Java’s Random Number Generator
Today, we are going to explore the process of generating a random number in Java. Occasionally, in Java programs, there arises a need to generate random numbers. This could be for various purposes such as playing a dice game or creating a random key id for encryption.
Java’s Random Number Generator
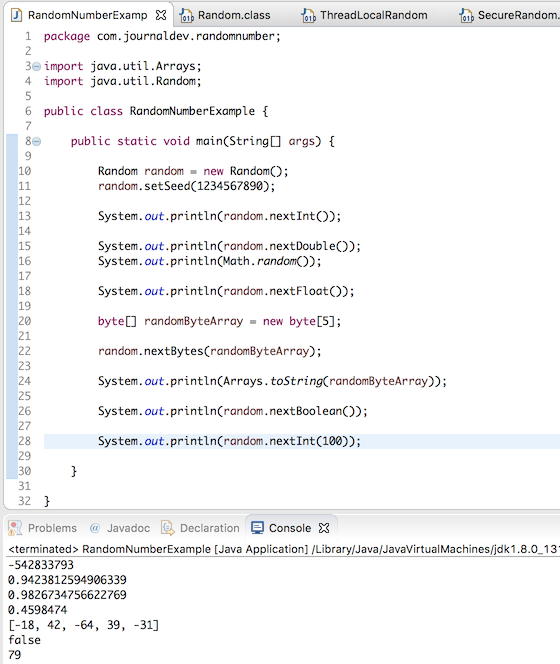
-
- The java.util.Random class is utilized for creating random numbers. It offers a variety of methods for generating random integers, longs, doubles, and so on.
-
- An alternative approach is to employ Math.random() in order to produce a double. This method internally relies on the Java Random class.
-
- When operating in a multithreaded environment, it is recommended to employ the java.util.concurrent.ThreadLocalRandom class. This class is a component of the Java Concurrent package and was introduced in Java 1.7. It features methods similar to those of the Java Random class.
- The java.security.SecureRandom class can be leveraged for generating highly secure random numbers. This class provides a random number generator with robust cryptographic capabilities. However, it may be slower in terms of processing. As a result, whether to employ it or not should be determined based on your application’s specific requirements.
Random number generator in Java
First, we will examine a few instances of generating a random number in Java. Afterwards, we will also examine a program that includes examples of ThreadLocalRandom and SecureRandom.
1. Create a random number that is an integer.
Random random = new Random();
int rand = random.nextInt();
Generating a random integer in Java is as straightforward as that. Upon creating the Random instance, a long seed value is automatically generated, which is utilized in all subsequent calls to the nextXXX methods. While it is possible to manually set this seed value in the program, it is often unnecessary in the majority of scenarios.
//set the long seed value using Random constructor
Random random = new Random(123456789);
//set long seed value using setter method
Random random1 = new Random();
random1.setSeed(1234567890);
Generate a random number between 1 and 10 in Java.
Occasionally, we may need to generate a random number within a specific range. In the case of a dice game, the potential values can range from 1 to 6 exclusively. The code provided below demonstrates how to generate a random number between 1 and 10, including both of these values.
Random random = new Random();
int rand = 0;
while (true){
rand = random.nextInt(11);
if(rand !=0) break;
}
System.out.println(rand);
The argument in the nextInt(int x) method is not considered, so we must input an argument as 11. Furthermore, since the generated random number includes 0, we need to keep calling the nextInt method until we obtain a value between 1 and 10. You can modify the provided code to generate a random number within any desired range.
Create a random double value.
In Java, we have the option to generate random double numbers by utilizing Math.random() or the nextDouble method from the Random class.
Random random = new Random();
double d = random.nextDouble();
double d1 = Math.random();
4. Produce a random decimal number.
Random random = new Random();
float f = random.nextFloat();
5. Create a random and lengthy value.
Random random = new Random();
long l = random.nextLong();
6. Create a random boolean value
Random random = new Random();
boolean flag = random.nextBoolean();
7. Create a byte array with randomly generated elements.
We have the ability to create unpredictable bytes and store them in a byte array provided by the user by utilizing the Random class. The quantity of random bytes generated matches the size of the byte array.
Random random = new Random();
byte[] randomByteArray = new byte[5];
random.nextBytes(randomByteArray);
System.out.println(Arrays.toString(randomByteArray)); // sample output [-70, -57, 74, 99, -78]
Using ThreadLocalRandom in a scenario with multiple threads
This is a basic illustration that demonstrates the utilization of ThreadLocalRandom in a concurrent setting.
package com.scdev.randomnumber;
import java.util.Random;
import java.util.concurrent.ThreadLocalRandom;
public class ThreadLocalRandomExample {
public static void main(String[] args) {
Runnable runnable = new MyRunnable();
for (int i = 0; i < 5; i++) {
Thread t = new Thread(runnable);
t.setName("MyRunnable-Thread-" + i);
t.start();
}
}
}
class MyRunnable implements Runnable {
@Override
public void run() {
String threadName = Thread.currentThread().getName();
System.out.println(threadName + "::" + ThreadLocalRandom.current().nextInt());
}
}
Here is an example result of running the program mentioned above.
MyRunnable-Thread-0::-1744088963
MyRunnable-Thread-3::139405798
MyRunnable-Thread-1::1403710182
MyRunnable-Thread-2::-1222080205
MyRunnable-Thread-4::-185825276
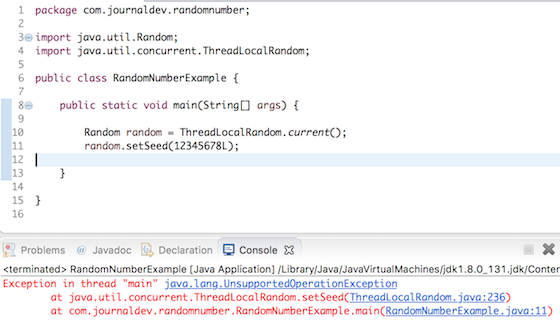
ThreadLocalRandom random = ThreadLocalRandom.current();
int rand = random.nextInt(1, 11);
ThreadLocalRandom offers comparable functionalities for generating random long and double values.
One option for paraphrasing “SecureRandom Example” natively could be: “An illustration showcasing the usage of SecureRandom.”
You have the option to utilize the SecureRandom class for generating highly secure random numbers using any of the providers mentioned. Here’s a brief code example demonstrating the usage of SecureRandom.
Random random = new SecureRandom();
int rand = random.nextInt();
System.out.println(rand);
This is everything regarding generating a random number in a Java program.
Our GitHub Repository offers the option to download the example code.
More tutorials
Converting string to array in the Java programming language(Opens in a new browser tab)
QR code generator in Java using zxing.(Opens in a new browser tab)
Python Substring refers to extracting a smaller portion of a string.(Opens in a new browser tab)
3 Simple Methods to Generate a Subset of a Python Dataframe(Opens in a new browser tab)
Addition Assignment Operator mean in Java(Opens in a new browser tab)