2D array for C++ implementation
In the beginning had been explained the introduction.
A two-dimensional array is the most basic type of a multi-dimensional array in C++. It can be thought of as an array made up of multiple arrays. The following illustration illustrates a two-dimensional array.
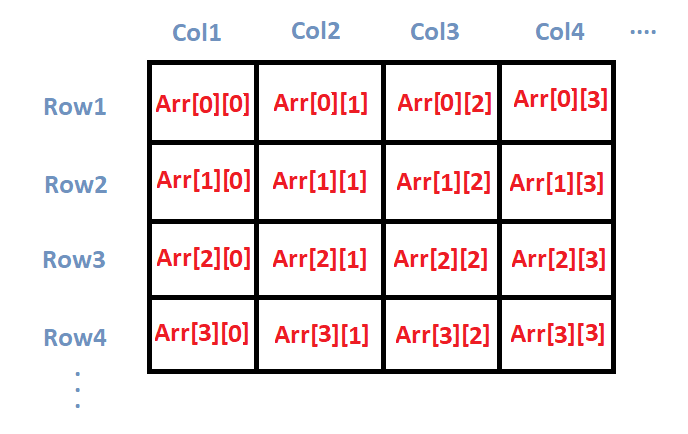
A matrix, which is also known as a two-dimensional array, can be of different types such as integer, character, float, etc., depending on the initialization. In the upcoming section, we will explore the methods of initializing 2D arrays.
Creating a 2-dimensional array in C++
So, how do we begin a two-dimensional array in C++? It’s as easy as this:
int arr[4][2] = {
{1234, 56},
{1212, 33},
{1434, 80},
{1312, 78}
} ;
So, to explain further, we create a two-dimensional array called “arr” that has four rows and two columns. Each element within the array is also an array, but this time it contains integers.
Another way to initialize a 2D array is by using the following method.
int arr[4][2] = {1234, 56, 1212, 33, 1434, 80, 1312, 78};
Also in this situation, arr is a 2D array containing 4 rows and 2 columns.
Printing a 2D Array in C++ can be rewritten as “Displaying a two-dimensional array in C++.”
We have finished the initialization of a 2D array, but without printing it, we cannot verify if it was done correctly.
Moreover, in numerous instances, it may be necessary to generate a printed 2D array following the execution of certain actions. How can we accomplish this?
Here’s one way we can accomplish that, as demonstrated in the code provided.
#include<iostream>
using namespace std;
main( )
{
int arr[4][2] = {
{ 10, 11 },
{ 20, 21 },
{ 30, 31 },
{ 40, 41 }
} ;
int i,j;
cout<<"Printing a 2D Array:\n";
for(i=0;i<4;i++)
{
for(j=0;j<2;j++)
{
cout<<"\t"<<arr[i][j];
}
cout<<endl;
}
}
Result:
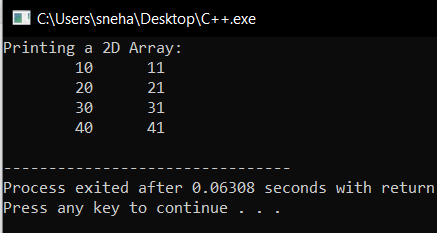
In the code provided above,
- We firstly initialize a 2D array, arr[4][2] with certain values,
- After that, we try to print the respective array using two for loops,
- the outer for loop iterates over the rows, while the inner one iterates over the columns of the 2D array,
- So, for each iteration of the outer loop, i increases and takes us to the next 1D array. Also, the inner loop traverses over the whole 1D array at a time,
- And accordingly, we print the individual element arr[ i ][ j ].
Receiving user input for the elements of a two-dimensional array.
Previously, we learned about initializing a 2D array with pre-set values. However, we can also allow the user to enter their own values. Now, let’s explore how to implement this.
