QR code generator in Java using zxing.
Today, our focus will be on the Java QR code generator program. If you keep up with technology and gadgets, then you probably know about QR codes. Nowadays, you can find them everywhere – on blogs, websites, and even in certain public locations. They are particularly popular in mobile apps, where you can scan a QR code using a QR code scanner app. This scan will either reveal the embedded text or direct you to a specific webpage if the QR code contains a URL. I recently came across this and found it fascinating. If you’re interested in learning more about QR codes, Wikipedia’s QR code page has a wealth of useful information.
A QR code generator written in Java.
After discovering QR code images on numerous websites, I began searching for a java QR code generator. Upon investigating various open source APIs, I concluded that zxing is the most user-friendly and uncomplicated option. If you intend to create a QR code image, you simply need to integrate its core library by incorporating the given dependency into your maven project.
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.3.2</version>
</dependency>
If you want to use the JavaSE library to read a QR image through the command line, you can include the following dependency.
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.3.2</version>
</dependency>
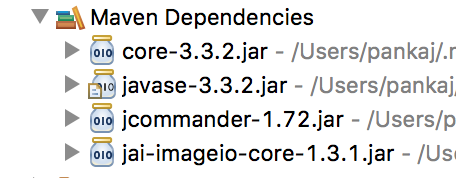
An example from zxing demonstrating the generation of a QR code image.
This is the program that you can utilize to produce an image of QR Code using zxing API. The file is named GenerateQRCode.java.
package com.scdev.qrcode.generator;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.Hashtable;
import javax.imageio.ImageIO;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
public class GenerateQRCode {
public static void main(String[] args) throws WriterException, IOException {
String qrCodeText = "https://www.scdev.com";
String filePath = "JD.png";
int size = 125;
String fileType = "png";
File qrFile = new File(filePath);
createQRImage(qrFile, qrCodeText, size, fileType);
System.out.println("DONE");
}
private static void createQRImage(File qrFile, String qrCodeText, int size, String fileType)
throws WriterException, IOException {
// Create the ByteMatrix for the QR-Code that encodes the given String
Hashtable<EncodeHintType, ErrorCorrectionLevel> hintMap = new Hashtable<>();
hintMap.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.L);
QRCodeWriter qrCodeWriter = new QRCodeWriter();
BitMatrix byteMatrix = qrCodeWriter.encode(qrCodeText, BarcodeFormat.QR_CODE, size, size, hintMap);
// Make the BufferedImage that are to hold the QRCode
int matrixWidth = byteMatrix.getWidth();
BufferedImage image = new BufferedImage(matrixWidth, matrixWidth, BufferedImage.TYPE_INT_RGB);
image.createGraphics();
Graphics2D graphics = (Graphics2D) image.getGraphics();
graphics.setColor(Color.WHITE);
graphics.fillRect(0, 0, matrixWidth, matrixWidth);
// Paint and save the image using the ByteMatrix
graphics.setColor(Color.BLACK);
for (int i = 0; i < matrixWidth; i++) {
for (int j = 0; j < matrixWidth; j++) {
if (byteMatrix.get(i, j)) {
graphics.fillRect(i, j, 1, 1);
}
}
}
ImageIO.write(image, fileType, qrFile);
}
}
This program has generated a QR Code image file, which you can test using your mobile QR Code scanner app. The QR Code should redirect you to the SC Home URL.
One possible paraphrase could be:
An instance of zxing being used as an illustration to decipher a QR code.
If you do not possess a mobile application to perform the testing, there is no need to worry. Through the command line, you can utilize the zxing API to scan QR codes. The provided command enables the reading of the QR code image file. Take note of the auxiliary jars in the classpath, which are essential for zxing to function properly.
$java -cp $HOME/.m2/repository/com/google/zxing/javase/3.3.2/javase-3.3.2.jar:.:$HOME/.m2/repository/com/google/zxing/core/3.3.2/core-3.3.2.jar:$HOME/.m2/repository/com/beust/jcommander/1.72/jcommander-1.72.jar:$HOME/.m2/repository/com/github/jai-imageio/jai-imageio-core/1.3.1/jai-imageio-core-1.3.1.jar com.google.zxing.client.j2se.CommandLineRunner JD.png
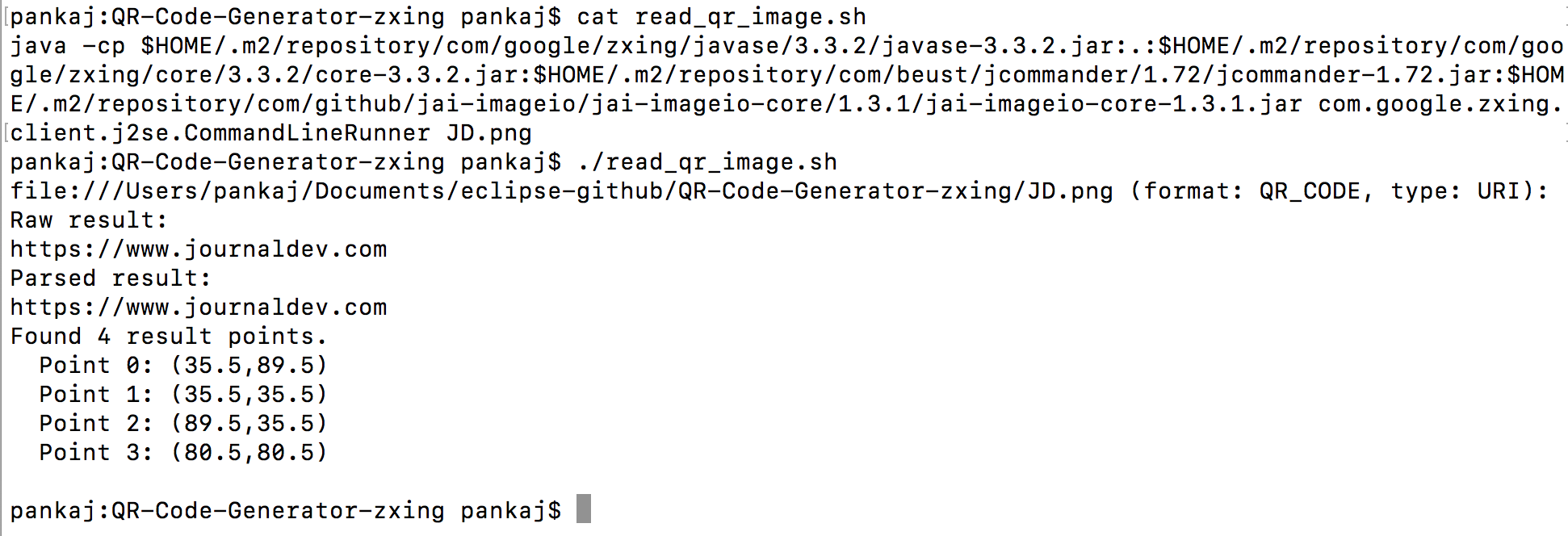
You have the option to obtain the QR Code Generator and Reader maven project from our GitHub Repository through download.
More Java Totor:
Addition Assignment Operator mean in Java(Opens in a new browser tab)
Tutorial on how to set up a Hibernate Tomcat JNDI DataSource.(Opens in a new browser tab)