Example of how to use RestTemplate in Spring
Spring RestTemplate offers a convenient method to conduct testing on RESTful web services.
The RestTemplate for Spring.
- Spring RestTemplate class is part of spring-web, introduced in Spring 3.
- We can use RestTemplate to test HTTP based restful web services, it doesn’t support HTTPS protocol.
- RestTemplate class provides overloaded methods for different HTTP methods, such as GET, POST, PUT, DELETE etc.
Example of using Spring RestTemplate
Let’s examine an example of Spring’s RestTemplate, where we will be testing REST web services from the previous Spring Data JPA article. The table below displays the URIs that are supported by this REST web service.
URI | HTTP Method | Description |
---|---|---|
/springData/person | GET | Get all persons from database |
/springData/person/{id} | GET | Get person by id |
/springData/person | POST | Add person to database |
/springData/person | PUT | Update person |
/springData/person/{id} | DELETE | Delete person by id |
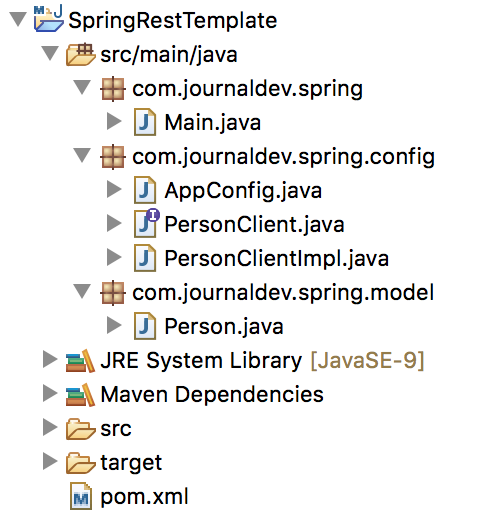
Dependencies for Maven for Spring RestTemplate.
To utilize the spring framework, we require dependencies like spring-core and spring-context. Furthermore, for the RestTemplate class, we need the spring-web artifact. Additionally, to enable Spring’s JSON support via the Jackson API, we need jackson-mapper-asl.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="https://maven.apache.org/POM/4.0.0" xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.scdev.spring</groupId>
<artifactId>SpringRestTemplate</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<spring.framework>4.3.0.RELEASE</spring.framework>
<spring.web>3.0.2.RELEASE</spring.web>
<serializer.version>2.8.1</serializer.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.framework}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.framework}</version>
</dependency>
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-mapper-asl</artifactId>
<version>1.9.4</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring.web}</version>
</dependency>
</dependencies>
</project>
Class for configuring Spring.
In order to configure the RestTemplate class, we need to create a spring bean which is accomplished within the AppConfig class.
package com.scdev.spring.config;
import org.codehaus.jackson.map.ObjectMapper;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.converter.json.MappingJacksonHttpMessageConverter;
import org.springframework.web.client.RestTemplate;
@Configuration
@ComponentScan("com.scdev.spring")
public class AppConfig {
@Bean
RestTemplate restTemplate() {
RestTemplate restTemplate = new RestTemplate();
MappingJacksonHttpMessageConverter converter = new MappingJacksonHttpMessageConverter();
converter.setObjectMapper(new ObjectMapper());
restTemplate.getMessageConverters().add(converter);
return restTemplate;
}
}
Please be aware that RestTamplate utilizes a MessageConverter and we must configure this property in the RestTemplate bean. In the given instance, we are using MappingJacksonHttpMessageConverter to retrieve data in the JSON format.
Class for a specific model
To convert the JSON received from our web service to a java object using the jackson mapper, it is necessary to develop a model class specifically for this purpose. It should be noted that this model class will closely resemble the one used in the web service, with the exception that JPA annotations are not required in this case.
package com.scdev.spring.model;
public class Person {
private Long id;
private Integer age;
private String firstName;
private String lastName;
public Person() {
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@Override
public String toString() {
return "Person{" + "id=" + id + ", age=" + age + ", firstName='" + firstName + '\'' + ", lastName='" + lastName
+ '\'' + '}';
}
}
The Class for the RestTemplate Client in Spring.