#include<iostream>
using namespace std;
main( )
{
int s[2][2];
int i, j;
cout<<"\n2D Array Input:\n";
for(i=0;i<2;i++)
{
for(j=0;j<2;j++)
{
cout<<"\ns["<<i<<"]["<<j<<"]= ";
cin>>s[i][j];
}
}
cout<<"\nThe 2-D Array is:\n";
for(i=0;i<2;i++)
{
for(j=0;j<2;j++)
{
cout<<"\t"<<s[i][j];
}
cout<<endl;
}
}
Result:
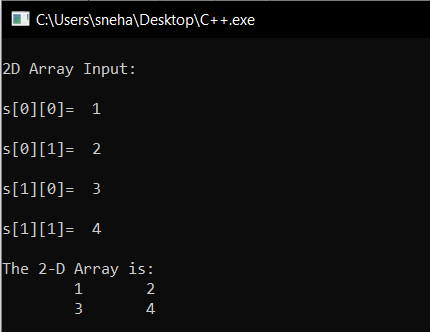
We start by declaring a 2X2 2D array called s in the given code. To fill up the array, we use two nested for loops to traverse through each element and take inputs from the user. Once the array is completely filled, we print it out to see the results.
How to perform matrix addition in C++ using two-dimensional arrays.
Let’s take a look at an example of how we can utilize 2D arrays to achieve matrix addition and display the outcome.
#include<iostream>
using namespace std;
main()
{
int m1[5][5], m2[5][5], m3[5][5];
int i, j, r, c;
cout<<"Enter the no.of rows of the matrices to be added(max 5):";
cin>>r;
cout<<"Enter the no.of columns of the matrices to be added(max 5):";
cin>>c;
cout<<"\n1st Matrix Input:\n";
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
cout<<"\nmatrix1["<<i<<"]["<<j<<"]= ";
cin>>m1[i][j];
}
}
cout<<"\n2nd Matrix Input:\n";
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
cout<<"\nmatrix2["<<i<<"]["<<j<<"]= ";
cin>>m2[i][j];
}
}
cout<<"\nAdding Matrices...\n";
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
m3[i][j]=m1[i][j]+m2[i][j];
}
}
cout<<"\nThe resultant Matrix is:\n";
for(i=0;i<r;i++)
{
for(j=0;j<c;j++)
{
cout<<"\t"<<m3[i][j];
}
cout<<endl;
}
}
Result:
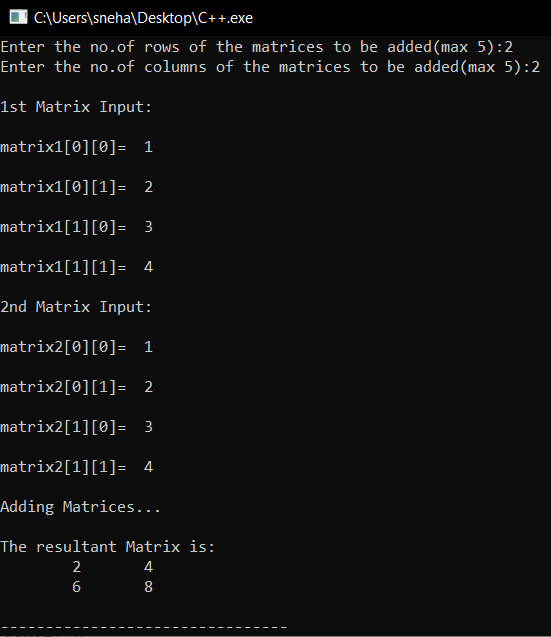
in this place
- We take two matrices m1 and m2 with a maximum of 5 rows and 5 columns. And another matrix m3 in which we are going to store the result,
- As user inputs, we took the number of rows and columns for both the matrices. Since we are performing matrix addition, the number of rows and columns should be the same for both the matrices,
- After that, we take both the matrices as user inputs, again using nested for loops,
- At this point, we have both the matrices m1 and m2,
- then we traverse through the m3 matrix, using two for loops and update the respective elements m3[ i ][ j ] by the value of m1[i][j]+m2[i][j]. In this way, by the end of the outer for loop, we get our desired matrix,
- At last, we print out the resultant matrix m3.
A native way to paraphrase the given statement in C++ would be: “Reference to a two-dimensional array in the C++ language.”
Can we have a pointer for an array if we can have pointers for integers, floats, and chars? The answer is yes and the following program demonstrates how to create and utilize it.
#include<iostream>
using namespace std;
/* Usage of pointer to an array */
main( )
{
int s[5][2] = {
{1, 2},
{1, 2},
{1, 2},
{1, 2}
} ;
int (*p)[2] ;
int i, j;
for (i = 0 ; i <= 3 ; i++)
{
p=&s[i];
cout<<"Row"<<i<<":";
for (j = 0; j <= 1; j++)
cout<<"\t"<<*(*p+j);
cout<<endl;
}
}
Can I get one alternative?
