Pythonにおける「not equal(等しくない)」の演算子
Pythonの「not equal」演算子は、2つの変数が同じ型であり異なる値を持つ場合にTrueを返します。値が同じ場合はFalseを返します。Pythonは動的かつ強く型付けされた言語であるため、2つの変数が同じ値を持つが型が異なる場合、不等号演算子はTrueを返します。
Pythonにおける等しくない演算子
Operator | Description |
---|---|
!= | Not Equal operator, works in both Python 2 and Python 3. |
<> | Not equal operator in Python 2, deprecated in Python 3. |
Python 2 の例
Python 2.7の「等しくない演算子」のいくつかの例を見てみましょう。
$ python2.7
Python 2.7.10 (default, Aug 17 2018, 19:45:58)
[GCC 4.2.1 Compatible Apple LLVM 10.0.0 (clang-1000.0.42)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>> 10 <> 20
True
>>> 10 <> 10
False
>>> 10 != 20
True
>>> 10 != 10
False
>>> '10' != 10
True
>>>
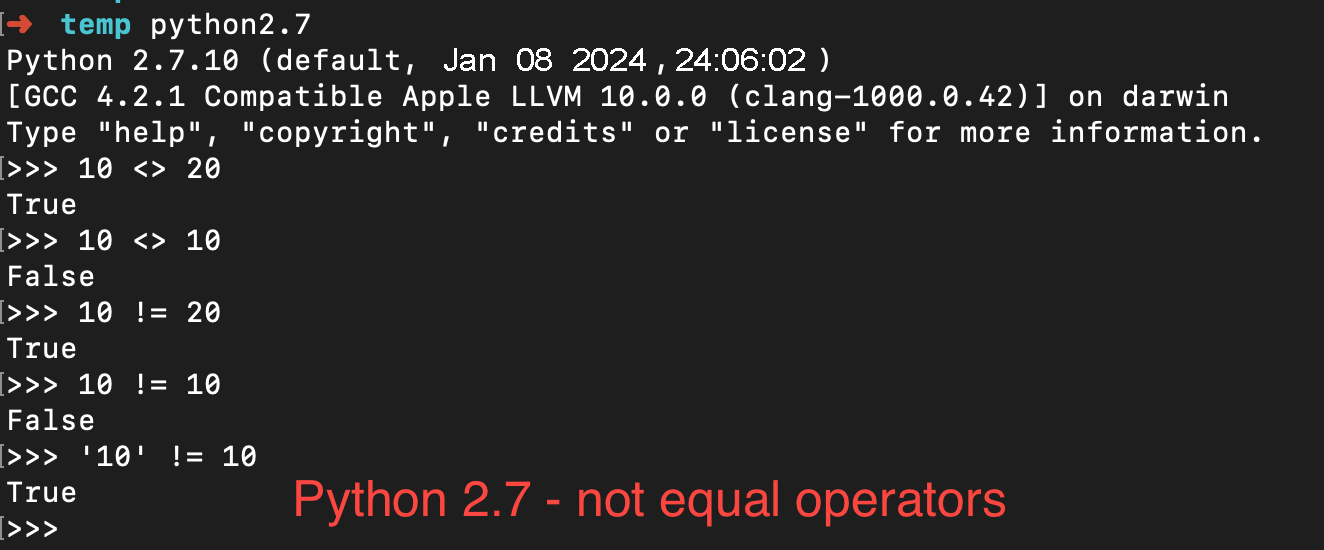
Python 3の例
Python 3 コンソールを使った例を以下に示します。
$ python3.7
Python 3.7.0 (v3.7.0:1bf9cc5093, Jun 26 2018, 23:26:24)
[Clang 6.0 (clang-600.0.57)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>> 10 <> 20
File "<stdin>", line 1
10 <> 20
^
SyntaxError: invalid syntax
>>> 10 != 20
True
>>> 10 != 10
False
>>> '10' != 10
True
>>>
