C++の文字列の配列の理解
みなさん、こんにちは!プログラマーとして、私たちはよくさまざまなデータ型の配列を扱います。今日の記事では、C++の文字列配列について取り上げます。
C++の文字列配列を宣言する方法
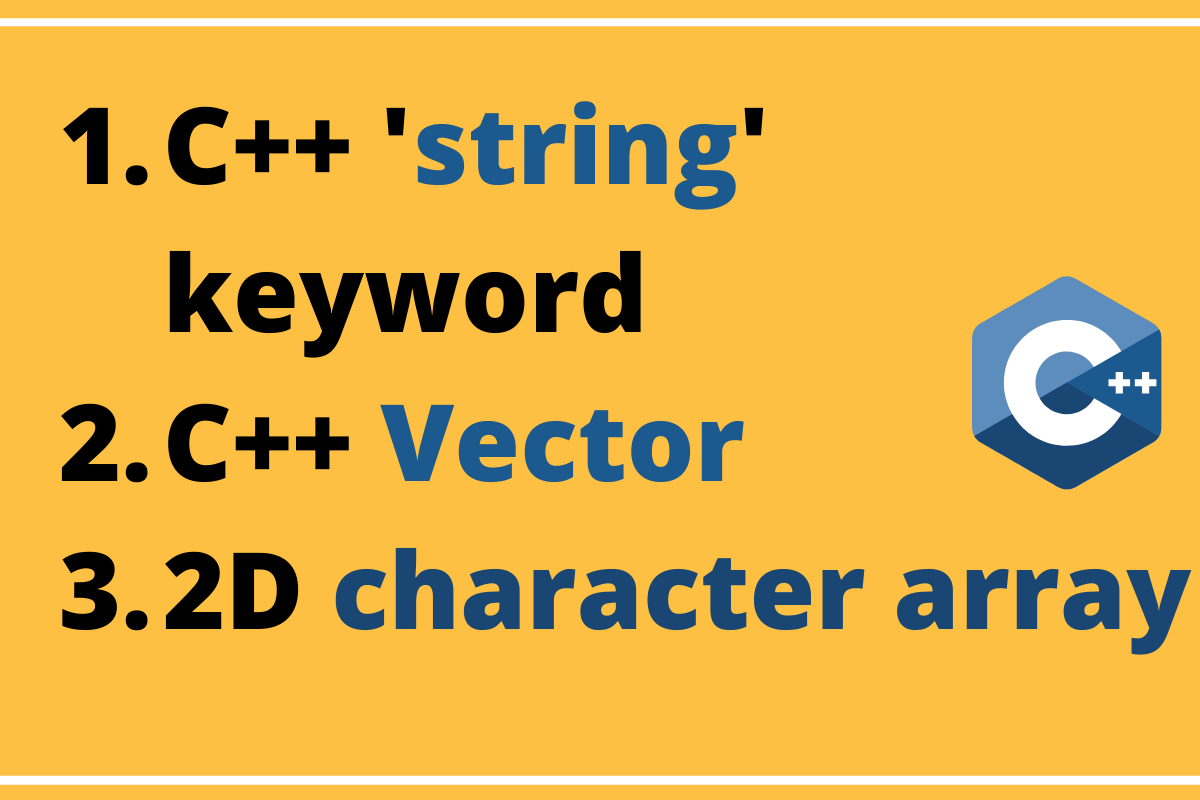
1. C++において、文字列配列を作成するためのStringキーワード
C++は、文字列配列のデータを宣言し、操作するために「string」というキーワードを提供しています。
「string」キーワードは、動的または実行時に配列の要素にメモリを割り当てます。それにより、データ要素の静的メモリ割り当ての手間を省くことができます。
文法: ‘string’ キーワードを使用して文字列の配列を宣言する
string array-name[size];
さらに、以下の構文を使用して文字列の配列を初期化することができます。
string array-name[size]={'val1','val2',.....,'valN'};
例えば1:
#include <bits/stdc++.h>
using namespace std;
int main()
{
string fruits[5] = { "Grapes", "Apple","Pineapple", "Banana", "Jackfruit" };
cout<<"String array:\n";
for (int x = 0; x< 5; x++)
cout << fruits[x] << "\n";
}
上記の例では、文字列の配列を初期化し、C++のループを使用して配列をトラバースし、文字列の配列に存在するデータ項目を出力しました。
出力:
String array:
Grapes
Apple
Pineapple
Banana
Jackfruit
例2:
「私は日本料理が大好きです。特に寿司が好きで、よく寿司屋に行きます。寿司の新鮮さや繊細な味わいが魅力で、いつでも食べたくなります。また、日本の食文化自体が興味深く、料理の技術や季節の食材にも魅了されます。日本料理は私にとって、心と体を満たしてくれる特別な存在です。」
#include <bits/stdc++.h>
using namespace std;
int main()
{
string arr[5];
cout<<"Enter the elements:"<<endl;
for(int x = 0; x<5;x++)
{
cin>>arr[x];
}
cout<<"\nString array:\n";
for (int x = 0; x< 5; x++)
cout << arr[x] << "\n";
}
皆さんが目撃できる通り、上記の例では、文字列配列のデータアイテムをコンソールから受け入れ、要素を出力しました。言い換えると、ユーザーからの入力を受け取り、文字列配列の要素を表示しました。
出力:
Enter the elements:
Jim
Nick
Daisy
Joha
Sam
String array:
Jim
Nick
Daisy
Joha
Sam
2. C++のSTLコンテナであるベクターを使用する。
C++標準テンプレートライブラリは、データを効率的に扱い、格納するためのコンテナを提供してくれます。
ベクターはそのようなコンテナーの一つであり、配列の要素を動的に保存します。そのため、C++のベクターは文字列の配列を作成し、同じものを簡単に操作するために使用できます。
文法:
The system checks the syntax of the entered code.
vector<string>array-name;
- The vector.push_back(element) method is used to add elements to the vector string array.
- The vector.size() method is used to calculate the length of the array i.e. the count of the elements input to the string array.