The last task is to generate the client classes which will utilize the previously defined RestTemplate bean.
package com.scdev.spring.config;
import java.util.List;
import org.springframework.http.HttpStatus;
import com.scdev.spring.model.Person;
public interface PersonClient {
List<Person> getAllPerson();
Person getById(Long id);
HttpStatus addPerson(Person person);
void updatePerson(Person person);
void deletePerson(Long id);
}
package com.scdev.spring.config;
import java.util.Arrays;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
import com.scdev.spring.model.Person;
@Service
public class PersonClientImpl implements PersonClient {
@Autowired
RestTemplate restTemplate;
final String ROOT_URI = "https://localhost:8080/springData/person";
public List<Person> getAllPerson() {
ResponseEntity<Person[]> response = restTemplate.getForEntity(ROOT_URI, Person[].class);
return Arrays.asList(response.getBody());
}
public Person getById(Long id) {
ResponseEntity<Person> response = restTemplate.getForEntity(ROOT_URI + "/"+id, Person.class);
return response.getBody();
}
public HttpStatus addPerson(Person person) {
ResponseEntity<HttpStatus> response = restTemplate.postForEntity(ROOT_URI, person, HttpStatus.class);
return response.getBody();
}
public void updatePerson(Person person) {
restTemplate.put(ROOT_URI, person);
}
public void deletePerson(Long id) {
restTemplate.delete(ROOT_URI + id);
}
}
We invoke RestTemplate methods by considering the URI and the HTTP method, and we pass the suitable request object if necessary. The code is easily comprehensible.
TestClass for testing Spring RestTemplate.
Now is the moment to evaluate our Spring RestTemplate example project. The following class demonstrates the utilization of RestTemplate methods in a Spring manner.
package com.scdev.spring;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.http.HttpStatus;
import com.scdev.spring.config.AppConfig;
import com.scdev.spring.config.PersonClient;
import com.scdev.spring.model.Person;
public class Main {
public static void main(String[] args) {
AnnotationConfigApplicationContext applicationContext = new AnnotationConfigApplicationContext(AppConfig.class);
PersonClient client = applicationContext.getBean(PersonClient.class);
System.out.println("Getting list of all people:");
for (Person p : client.getAllPerson()) {
System.out.println(p);
}
System.out.println("\nGetting person with ID 2");
Person personById = client.getById(2L);
System.out.println(personById);
System.out.println("Adding a Person");
Person p = new Person();
p.setAge(50);
p.setFirstName("David");
p.setLastName("Blain");
HttpStatus status = client.addPerson(p);
System.out.println("Add Person Response = " + status);
applicationContext.close();
}
}
Once I execute the program on my local configuration, I receive the ensuing output.
Getting list of all people:
Person{id=2, age=30, firstName='Oksi', lastName=' Bahatskaya'}
Person{id=1, age=30, firstName='Vlad', lastName='Mateo'}
Getting person with ID 2
Person{id=2, age=30, firstName='Oksi', lastName=' Bahatskaya'}
Adding a Person
Add Person Response = 201
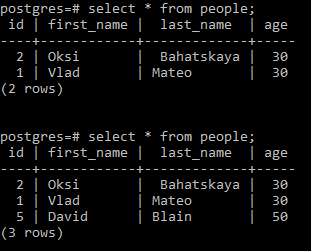
Get the Spring RestTemplate Example Project for download.
Source: API Documentation
More tutorials
Spring MVC Controller(Opens in a new browser tab)
Dependency Injection in Spring(Opens in a new browser tab)
The Spring Framework(Opens in a new browser tab)
Spring WebFlux the Spring Reactive Programming(Opens in a new browser tab)
Spring Component annotation(Opens in a new browser tab)