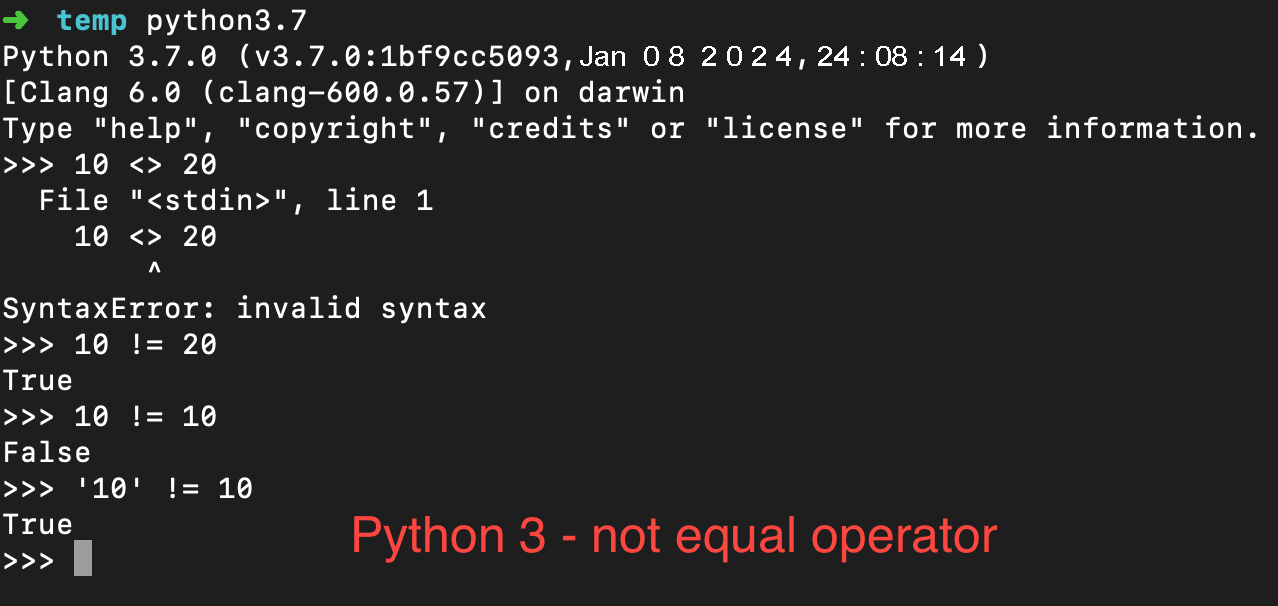
x = 10
y = 10
z = 20
print(f'x is not equal to y = {x!=y}')
flag = x != z
print(f'x is not equal to z = {flag}')
# python is strongly typed language
s = '10'
print(f'x is not equal to s = {x!=s}')
出力:
x is not equal to y = False
x is not equal to z = True
x is not equal to s = True
Pythonでは、カスタムオブジェクトとは異なるということです。
不等号演算子を使用すると、__ne__(self, other)関数が呼び出されます。したがって、オブジェクトに対してカスタムな実装を定義し、出力を変更することができます。例えば、idとrecordというフィールドを持つDataクラスがあるとします。不等号演算子を使用する場合、recordの値だけを比較したいとします。これは、独自の__ne__()関数を実装することで実現できます。
class Data:
id = 0
record = ''
def __init__(self, i, s):
self.id = i
self.record = s
def __ne__(self, other):
# return true if different types
if type(other) != type(self):
return True
if self.record != other.record:
return True
else:
return False
d1 = Data(1, 'Java')
d2 = Data(2, 'Java')
d3 = Data(3, 'Python')
print(d1 != d2)
print(d2 != d3)
出力:
False
True
d1とd2のレコードの値は同じですが、”id”が異なることに注意してください。__ne__()関数を削除すると、出力は次のようになります。
True
True
私たちのGitHubリポジトリから、完全なPythonスクリプトとその他のPythonの例をチェックアウトできます。