Pythonのnumpy.append()
Pythonのnumpyのappend()関数は、2つの配列を結合するために使用されます。この関数は新しい配列を返し、元の配列は変更されません。
NumPyのappend()の構文
関数の構文は次のようになります。
numpy.append(arr, values, axis=None)
- The arr can be an array-like object or a NumPy array. The values are appended to a copy of this array.
- The values are array-like objects and it’s appended to the end of the “arr” elements.
- The axis specifies the axis along which values are appended. If the axis is not provided, both the arrays are flattened.
Pythonのnumpy.append()の例
NumPyのappend()関数のいくつかの例を見てみましょう。
1. 2つの配列を平坦化する
import numpy as np
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[10, 20], [30, 40]])
# no axis provided, array elements will be flattened
arr_flat = np.append(arr1, arr2)
print(arr_flat) # [ 1 2 3 4 10 20 30 40]
2. 軸に沿って統合
import numpy as np
arr_merged = np.append([[1, 2], [3, 4]], [[10, 20], [30, 40]], axis=0)
print(f'Merged 2x2 Arrays along Axis-0:\n{arr_merged}')
arr_merged = np.append([[1, 2], [3, 4]], [[10, 20], [30, 40]], axis=1)
print(f'Merged 2x2 Arrays along Axis-1:\n{arr_merged}')
出力:
Merged 2x2 Arrays along Axis-0:
[[ 1 2]
[ 3 4]
[10 20]
[30 40]]
Merged 2x2 Arrays along Axis-1:
[[ 1 2 10 20]
[ 3 4 30 40]]
- When the 2×2 arrays are merged along the x-axis, the output array size is 4×2.
- When the 2×2 arrays are merged along the y-axis, the output array size is 2×4.
3. 異なる形状の配列の結合
append()関数は、軸を除いた場合に両方の配列の形状が異なる場合にValueErrorを送出します。単純な例を使ってこのシナリオを理解しましょう。
arr3 = np.append([[1, 2]], [[1, 2, 3], [1, 2, 3]])
print(arr3)
arr3 = np.append([[1, 2]], [[1, 2], [3, 4]], axis=0)
print(arr3)
- In the first example, the array elements are flattened. So even if they have completely different size – 1×2 and 2×3, the append() works fine.
- In the second example, the array shapes are 1×2 and 2×2. Since we are appending along the 0-axis, the 0-axis shape can be different. The other shapes should be the same, so this append() will also work fine.
結果:
[1 2 1 2 3 1 2 3]
[[1 2]
[1 2]
[3 4]]
別の例を見てみましょう。ValueErrorが発生する場合を考えましょう。
>>> import numpy as np
>>>
>>> arr3 = np.append([[1, 2]], [[1, 2, 3]], axis=0)
Traceback (most recent call last):
File "", line 1, in
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/site-packages/numpy/lib/function_base.py", line 4528, in append
return concatenate((arr, values), axis=axis)
ValueError: all the input array dimensions except for the concatenation axis must match exactly
>>>
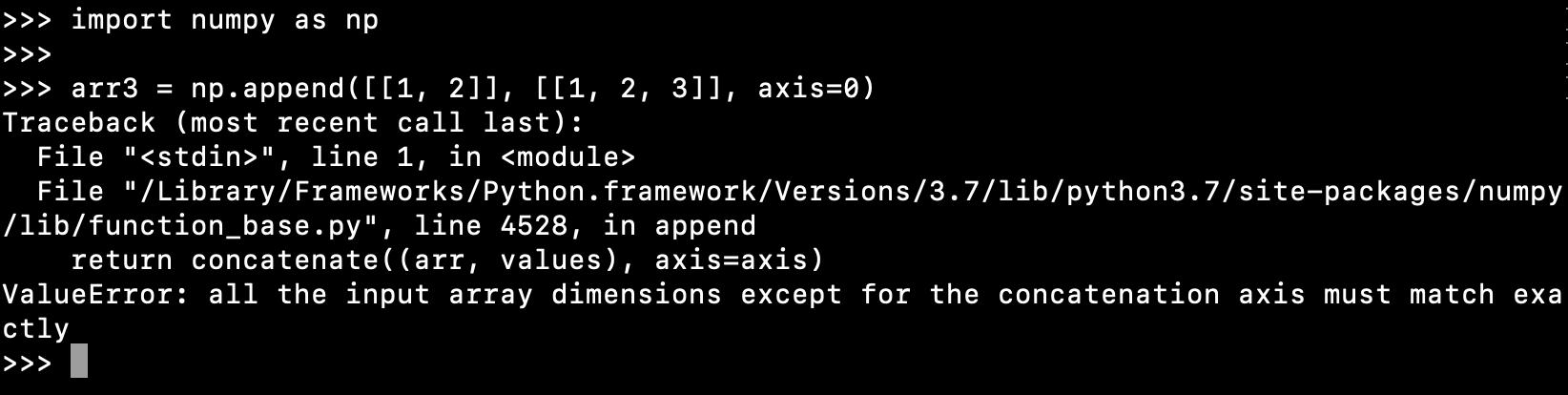
配列の形状は1×2と2×3です。axis-1の形状が異なるため、ValueErrorが発生します。参照:APIドキュメント