Spring Component annotation
The Spring Component annotation designates a class as a Component. This implies that the Spring framework will automatically detect these classes for dependency injection when utilizing annotation-based configuration and classpath scanning.
Spring Component -> Component in Spring framework.
Simply put, a Component is accountable for certain tasks. The Spring framework offers three distinct annotations that can be employed to designate a class as a Component.
-
- Service: Indicates that the class offers certain services. Our utility classes can be designated as Service classes.
-
-
-
- Repository: This annotation signifies that the class manages CRUD operations, typically used with
-
- implementations handling database tables.
-
- Controller: Primarily employed in web applications or REST web services to declare that the class serves as a front controller, responsible for managing user requests and providing suitable responses.
Please keep in mind that all of these four annotations are present in the org.springframework.stereotype package and are included in the spring-context jar. In most cases, our component classes will belong to one of these three specific annotations, which means that the @Component annotation may not be used frequently.
An instance of a Spring component as an example.
We can create a basic Maven application using Spring framework to highlight the usage of Spring Component annotation and how Spring automatically detects it through annotation-based configuration and classpath scanning. To begin, generate a Maven project and include the specified spring core dependency accordingly.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.6.RELEASE</version>
</dependency>
To acquire the core features of the spring framework, all we require is to create a basic component class and designate it with the @Component annotation.
package com.scdev.spring;
import org.springframework.stereotype.Component;
@Component
public class MathComponent {
public int add(int x, int y) {
return x + y;
}
}
We are now able to establish a spring context based on annotations and retrieve the MathComponent bean from it.
package com.scdev.spring;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class SpringMainClass {
public static void main(String[] args) {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext();
context.scan("com.scdev.spring");
context.refresh();
MathComponent ms = context.getBean(MathComponent.class);
int result = ms.add(1, 2);
System.out.println("Addition of 1 and 2 = " + result);
context.close();
}
}
Simply execute the aforementioned class as a regular java application and you will receive the subsequent output in the console.
Jun 05, 2018 12:49:26 PM org.springframework.context.support.AbstractApplicationContext prepareRefresh
INFO: Refreshing org.springframework.context.annotation.AnnotationConfigApplicationContext@ff5b51f: startup date [Tue Jun 05 12:49:26 IST 2018]; root of context hierarchy
Addition of 1 and 2 = 3
Jun 05, 2018 12:49:26 PM org.springframework.context.support.AbstractApplicationContext doClose
INFO: Closing org.springframework.context.annotation.AnnotationConfigApplicationContext@ff5b51f: startup date [Tue Jun 05 12:49:26 IST 2018]; root of context hierarchy
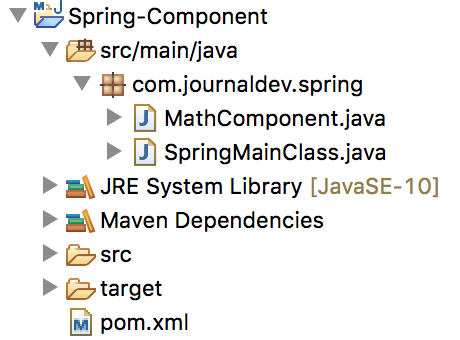
@Component("mc")
public class MathComponent {
}
MathComponent ms = (MathComponent) context.getBean("mc");
Even though I applied the @Component annotation to MathComponent, it should actually be labeled as a service class with the @Service annotation. The outcome will remain unchanged.
You have the option to retrieve the project from our GitHub Repository.
Source: API Documentation
Paraphrased: Documentation on Application Programming Interface
more tutorials
React Application Component Testing Integrate with Playwright(Opens in a new browser tab)
Spring MVC HandlerInterceptorAdapter and HandlerInterceptor.(Opens in a new browser tab)