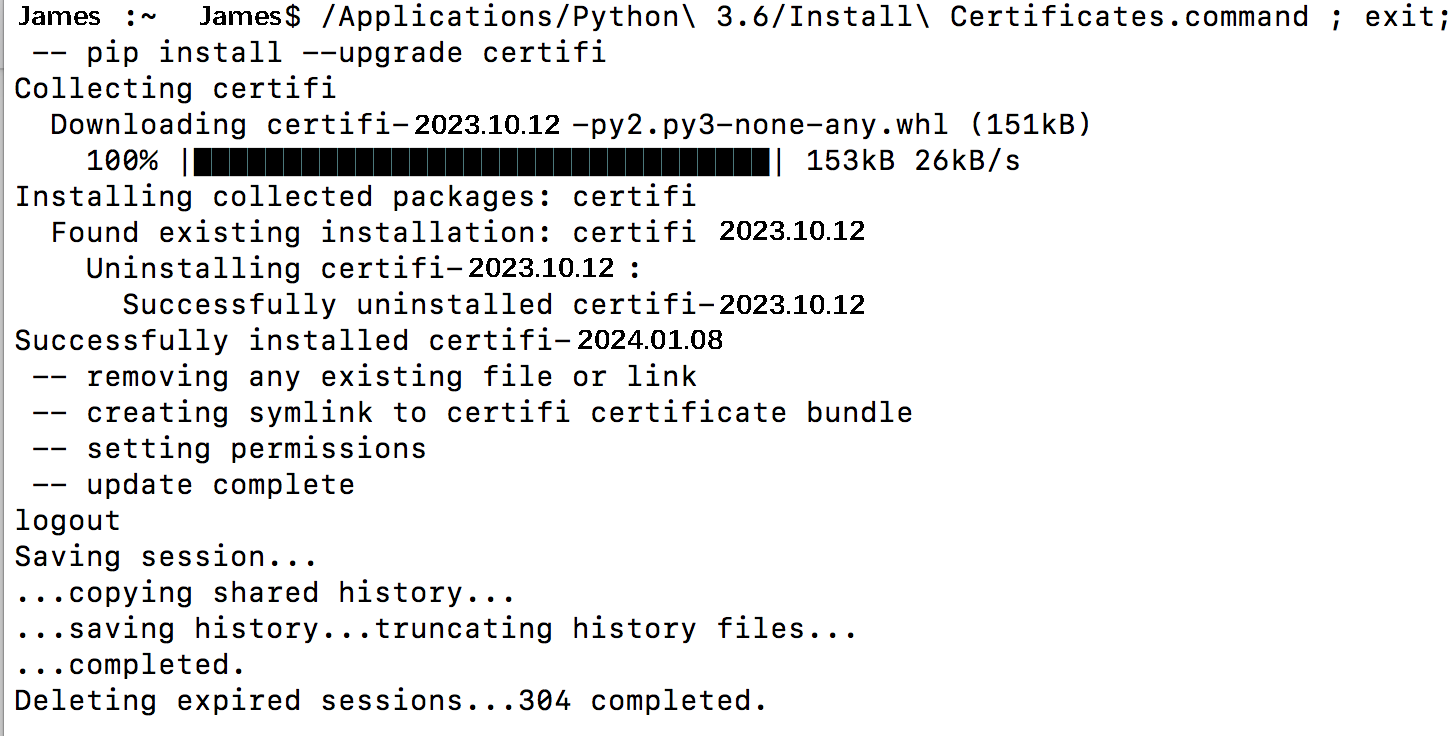

Obtaining the list of headers from the response.
Typically, the headers also include crucial details about the data type and response status in the received response. The response object itself provides access to a collection of headers. Here is a slightly modified version of the previous code snippet:
import http.client
import pprint
connection = http.client.HTTPSConnection("www.scdev.com")
connection.request("GET", "/")
response = connection.getresponse()
headers = response.getheaders()
pp = pprint.PrettyPrinter(indent=4)
pp.pprint("Headers: {}".format(headers))
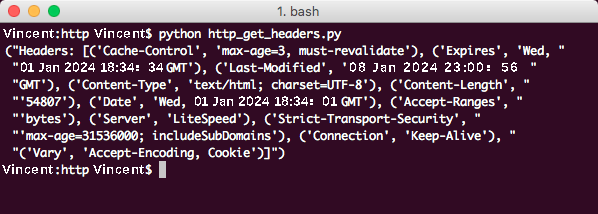
Sending an HTTP POST request using Python.
You can also utilize the HTTP module to send data to a URL and receive a response. Below is an example program:
import http.client
import json
conn = http.client.HTTPSConnection('www.httpbin.org')
headers = {'Content-type': 'application/json'}
foo = {'text': 'Hello HTTP #1 **cool**, and #1!'}
json_data = json.dumps(foo)
conn.request('POST', '/post', json_data, headers)
response = conn.getresponse()
print(response.read().decode())
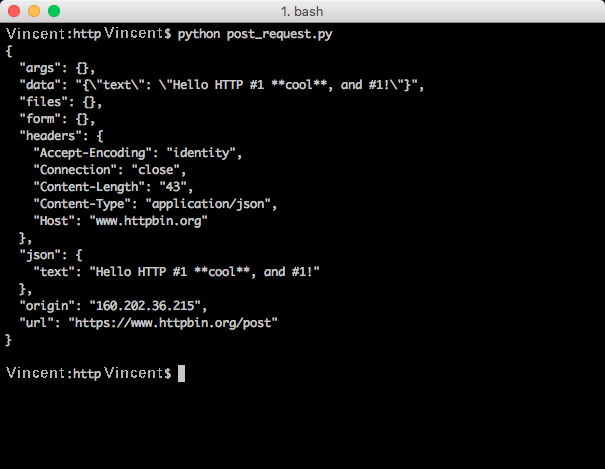
Making a PUT request in Python for HTTP.
Certainly, we can utilize the HTTP module to execute a PUT request within the same program. Let’s examine a code snippet for this purpose.
import http.client
import json
conn = http.client.HTTPSConnection('www.httpbin.org')
headers = {'Content-type': 'application/json'}
foo = {'text': 'Hello HTTP #1 **cool**, and #1!'}
json_data = json.dumps(foo)
conn.request("PUT", "/put", json_data)
response = conn.getresponse()
print(response.status, response.reason)
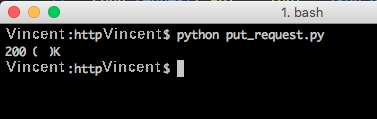
In conclusion
During this lesson, we have explored basic HTTP tasks conducted through http.client. Additionally, we can construct a Python-based HTTP server by utilizing the SimpleHTTPServer module. Reference: API Documentation.
see our other tutorials
Spring MVC HandlerInterceptorAdapter and HandlerInterceptor.(Opens in a new browser tab)
Interview Questions for Web Services – SOAP, RESTful(Opens in a new browser tab)
Server Configurations Frequently Used for Your Web Application(Opens in a new browser tab)