Pythonファイル操作 – Pythonでファイルを読み書き
このチュートリアルでは、Pythonでのさまざまなファイル操作に取り組みます。ファイルの読み込み、書き込み、削除など、Pythonを使用する方法を説明します。では、遅延せずに始めましょう。
Pythonでファイルを操作する
前のチュートリアルでは、コンソールを使用して入力を行いました。今回は、ファイルを使用して入力を行います。つまり、ファイルから読み込んで書き込みを行います。これを行うためには、いくつかの手順を守る必要があります。
-
- ファイルを開く
-
- そのファイルからデータを入力する / そのファイルに出力を書き込む
- ファイルを閉じる
私たちは、ファイルのコピーと削除といった便利な操作方法も学びます。
なぜPythonでのファイル操作は必要か? (Naze Python de no fairu sōsa wa hitsuyō ka?)
機械学習の問題で大規模なデータセットを扱う場合、ファイル操作は基本的な必要条件です。Pythonはデータサイエンスで主要に使用される言語なので、Pythonが提供する異なるファイル操作に精通している必要があります。
それでは、ここでいくつかのPythonファイル操作を探索しましょう。
Pythonのopen()関数を使ってファイルを開く。
Pythonでファイルを扱うための最初のステップは、ファイルの開き方を学ぶことです。open()メソッドを使用してファイルを開くことができます。
Pythonのopen()関数は2つの引数を受け入れます。最初の引数はファイル名と完全なパスです。2番目の引数はファイルのオープンモードです。
以下に、ファイルの一般的な読み込みモードのいくつかをリストにしました。
- ‘r’ : This mode indicate that file will be open for reading only
- ‘w’ : This mode indicate that file will be open for writing only. If file containing containing that name does not exists, it will create a new one
- ‘a’ : This mode indicate that the output of that program will be append to the previous output of that file
- ‘r+’ : This mode indicate that file will be open for both reading and writing
また、Windowsオペレーティングシステムでは、バイナリファイルにアクセスするために「b」を付けることができます。これは、Windowsがバイナリテキストファイルと通常のテキストファイルを区別するためです。
仮に、コードが配置されているディレクトリと同じ場所に『file.txt』という名前のテキストファイルを配置したとします。それを開きたいとします。
ただし、open(ファイル名、モード)関数はファイルオブジェクトを返します。そのファイルオブジェクトを使用してさらなる操作を行うことができます。
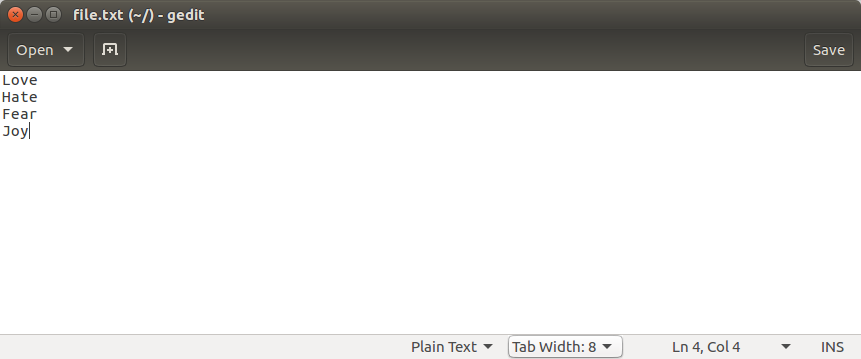
#directory: /home/imtiaz/code.py
text_file = open('file.txt','r')
#Another method using full location
text_file2 = open('/home/imtiaz/file.txt','r')
print('First Method')
print(text_file)
print('Second Method')
print(text_file2)
以下のコードの出力は次のようになります。
================== RESTART: /home/imtiaz/code.py ==================
First Method
Second Method
>>>
2. ファイルの読み書きをPythonで行う
Pythonでは、異なる方法でファイルの読み書きができるため、各関数が異なる振る舞いをします。重要な点は、ファイルの操作モードです。ファイルを読み込むには、読み込みモードまたは書き込みモードでファイルを開く必要があります。一方、Pythonでファイルに書き込むためには、ファイルを書き込みモードで開く必要があります。
以下は、ファイルの読み書きを可能にするPythonのいくつかの関数です。
- read() : This function reads the entire file and returns a string
- readline() : This function reads lines from that file and returns as a string. It fetch the line n, if it is been called nth time.
