How can I convert a Java String into a double data type?
There are several ways to convert a Java String to a double value. In this article, we will explore some commonly used methods for converting a Java String to either a double primitive type or a Double object. It is important to note that Java’s support for autoboxing allows for seamless interchangeability between the double primitive type and the Double object.
Double d1 = 10.25d;
//autoboxing from double to Double
double d = Double.valueOf(10.25);
//unboxing from Double to double
How to convert a String to a Double in Java.
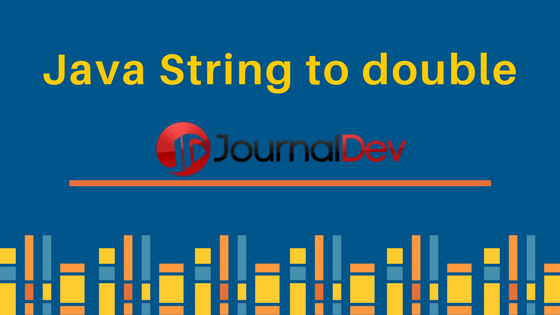
-
- Double.parseDouble()
-
- The parseDouble() method can be used to convert a String to a double. The String can start with “-” to indicate a negative number or “+” to indicate a positive number. Trailing 0s are removed from the double value. The identifier “d” can also be used to indicate that the String is a double value. This method returns a double primitive type. The code snippet below demonstrates how to convert a String to a double using Double.parseDouble():
-
- String str = “+123.4500d”;
-
- double d = Double.parseDouble(str); // returns double primitive
-
- System.out.println(d); //-123.45, trailing 0s are removed
System.out.println(Double.parseDouble(“123.45001”)); //123.45001
System.out.println(Double.parseDouble(“123.45001d”)); //123.45001
System.out.println(Double.parseDouble(“123.45000”)); //123.45
System.out.println(Double.parseDouble(“123.45001D”)); //123.45001
Double.valueOf()
This method works in a similar way to the parseDouble() method, but it returns a Double object. The code snippet below shows how to use this method to convert a String to a Double object:
String str = “123.45”;
Double d = Double.valueOf(str); // returns Double object
System.out.println(d); //123.45
System.out.println(Double.valueOf(“123.45d”)); //123.45
System.out.println(Double.valueOf(“123.4500d”)); //123.45
System.out.println(Double.valueOf(“123.45D”)); //123.45
new Double(String s)
We can also convert a String to a Double object using its constructor. If we want a double primitive type, we can use the doubleValue() method on it. Note that this constructor has been deprecated in Java 9 and it is recommended to use the parseDouble() or valueOf() methods instead. The code snippet below demonstrates how to convert a String to a Double object using its constructor:
String str = “98.7”;
double d = new Double(str).doubleValue(); //constructor deprecated in java 9
System.out.println(d); //98.7
DecimalFormat parse()
This method is useful for parsing a formatted string to a double. For example, if the String is “1,11,111.23d”, we can use DecimalFormat to parse this string to a double as shown below:
String str = “1,11,111.23d”;
try {
double l = DecimalFormat.getNumberInstance().parse(str).doubleValue();
System.out.println(l); //111111.23
} catch (ParseException e) {
e.printStackTrace();
}
Note that the parse() method returns an instance of Number, so we are calling doubleValue() to get the double primitive type from it. This method also throws a ParseException if the string is not properly formatted.
The above information concludes the process of converting a string to a double in a Java program. You can refer to the Double API documentation for more details.
More tutorials
Comprehending Java’s Data Types(Opens in a new browser tab)
Converting string to array in the Java programming language(Opens in a new browser tab)
Addition Assignment Operator mean in Java(Opens in a new browser tab)
Java’s Random Number Generator(Opens in a new browser tab)
The Python functions ord() and chr()(Opens in a new browser tab)