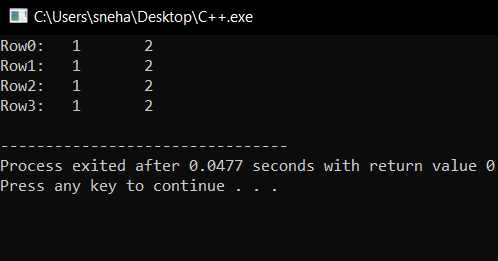
I only need one option for paraphrasing the following statement natively:
This is the place.
- In the above code, we try to print a 2D array using pointers,
- As we earlier did, at first we initialize the 2D array, s[5][2]. And also a pointer (*p)[2], where p is a pointer which stores the address of an array with 2 elements,
- As we already said, we can break down a 2D array as an array of arrays. So in this case, s is actually an array with 5 elements, which further are actually arrays with 2 elements for each row.
- We use a for loop to traverse over these 5 elements of the array, s. For each iteration, we assign p with the address of s[i],
- Further, the inner for loop prints out the individual elements of the array s[i] using the pointer p. Here, (*p + j) gives us the address of the individual element s[i][j], so using *(*p+j) we can access the corresponding value.
Passing a two-dimensional array as an argument to a function.
In this section, we will be taught the process of passing a 2D array to any function and accessing its respective elements. In the code provided, we pass the array ‘a’ to two functions, namely show() and print(), which then display the 2D array that was passed.
#include<iostream>
using namespace std;
void show(int (*q)[4], int row, int col)
{
int i, j ;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
cout<<"\t"<<*(*(q + i)+j);
cout<<"\n";
}
cout<<"\n";
}
void print(int q[][4], int row, int col)
{
int i, j;
for(i=0;i<row;i++)
{
for(j=0;j<col;j++)
cout<<"\t"<<q[i][j];
cout<<"\n";
}
cout<<"\n";
}
int main()
{
int a[3][4] = { 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21} ;
show (a, 3, 4);
print (a, 3, 4);
return 0;
}
Can you provide only one alternative paraphrase of the given sentence?
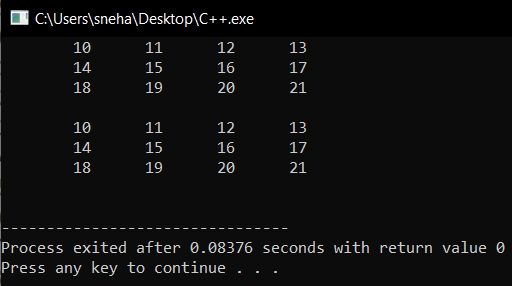
in this place
- In the show( ) function we have defined q to be a pointer to an array of 4 integers through the declaration int (*q)[4],
- q holds the base address of the zeroth 1-D array
- This address is then assigned to q, an int pointer, and then using this pointer all elements of the zeroth 1D array are accessed.
- Next time through the loop when i takes a value 1, the expression q+i fetches the address of the first 1-D array. This is because q is a pointer to the zeroth 1-D array and adding 1 to it would give us the address of the next 1-D array. This address is once again assigned to q and using it all elements of the next 1-D array are accessed
- In the second function print(), the declaration of q looks like this: int q[][4] ,
- This is same as int (*q )[4], where q is a pointer to an array of 4 integers. The only advantage is that we can now use the more familiar expression q[i][j] to access array elements. We could have used the same expression in show() as well but for better understanding of the use of pointers, we use pointers to access each element.
In conclusion,
In this article, we covered the topic of two-dimensional arrays in C++, exploring different operations that can be performed with them and their relevance in matrix addition. If you have any additional inquiries, please don’t hesitate to ask in the comments section.
Citations
- https://en.wikipedia.org/wiki/Array_data_structure
- /community/tutorials/arrays-in-c
more tutorials
convert string to character array in Java.(Opens in a new browser tab)
Creating RAID Arrays on Ubuntu 22.04 using mdadm(Opens in a new browser tab)
QR code generator in Java using zxing.(Opens in a new browser tab)
How to include items to a list in Python(Opens in a new browser tab)
Basics of Graph Plotting – Comprehending the plot() Function in R(Opens in a new browser tab)