「C++におけるexit()関数」
はじめに
今日はC++のexit()について学びます。C++では、組み込みのブレイク関数を使ってループから抜けることができることを知っています。同様に、exit()関数を使用すると、C++プログラム全体からも抜けることができます。
プログラムの中で結論を出さなければならない状況を考えてみてください。プログラム全体がコンパイルや評価される前に結果を得て、何かを結論します。
必要な情報や結果を得たら、プログラムをすぐに終了する方法は何ですか?
上記の質問に対する答えは、C++の組み込みのexit()関数を使用することです。では、この関数とその動作について詳しく見てみましょう。
C++におけるexit()関数の定義
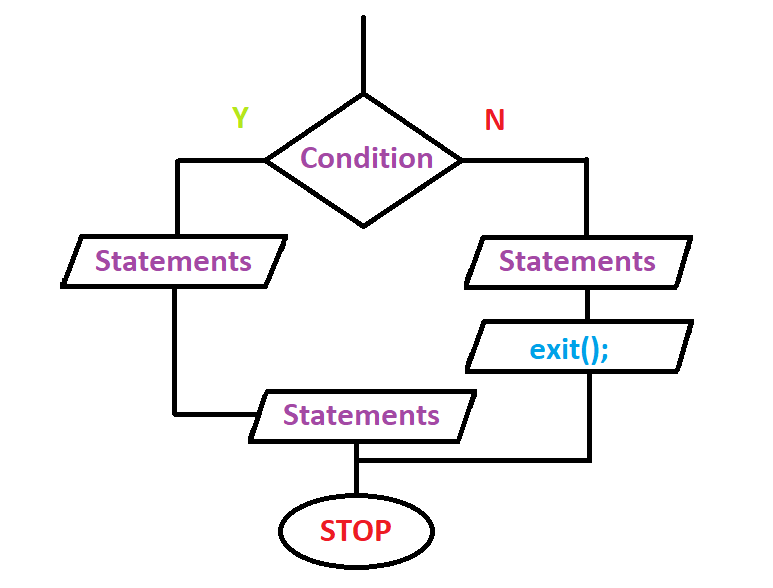
理論的には、C++のexit()関数は、プログラムリスト内のどこに現れてもすぐにプログラムを終了させます。この関数は、stdlib.hのヘッダーファイル内で定義されており、exit()関数を使用する際にはこのファイルを含める必要があります。
C++におけるexit()関数の構文
exit()関数を使用するための文法は以下の通りです。
exit( exit_value );
成功的にプログラムが終了した後、exit_valueは操作システムに渡される値です。この値は、ERROR LEVELがexit()関数によって返される返り値を示すバッチファイルでテストすることができます。一般的に、値0は正常な終了を示し、他の数値はエラーを示します。
C++におけるexit()関数の動作
覚えておいてください、exit()関数は常に値を返しません。それはプロセスを終了し、終了プログラムの通常のクリーンアップを行います。
また、C++でこの関数を呼び出しても自動的な記憶オブジェクトは破棄されません。
以下の例を注意深く見てください。
#include<iostream>
using namespace std;
int main()
{
int i;
cout<<"Enter a non-zero value: "; //user input
cin>>i;
if(i) // checks whether the user input is non-zero or not
{
cout<<"Valid input.\n";
}
else
{
cout<<"ERROR!"; //the program exists if the value is 0
exit(0);
}
cout<<"The input was : "<<i;
}
出力:
Enter a non-zero value: 0
ERROR!
- Since the user input for the above code provided is zero(0), the else part is executed for the if-else statement. Further where the compiler encounters the exit() function and terminates the program.
- Even the print statement below the if-else is not executed since the program has been terminated by the exit() function already.
では、別の例を見てみましょう。数が素数かどうかを判定しようとする場合です。
C++のexit()関数を使用する
以下のプログラムは、exit() 関数の使用例を示しています。
#include<iostream>
using namespace std;
int main()
{
int i,num;
cout<<"Enter the number : ";
cin>>num;
for(i=2;i<=num/2;i++)
{
if(num%i==0)
{
cout<<"\nNot a prime number!";
exit(0);
}
}
cout<<"\nIt is a prime number!";
return 0;
}
出力:
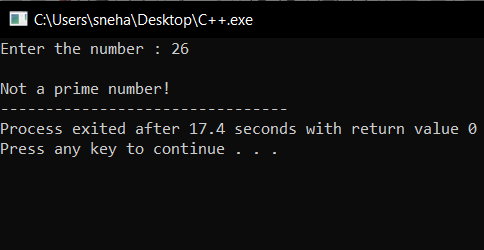
上記のコードについてさらに述べると、
- firstly we took a user input for the number. We need to check whether this number,num is prime or not.
- After that, we apply a for loop which works from 2 to n/2. This is because we already know that all numbers are divisible by 1 as well as a number is indivisible by numbers above its half.
- Inside the for loop, we check whether the number is divisible by the loop iterator at that instant. If so, we can directly conclude that the number is not prime and terminate the program using the exit() function,
- Else, the loop goes on checking. After the execution of the whole loop structure, we declare the number as a prime one.
結論は次のように言えます。
このチュートリアルでは、C++の組み込みのexit()関数の動作と使用方法について説明しました。これはプログラムの実行を終了するために広く使われています。
質問があれば、下にコメントしてください。
参考資料
- https://stackoverflow.com/questions/461449/return-statement-vs-exit-in-main
- /community/tutorials/c-plus-plus