- readlines() : This function returns a list where each element is single line of that file.
- readlines() : This function returns a list where each element is single line of that file.
- write() : This function writes a fixed sequence of characters to a file.
- writelines() : This function writes a list of string.
- append() : This function append string to the file instead of overwriting the file.
「abc.txt」という例のファイルを使って、forループでファイルの各行を読み取りましょう。
#open the file
text_file = open('/Users/scdev/abc.txt','r')
#get the list of line
line_list = text_file.readlines();
#for each line from the list, print the line
for line in line_list:
print(line)
text_file.close() #don't forget to close the file
結果:
さて、Pythonでファイルの読み込み方法を知ったので、次にwritelines()関数を使って書き込み操作を行いましょう。
#open the file
text_file = open('/Users/scdev/file.txt','w')
#initialize an empty list
word_list= []
#iterate 4 times
for i in range (1, 5):
print("Please enter data: ")
line = input() #take input
word_list.append(line) #append to the list
text_file.writelines(word_list) #write 4 words to the file
text_file.close() #don’t forget to close the file
出力
3. Pythonのshutil()メソッドを使用してファイルをコピーする。
Pythonでファイルをコピーするためにshutilモジュールを使用できます。このユーティリティは、Pythonで異なるファイルのコピーおよび移動操作を行うことができます。以下の例を使って取り組みましょう。
import shutil
shutil.copy2('/Users/scdev/abc.txt', '/Users/scdev/abc_copy2.txt')
#another way to copy file
shutil.copyfile('/Users/scdev/abc.txt', '/Users/scdev/abc_copyfile.txt')
print("File Copy Done")
Pythonのshutil.os.remove()メソッドを使ってファイルを削除する。
Pythonのshutilモジュールは、remove()メソッドを提供しており、ファイルシステムからファイルを削除することができます。Pythonで削除操作を実行する方法を見てみましょう。
import shutil
import os
#two ways to delete file
shutil.os.remove('/Users/scdev/abc_copy2.txt')
os.remove('/Users/scdev/abc_copy2.txt')
5. Pythonのclose()メソッドを使用して、開いているファイルを閉じる。
Pythonでファイルを開いたら、変更を行った後に必ずファイルを閉じることが非常に重要です。これにより、以前に行った変更が保存され、ファイルはメモリから削除され、プログラム内でのさらなる読み書きが防止されます。
Pythonでオープンされているファイルを閉じるための構文。
fileobject.close()
前回の例から続けて、ファイルを読み込む方法を紹介しますが、ファイルを閉じる方法は以下の通りです。
text_file = open('/Users/scdev/abc.txt','r')
# some file operations here
text_file.close()
また、withブロックを使用すれば、手動でファイルを閉じる必要はありません。withブロックが実行されるとすぐに、ファイルは閉じられ、読み書きすることはできなくなります。
6. パイソンのファイルが見つかりませんでした。
Pythonでファイルを操作する際にFileNotFoundErrorを受け取ることは一般的です。ファイルオブジェクトを作成する際に、完全なファイルパスを指定することで簡単に回避することができます。
File "/Users/scdev/Desktop/string1.py", line 2, in <module>
text_file = open('/Users/scdev/Desktop/abc.txt','r')
FileNotFoundError: [Errno 2] No such file or directory: '/Users/scdev/Desktop/abc.txt'
FileNotFoundErrorを修正するには、単純に、ファイルを開くメソッドに指定したパスが正しいことを確認するだけです。
結論
これらはPythonにおけるファイル操作です。Python内でファイルを使用する方法は他にもたくさんありますが、CSVデータの読み取りなどが含まれます。こちらの記事では、PythonでPandasモジュールを使用してCSVデータセットを読み込む方法について紹介しています。
記事を読んで楽しんでいただけたと嬉しいです!学びを楽しんでくださいね 🙂
参照:
https://docs.python.org/3/tutorial/inputoutput.html#reading-and-writing-files