Pythonのforループ[簡単な例で]
Pythonのforループは反復処理を行う関数です。リストのようなシーケンスオブジェクトがあれば、forループを使ってリスト内のアイテムを一つずつ反復処理することができます。
forループの機能は、他の多くのプログラミング言語で見られるものとあまり変わりません。
この記事では、Pythonのforループについて詳しく説明し、リストやタプルなどの異なるシーケンスを繰り返し処理する方法を学びます。さらに、break文やcontinue文を使用してループのフローを制御する方法も学びます。
Pythonのforループの基本構文
Pythonのforループの基本的な構文は、以下に示すものと似ています。
for itarator_variable in sequence_name:
Statements
. . .
Statements
Pythonのforループの構文をより詳しく説明させてください。
- The first word of the statement starts with the keyword “for” which signifies the beginning of the for loop.
- Then we have the iterator variable which iterates over the sequence and can be used within the loop to perform various functions
- The next is the “in” keyword in Python which tells the iterator variable to loop for elements within the sequence
- And finally, we have the sequence variable which can either be a list, a tuple, or any other kind of iterator.
- The statements part of the loop is where you can play around with the iterator variable and perform various function
1. フォーループを使用して、文字列の個々の文字を出力する。
Pythonの文字列は、文字の並びです。プログラミングのアプリケーション内で文字列の各文字を個別に処理する必要がある場合は、ここでforループを使用することができます。
あなたにとってのその結果の進め方は次のようになります。
word="anaconda"
for letter in word:
print (letter)
出力:
a
n
a
c
o
n
d
a
このループが機能する理由は、Pythonが文字列を一つの単位ではなく、文字の連続として扱っているからです。
2. Pythonのリストやタプルをforループを使って反復処理する。
リストとタプルはイテラブルなオブジェクトです。では、これらのオブジェクト内の要素をループで処理する方法を見てみましょう。
words= ["Apple", "Banana", "Car", "Dolphin" ]
for word in words:
print (word)
出力:
Apple
Banana
Car
Dolphin
さて、ここではタプルの要素をループ処理する作業に進みましょう。
nums = (1, 2, 3, 4)
sum_nums = 0
for num in nums:
sum_nums = sum_nums + num
print(f'Sum of numbers is {sum_nums}')
# Output
# Sum of numbers is 10
3. ネストされたPythonのforループ
もし私たちが一つのforループの中に別のforループを持っている場合、それはネストされたforループと呼ばれます。ネストされたforループには複数の応用があります。
上記のリストの例を考えてみてください。forループはリストから個々の単語を出力しますが、リスト内の各単語の個々の文字を出力したい場合はどうすればよいでしょうか?
これはネストされたforループがより適している場所です。第一のループ(親ループ)では単語を一つずつ確認します。第二のループ(子ループ)では各単語の文字をループします。
words= ["Apple", "Banana", "Car", "Dolphin" ]
for word in words:
#This loop is fetching word from the list
print ("The following lines will print each letters of "+word)
for letter in word:
#This loop is fetching letter for the word
print (letter)
print("") #This print is used to print a blank line
出力
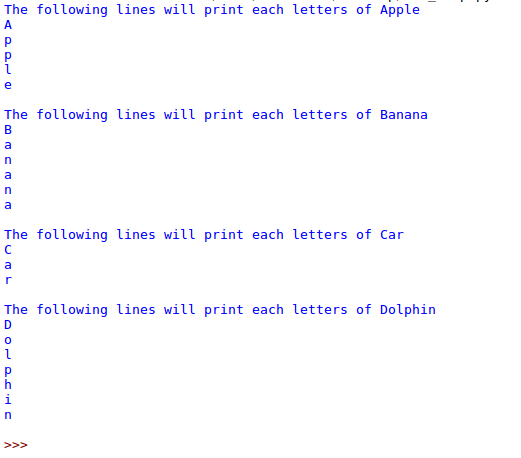
4. range()関数を使用したPythonのforループ
Pythonのrange()は組み込み関数の一つです。forループを特定の回数実行したい場合や、出力するオブジェクトの範囲を指定する必要がある場合に、range関数が非常に便利です。以下の例を考えてみましょう。1、2、3の数値を出力したい場合です。
for x in range(3):
print("Printing:", x)
# Output
# Printing: 0
# Printing: 1
# Printing: 2
範囲関数は、開始と停止以外にもう一つのパラメーターを取ります。それがステップパラメーターです。このパラメーターは、範囲関数に対して各カウントの間にスキップする数字の数を示します。
以下の例では、ステップとして数字3を使用しました。そして、出力される数字は前の数字に3を加えたものです。
for n in range(1, 10, 3):
print("Printing with step:", n)
# Output
# Printing with step: 1
# Printing with step: 4
# Printing with step: 7
5. forループの中のbreak文
「break文は、forループを途中で終了させるために使用されます。特定の条件が満たされた場合、forループを中断させるために使用されます。」
仮に数値のリストがあるとしましょう。その中に特定の数値が含まれているかどうかを確認したい場合、数値のリストを順番に調べ、もし数値が見つかればループを抜け出します。なぜなら、残りの要素を繰り返し確認する必要がないからです。
この場合、forループと一緒にPythonのif else条件を使用します。
nums = [1, 2, 3, 4, 5, 6]
n = 2
found = False
for num in nums:
if n == num:
found = True
break
print(f'List contains {n}: {found}')
# Output
# List contains 2: True
6. ループの中でのcontinue文。
特定の条件に対して、forループ内でcontinue文を使用して、forループ本体の実行をスキップすることができます。
数字のリストがあり、正の数の合計を表示したいとします。負の数の場合は継続文を使用してforループをスキップできます。
nums = [1, 2, -3, 4, -5, 6]
sum_positives = 0
for num in nums:
if num < 0:
continue
sum_positives += num
print(f'Sum of Positive Numbers: {sum_positives}')
6. それに対応する else ブロックを持つ Python の for ループ。
Pythonのforループでは、elseブロックを使うことができます。elseブロックは、forループがbreak文によって終了しなかった場合にのみ実行されます。
仮に、全ての数字が偶数の場合にのみ、数字の合計を出力する関数があるとしましょう。
奇数が存在する場合は、break文を使用してforループを終了させることができます。通常の実行時にのみ出力されるように、else部分で和を表示することができます。
def print_sum_even_nums(even_nums):
total = 0
for x in even_nums:
if x % 2 != 0:
break
total += x
else:
print("For loop executed normally")
print(f'Sum of numbers {total}')
# this will print the sum
print_sum_even_nums([2, 4, 6, 8])
# this won't print the sum because of an odd number in the sequence
print_sum_even_nums([2, 4, 5, 8])
# Output
# For loop executed normally
# Sum of numbers 20
結論
Pythonのforループは、他のプログラミング言語と非常に似ています。forループでは、breakやcontinueステートメントを使用して実行を変更することができます。ただし、Pythonでは、forループにオプションのelseブロックを持つこともできます。
上記のチュートリアルからいくつかの興味深いアイデアを得られたと願っています。もし質問がありましたら、下のコメント欄で教えてください。