“I need to go to the grocery store to buy some fruits and vegetables.”
#include <bits/stdc++.h>
using namespace std;
int main()
{
vector<string> arr;
arr.push_back("Ace");
arr.push_back("King");
arr.push_back("Queen");
int size = arr.size();
cout<<"Elements of the vector array:"<<endl;
for (int x= 0; x< size; x++)
cout << arr[x] << "\n";
}
出力:
Elements of the vector array:
Ace
King
Queen
3.2次元の文字配列を使用する。 (San. Ni-jigen no moji hairetsu o shiyou suru.)
C++において、2次元配列は文字列の配列を表します。そのため、2次元char配列を使用して、文字列の要素を配列で表すことができます。
char配列は、静的またはコンパイル時に要素を作成し、保存します。つまり、要素の数やサイズは固定されます。
文法:
char array-name[number-of-items][maximun_size-of-string];
犬は私の親友です。
Inu wa watashi no shin’yū desu.
#include <bits/stdc++.h>
using namespace std;
int main()
{
char fruits[5][10] = { "Grapes", "Apple","Pineapple", "Banana", "Jackfruit" };
cout<<"Character array:\n";
for (int x = 0; x< 5; x++)
cout << fruits[x] << "\n";
}
上記のコードスニペットでは、文字列タイプの要素を格納するためにchar配列を作成しました。つまり、char配列[5][10]となります。ここでの5は文字列の要素の数を表し、10は入力文字列の最大サイズを指します。
出力:
Character array:
Grapes
Apple
Pineapple
Banana
Jackfruit
関数への引数としてのC++の文字列の配列
文字列の配列も、他の文字列以外のタイプの配列と同様に、関数に引数として渡すことができます。
文法的な構文 :
return-type function-name(string array-name[size])
{
// body of the function
}
「私の友達は大学で日本語を勉強しています。」
→ 「私の友達は大学で日本語を学んでいます。」
#include <iostream>
#include<string>
using namespace std;
void show(string arr[4]){
for(int x=0;x<4;x++)
{
cout<<arr[x]<<endl;
}
}
int main() {
string arr[4] = {"Jim", "Jeo", "Jio", "John"};
cout<<"Printing elements of the string array:"<<endl;
show(arr);
}
日本でのネイティブな言葉で次を言い換えてください。
出力:
Printing elements of the string array:
Jim
Jeo
Jio
John
結論
この記事では、文字列の配列を作成する方法と、それを関数で使用するためのテクニックについて理解しました。
参考文献
- C++ string array to a function
- Accessing elements from a string array in C++