Pythonのソケットプログラミング-サーバー、クライアントの例
皆さん、こんにちは!前回のチュートリアルでは、Pythonのunittestモジュールについて話しました。今日は、Pythonのソケットプログラミングの例を見ていきます。Pythonのソケットサーバーとクライアントのアプリケーションを作成します。
Pythonのソケットプログラミング
Pythonソケットプログラミングを理解するためには、Socket Server、Socket Client、Socketという3つの興味深いトピックについて知る必要があります。では、サーバは何でしょうか?サーバは、クライアントのリクエストを待ち、それに応じて処理または提供するソフトウェアです。一方、クライアントはこのサービスの要求者です。クライアントプログラムはサーバにリソースを要求し、サーバはその要求に応答します。Socketは、サーバとクライアントの間の双方向通信チャネルのエンドポイントです。Socketは、プロセス内、同じマシン上のプロセス間、または異なるマシン上のプロセス間で通信することができます。リモートプログラムとの通信には、ソケットポートを介して接続する必要があります。このソケットプログラミングチュートリアルの主な目的は、ソケットサーバとクライアントが互いに通信する方法を紹介することです。また、Pythonのソケットサーバプログラムの書き方も学びます。
Pythonのソケットの例
以前に述べたように、ソケットクライアントはソケットサーバーに対してリソースの要求を行い、サーバーはその要求に応答すると述べました。したがって、私たちはサーバーとクライアントのモデルを設計し、それぞれが通信できるようにします。以下の手順で考えることができます。
-
- 最初にPythonのソケットサーバープログラムが実行され、リクエストを待ちます。
-
- Pythonのソケットクライアントプログラムが最初に会話を開始します。
-
- その後、サーバープログラムはクライアントの要求に応じて適切な応答を返します。
- ユーザーが「さようなら」と入力した場合、クライアントプログラムは終了します。サーバープログラムもクライアントプログラムの終了時に終了しますが、これはオプションであり、サーバープログラムを無期限に実行したり、クライアントの要求に特定のコマンドで終了することもできます。
Pythonのソケットサーバー
Pythonのソケットサーバープログラムをsocket_server.pyとして保存します。Pythonのソケット接続を使用するためには、socketモジュールをインポートする必要があります。そして、順番にいくつかのタスクを実行してサーバーとクライアントの間に接続を確立する必要があります。socket.gethostname()関数を使用することでホストアドレスを取得できます。ポート番号は、1024より大きい値を使用することが推奨されています。なぜなら、1024未満のポート番号は標準のインターネットプロトコルのために予約されているからです。以下のPythonのソケットサーバーの例コードをご覧ください。コメントがコードを理解するのに役立ちます。
import socket
def server_program():
# get the hostname
host = socket.gethostname()
port = 5000 # initiate port no above 1024
server_socket = socket.socket() # get instance
# look closely. The bind() function takes tuple as argument
server_socket.bind((host, port)) # bind host address and port together
# configure how many client the server can listen simultaneously
server_socket.listen(2)
conn, address = server_socket.accept() # accept new connection
print("Connection from: " + str(address))
while True:
# receive data stream. it won't accept data packet greater than 1024 bytes
data = conn.recv(1024).decode()
if not data:
# if data is not received break
break
print("from connected user: " + str(data))
data = input(' -> ')
conn.send(data.encode()) # send data to the client
conn.close() # close the connection
if __name__ == '__main__':
server_program()
私たちのPythonソケットサーバーはポート5000で実行され、クライアントの要求を待ちます。クライアント接続が閉じられたときにサーバーが終了しないようにするには、if条件とbreak文を削除してください。Pythonのwhileループは、サーバープログラムを無期限に実行し、クライアントの要求を待ち続けるために使用されます。
Pythonのソケットクライアント
私たちはPythonのソケットクライアントプログラムをsocket_client.pyとして保存します。このプログラムは、サーバープログラムと似ていますが、バインディングはありません。サーバープログラムとクライアントプログラムの主な違いは、サーバープログラムではホストアドレスとポートアドレスを結び付ける必要があることです。以下のPythonソケットクライアントのサンプルコードをご覧ください。コメントがコードの理解に役立つでしょう。
import socket
def client_program():
host = socket.gethostname() # as both code is running on same pc
port = 5000 # socket server port number
client_socket = socket.socket() # instantiate
client_socket.connect((host, port)) # connect to the server
message = input(" -> ") # take input
while message.lower().strip() != 'bye':
client_socket.send(message.encode()) # send message
data = client_socket.recv(1024).decode() # receive response
print('Received from server: ' + data) # show in terminal
message = input(" -> ") # again take input
client_socket.close() # close the connection
if __name__ == '__main__':
client_program()
Pythonのソケットプログラミングの出力
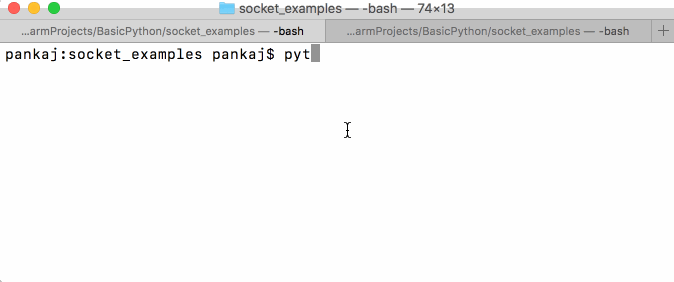
scdev$ python3.6 socket_server.py
Connection from: ('127.0.0.1', 57822)
from connected user: Hi
-> Hello
from connected user: How are you?
-> Good
from connected user: Awesome!
-> Ok then, bye!
scdev$
scdev$ python3.6 socket_client.py
-> Hi
Received from server: Hello
-> How are you?
Received from server: Good
-> Awesome!
Received from server: Ok then, bye!
-> Bye
scdev$
ソケットサーバーはポート5000で実行されていることに注意してください。しかし、クライアントもサーバーに接続するためにソケットポートを必要とします。このポートはクライアントの接続呼び出しによってランダムに割り当てられます。この場合、ポート番号は57822です。以上がPythonのソケットプログラミング、Pythonソケットサーバー、ソケットクライアントの例プログラムについての説明です。参考:公式ドキュメント