numpy.ones() in Python
The Python numpy.ones() function generates a fresh array with specific size and data type, where each element is assigned a value of 1. This function closely resembles the numpy zeros() function.
arguments of the numpy.ones() function
The syntax for the numpy.ones() function is as follows:
ones(shape, dtype=None, order='C')
- The shape is an int or tuple of ints to define the size of the array. If we just specify an int variable, a one-dimensional array will be returned. For a tuple of ints, the array of given shape will be returned.
- The dtype is an optional parameter with default value as a float. It’s used to specify the data type of the array, for example, int.
- The order defines the whether to store multi-dimensional array in row-major (C-style) or column-major (Fortran-style) order in memory.
Here is an example of how the Python numpy.ones() function can be used.
Let’s examine a few instances of building arrays using the numpy ones() function.
Creating an array with a single dimension, filled with 1s.
import numpy as np
array_1d = np.ones(3)
print(array_1d)
Result:
[1. 1. 1.]
Take note that the elements in the array have a default data type of float, which is why the values for “one” are displayed as 1.
2. Forming an array with multiple dimensions.
import numpy as np
array_2d = np.ones((2, 3))
print(array_2d)
Result:
[[1. 1. 1.]
[1. 1. 1.]]
A NumPy array with integer data type, consisting of all ones.
import numpy as np
array_2d_int = np.ones((2, 3), dtype=int)
print(array_2d_int)
The result:
[[1 1 1]
[1 1 1]]
4. Creating a NumPy array with a tuple data type and filled with ones.
We have the option to define the array elements as a tuple, along with their respective data types.
import numpy as np
array_mix_type = np.ones((2, 2), dtype=[('x', 'int'), ('y', 'float')])
print(array_mix_type)
print(array_mix_type.dtype)
Give me one option to paraphrase the following sentence natively: “Output.”
[[(1, 1.) (1, 1.)]
[(1, 1.) (1, 1.)]]
[('x', '<i8'), ('y', '<f8')]
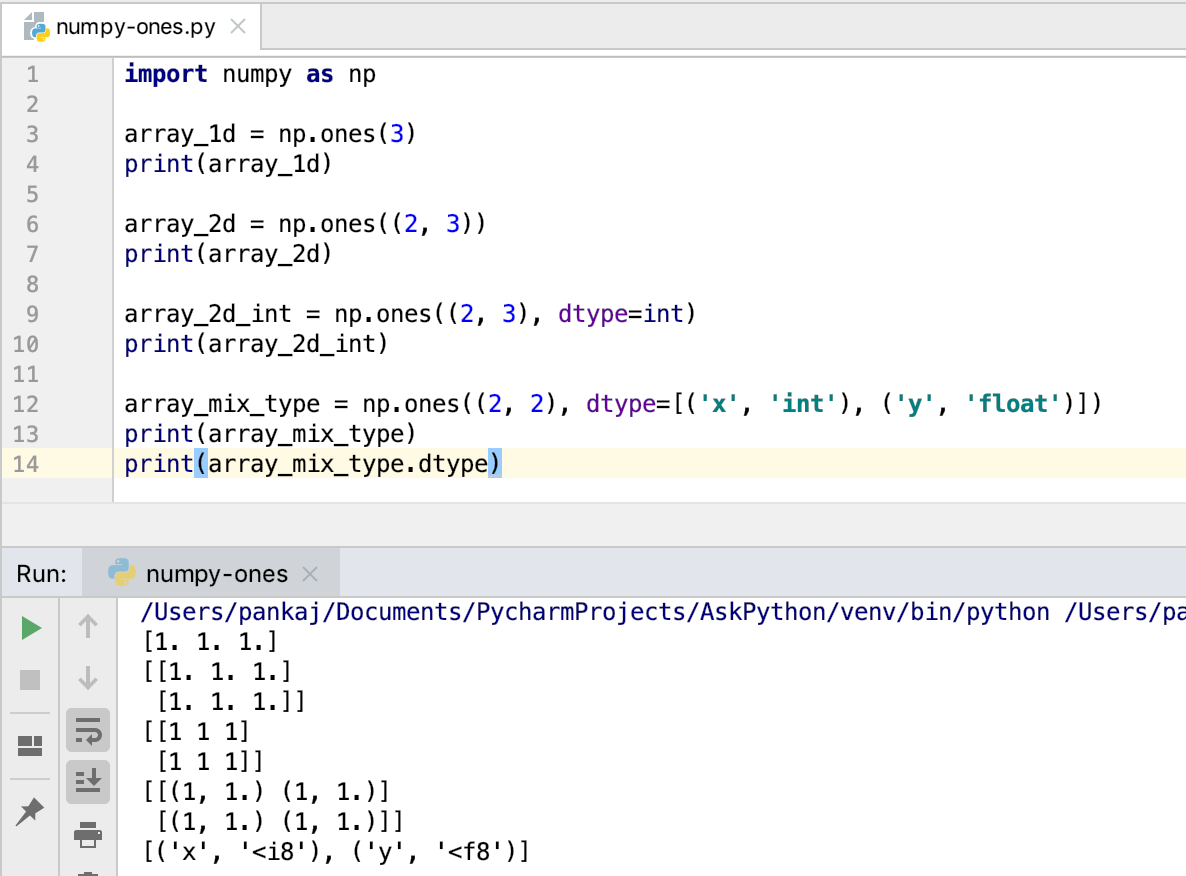
Source: Documentation for Application Programming Interface (API)
Paraphrased: Documentation about the Application Programming Interface (API) is being referenced.
more tutorials
A tutorial on the Python Pandas module.(Opens in a new browser tab)
convert string to character array in Java.(Opens in a new browser tab)
Converting string to array in the Java programming language(Opens in a new browser tab)