スプリングのJdbcTemplateを使った例
Spring JdbcTemplateはSpring JDBCパッケージで最も重要なクラスです。
春のJdbcTemplate
- JDBC produces a lot of boiler plate code, such as opening/closing a connection to a database, handling sql exceptions etc. It makes the code extremely cumbersome and difficult to read.
- Implementing JDBC in the Spring Framework takes care of working with many low-level operations (opening/closing connections, executing SQL queries, etc.).
- Thanks to this, when working with the database in the Spring Framework, we only need to define the connection parameters from the database and register the SQL query, the rest of the work for us is performed by Spring.
- JDBC in Spring has several classes (several approaches) for interacting with the database. The most common of these is using the JdbcTemplate class. This is the base class that manages the processing of all events and database connections.
- The JdbcTemplate class executes SQL queries, iterates over the ResultSet, and retrieves the called values, updates the instructions and procedure calls, “catches” the exceptions, and translates them into the exceptions defined in the org.springframwork.dao package.
- Instances of the JdbcTemplate class are thread-safe. This means that by configuring a single instance of the JdbcTemplate class, we can then use it for several DAO objects.
- When using JdbcTemplate, most often, it is configured in the Spring configuration file. After that, it is implemented using bean in DAO classes.
Spring JdbcTemplateの例
「Spring JdbcTemplateの例題プログラムを見てみましょう。ここではPostgresqlデータベースを使用していますが、他のリレーショナルデータベース、例えばMySQLやOracleを利用することもできます。必要なのはデータベースの設定を変更するだけで、問題なく動作します。まずは実際に操作するためのサンプルデータが必要です。以下のSQLクエリを実行すると、テーブルが作成され、データが挿入されます。」
create table people (
id serial not null primary key,
first_name varchar(20) not null,
last_name varchar(20) not null,
age integer not null
);
insert into people (id, first_name, last_name, age) values
(1, 'Vlad', 'Boyarskiy', 21),
(2,'Oksi', ' Bahatskaya', 30),
(3,'Vadim', ' Vadimich', 32);
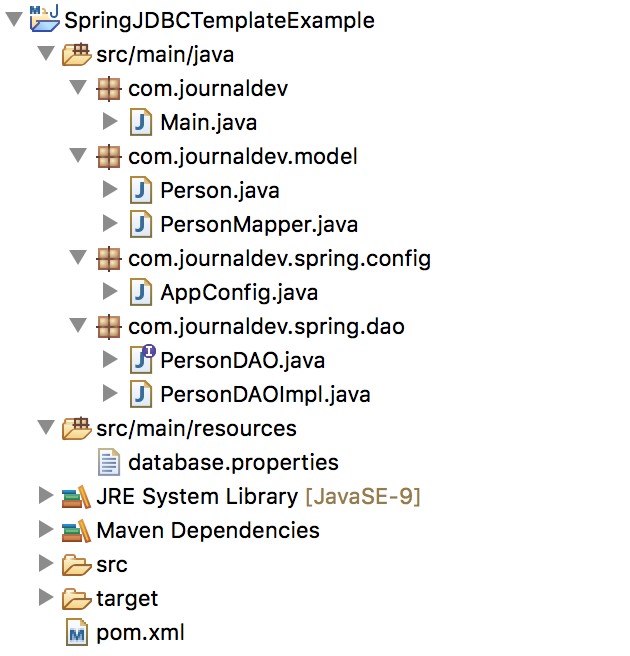
春のJDBC Mavenの依存関係
以下の依存関係が必要です- spring-core、spring-context、spring-jdbc、およびpostgresql。もし他のリレーショナルデータベース(例:MySQL)を使用している場合は、対応するJavaドライバの依存関係を追加してください。以下は私たちの最終的なpom.xmlファイルです。
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="https://maven.apache.org/POM/4.0.0" xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.scdev.spring</groupId>
<artifactId>JdbcTemplate</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<spring.framework>4.3.0.RELEASE</spring.framework>
<postgres.version>42.1.4</postgres.version>
</properties>
<dependencies>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>${postgres.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.framework}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.framework}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.framework}</version>
</dependency>
</dependencies>
</project>
Springのデータソースの設定
次のステップは、DataSourceビーンを定義するためにSpringの設定クラスを作成することです。私はJavaベースの設定を使用していますが、Springのビーン設定XMLファイルを使用することもできます。
package com.scdev.spring.config;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
import org.springframework.core.env.Environment;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
@Configuration
@ComponentScan("com.scdev.spring")
@PropertySource("classpath:database.properties")
public class AppConfig {
@Autowired
Environment environment;
private final String URL = "url";
private final String USER = "dbuser";
private final String DRIVER = "driver";
private final String PASSWORD = "dbpassword";
@Bean
DataSource dataSource() {
DriverManagerDataSource driverManagerDataSource = new DriverManagerDataSource();
driverManagerDataSource.setUrl(environment.getProperty(URL));
driverManagerDataSource.setUsername(environment.getProperty(USER));
driverManagerDataSource.setPassword(environment.getProperty(PASSWORD));
driverManagerDataSource.setDriverClassName(environment.getProperty(DRIVER));
return driverManagerDataSource;
}
}
- @Configuration – says that this class is configuration for Spring context.
