Pythonの文字列を整数に変換する方法と、整数を文字列に変換する方法
このチュートリアルでは、Pythonの文字列を整数に変換する方法と整数を文字列に変換する方法を学びます。前のチュートリアルでは、Pythonのリストのappend関数について学びました。
Pythonの文字列を整数に変換する
私たちの前のチュートリアルを読んだ場合、この変換を使用したことに気づくかもしれません。実際に、これは多くの場合に必要です。例えば、ファイルからデータを読み取っている場合、それはString形式であり、Stringをintに変換する必要があります。それでは、直接コードに移りましょう。文字列で表されている数値をintに変換したい場合は、int()関数を使用する必要があります。以下の例をご覧ください。
num = '123' # string data
# print the type
print('Type of num is :', type(num))
# convert using int()
num = int(num)
# print the type again
print('Now, type of num is :', type(num))
以下のコードの出力は、次のようになります。
Type of num is : <class 'str'>
Now, type of num is : <class 'int'>
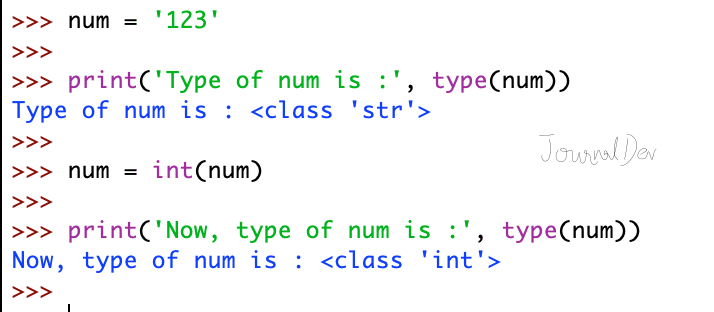
異なる基数からの文字列をintに変換する
もしintに変換したい文字列が10進数以外の異なる基数に属している場合、変換のために基数を指定することができます。ただし、出力される整数は常に10進数です。もう一つ覚えておくべきことは、与えられた基数は2から36の間である必要があるということです。文字列をintに変換する際の基数引数の例を以下に示します。
num = '123'
# print the original string
print('The original string :', num)
# considering '123' be in base 10, convert it to base 10
print('Base 10 to base 10:', int(num))
# considering '123' be in base 8, convert it to base 10
print('Base 8 to base 10 :', int(num, base=8))
# considering '123' be in base 6, convert it to base 10
print('Base 6 to base 10 :', int(num, base=6))

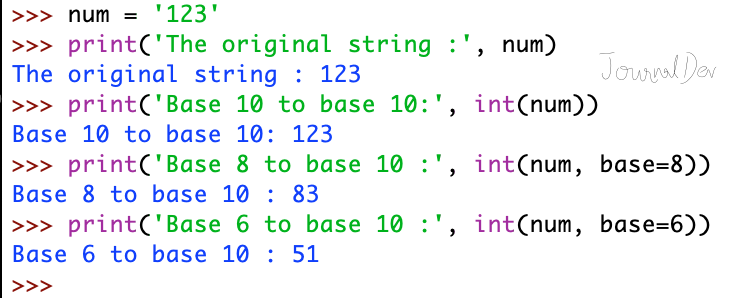
「Stringをintに変換する際にValueErrorが発生しました。」
文字列から整数に変換する際に、ValueError例外が発生することがあります。この例外は、変換したい文字列が数値を表していない場合に発生します。例えば、16進数を整数に変換したいとします。しかし、int()関数にbase=16という引数を渡していない場合、10進数の数字に属さない数字が含まれている場合にValueError例外が発生します。以下の例は、文字列を整数に変換する際にこの例外を示します。
"""
Scenario 1: The interpreter will not raise any exception but you get wrong data
"""
num = '12' # this is a hexadecimal value
# the variable is considered as decimal value during conversion
print('The value is :', int(num))
# the variable is considered as hexadecimal value during conversion
print('Actual value is :', int(num, base=16))
"""
Scenario 2: The interpreter will raise ValueError exception
"""
num = '1e' # this is a hexadecimal value
# the variable is considered as hexadecimal value during conversion
print('Actual value of \'1e\' is :', int(num, base=16))
# the variable is considered as decimal value during conversion
print('The value is :', int(num)) # this will raise exception
上記のコードの出力は次のようになります。
The value is : 12
Actual value is : 18
Actual value of '1e' is : 30
Traceback (most recent call last):
File "/home/imtiaz/Desktop/str2int_exception.py", line 22, in
print('The value is :', int(num)) # this will raise exception
ValueError: invalid literal for int() with base 10: '1e'
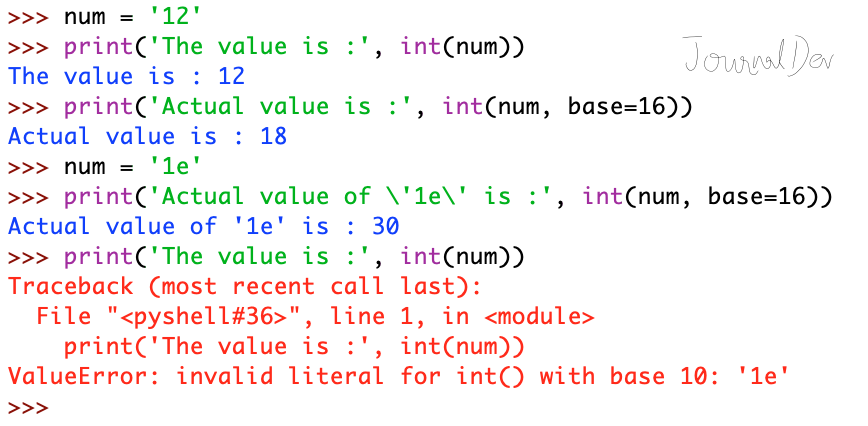
Pythonの整数を文字列に変換する方法
intを文字列に変換するのは、努力やチェックが必要ありません。変換するためには、単純にstr()関数を使用するだけです。以下の例をご覧ください。
hexadecimalValue = 0x1eff
print('Type of hexadecimalValue :', type(hexadecimalValue))
hexadecimalValue = str(hexadecimalValue)
print('Type of hexadecimalValue now :', type(hexadecimalValue))
以下のコードの出力は次の通りです。
Type of hexadecimalValue : <class 'int'>
Type of hexadecimalValue now : <class 'str'>
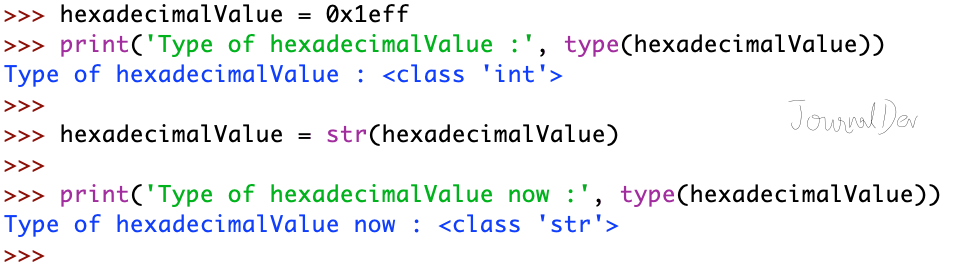
Pythonにおける文字列から整数への変換と整数から文字列への変換についての説明は以上です。参考:Python公式ドキュメント