- @ComponentScan(“com.scdev.spring”)- specifies the package to scan for component classes.
- @PropertySource(“classpath:database.properties”)- says that properties will be read from database.properties file.
以下は、database.propertiesファイルの内容です。
driver=org.postgresql.Driver
url=jdbc:postgresql://127.0.0.1:5432/school
dbuser=postgres
dbpassword=postgres
もしMySQLや他のリレーショナルデータベースを使用している場合は、上記の設定を適宜変更してください。
スプリングのJDBCモデルクラス
次のステップは、データベースのテーブルに対応するモデルクラスを作成することです。
package com.scdev.model;
public class Person {
private Long id;
private Integer age;
private String firstName;
private String lastName;
public Person() {
}
public Person(Long id, Integer age, String firstName, String lastName) {
this.id = id;
this.age = age;
this.firstName = firstName;
this.lastName = lastName;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@Override
public String toString() {
return "Person{" + "id=" + id + ", age=" + age + ", firstName='" + firstName + '\'' + ", lastName='" + lastName
+ '\'' + '}';
}
}
データベースからデータを取得するためには、インターフェースRowMapperを実装する必要があります。このインターフェースには、mapRowという1つのメソッドがあります。このメソッドは、ResultSet resultSetとint iを引数に受け取り、モデルクラス(例:Person)の1つのインスタンスを返します。
package com.scdev.model;
import java.sql.ResultSet;
import java.sql.SQLException;
import org.springframework.jdbc.core.RowMapper;
public class PersonMapper implements RowMapper<Person> {
public Person mapRow(ResultSet resultSet, int i) throws SQLException {
Person person = new Person();
person.setId(resultSet.getLong("id"));
person.setFirstName(resultSet.getString("first_name"));
person.setLastName(resultSet.getString("last_name"));
person.setAge(resultSet.getInt("age"));
return person;
}
}
Spring JDBC DAO クラス
最後のステップは、モデルクラスをデータベーステーブルにマッピングするためのDAOクラスを作成し、SQLクエリを使用します。また、@Autowiredアノテーションを使用してDataSourceを設定し、いくつかのAPIを公開します。
package com.scdev.spring.dao;
import java.util.List;
import com.scdev.model.Person;
public interface PersonDAO {
Person getPersonById(Long id);
List<Person> getAllPersons();
boolean deletePerson(Person person);
boolean updatePerson(Person person);
boolean createPerson(Person person);
}
package com.scdev.spring.dao;
import java.util.List;
import javax.sql.DataSource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
import com.scdev.model.Person;
import com.scdev.model.PersonMapper;
@Component
public class PersonDAOImpl implements PersonDAO {
JdbcTemplate jdbcTemplate;
private final String SQL_FIND_PERSON = "select * from people where id = ?";
private final String SQL_DELETE_PERSON = "delete from people where id = ?";
private final String SQL_UPDATE_PERSON = "update people set first_name = ?, last_name = ?, age = ? where id = ?";
private final String SQL_GET_ALL = "select * from people";
private final String SQL_INSERT_PERSON = "insert into people(id, first_name, last_name, age) values(?,?,?,?)";
@Autowired
public PersonDAOImpl(DataSource dataSource) {
jdbcTemplate = new JdbcTemplate(dataSource);
}
public Person getPersonById(Long id) {
return jdbcTemplate.queryForObject(SQL_FIND_PERSON, new Object[] { id }, new PersonMapper());
}
public List<Person> getAllPersons() {
return jdbcTemplate.query(SQL_GET_ALL, new PersonMapper());
}
public boolean deletePerson(Person person) {
return jdbcTemplate.update(SQL_DELETE_PERSON, person.getId()) > 0;
}
public boolean updatePerson(Person person) {
return jdbcTemplate.update(SQL_UPDATE_PERSON, person.getFirstName(), person.getLastName(), person.getAge(),
person.getId()) > 0;
}
public boolean createPerson(Person person) {
return jdbcTemplate.update(SQL_INSERT_PERSON, person.getId(), person.getFirstName(), person.getLastName(),
person.getAge()) > 0;
}
}
PersonDAOImplクラスは@Componentアノテーションで注釈が付けられており、このクラス内にはJdbcTemplate型のフィールドがあります。このクラスのコンストラクタが呼び出されると、DataSourceのインスタンスが注入され、JdbcTemplateのインスタンスを作成することができます。その後、このインスタンスをメソッド内で使用することができます。
スプリングのJdbcTemplateのテストプログラム
私たちのSpring JdbcTemplateのサンプルプロジェクトは準備が整いましたので、テストクラスで試しましょう。
package com.scdev;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import com.scdev.model.Person;
import com.scdev.spring.config.AppConfig;
import com.scdev.spring.dao.PersonDAO;
public class Main {
public static void main(String[] args) {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
PersonDAO personDAO = context.getBean(PersonDAO.class);
System.out.println("List of person is:");
for (Person p : personDAO.getAllPersons()) {
System.out.println(p);
}
System.out.println("\nGet person with ID 2");
Person personById = personDAO.getPersonById(2L);
System.out.println(personById);
System.out.println("\nCreating person: ");
Person person = new Person(4L, 36, "Sergey", "Emets");
System.out.println(person);
personDAO.createPerson(person);
System.out.println("\nList of person is:");
for (Person p : personDAO.getAllPersons()) {
System.out.println(p);
}
System.out.println("\nDeleting person with ID 2");
personDAO.deletePerson(personById);
System.out.println("\nUpdate person with ID 4");
Person pperson = personDAO.getPersonById(4L);
pperson.setLastName("CHANGED");
personDAO.updatePerson(pperson);
System.out.println("\nList of person is:");
for (Person p : personDAO.getAllPersons()) {
System.out.println(p);
}
context.close();
}
}
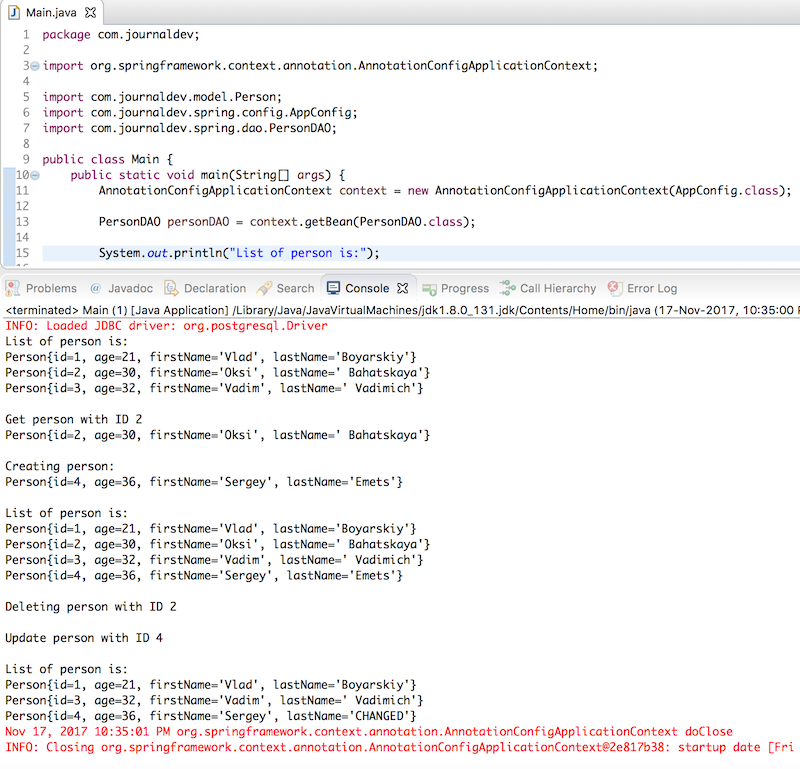
「Spring JdbcTemplateのサンプルプロジェクトをダウンロードしてください。」
参照:API ドキュメント