Java Tutorial for beginners
Greetings, and thank you for visiting the Core Java Tutorial. Throughout my writing career, I have extensively covered topics on Core Java and Java EE frameworks. Due to popular demand, I received numerous emails requesting an index post specifically for the Core Java tutorial. This way, beginners can easily navigate through the posts and efficiently grasp the concepts of Core Java programming. Luckily, I finally found the time to fulfill this request, and I have compiled a comprehensive list of Core Java tutorial-related posts that I believe will expedite your learning journey. This list is up to date with Java-10, and I will continue to update it with the latest developments in Java-11 and future versions.
Java Tutorial for Beginners
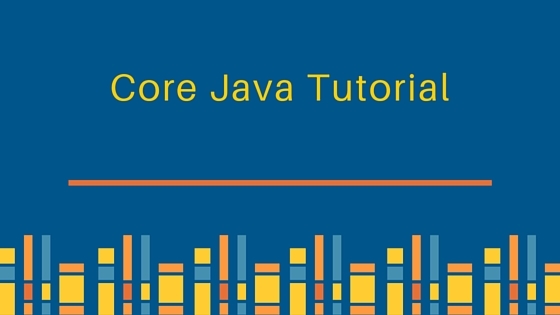
Getting Started with the Core Java Tutorial.
-
- Setting up Java on a Windows operating system
-
- Creating your initial Java application
-
- Using a Java method
-
- Java constructor
-
- Java Access Modifiers: public, protected, private, and default
-
- Looping in Java with a for loop
-
- Looping in Java with a while loop
-
- Looping in Java with a do-while loop
-
- Understanding the Java static keyword
-
- Using the Java break keyword
- Utilizing the Java continue keyword
Tutorial on the foundational Java programming language – Object-oriented principles.
-
- The concept of OOPS
-
- Java Composition
-
- Java Inheritance
-
- Comparison of Composition and Inheritance in Java
- Nested Classes in Java
Tutorial on Core Java – Types of Data and Operators
-
- Java Data Types, Primitives, and Binary Literals – Java’s classifications of data, the fundamental building blocks, and syntax for binary representation.
-
- Java Autoboxing and Unboxing – Java’s ability to automatically convert between primitive and wrapper types.
-
- Java Wrapper Classes – The classes in Java that provide objects corresponding to primitive types.
- Java Ternary Operator – Java’s conditional operator that evaluates an expression, providing a concise way to write if-else statements.
Java Tutorial: Interface and Abstract Class
-
- Java’s abstract class is a class that cannot be instantiated and serves as a blueprint for other classes. Meanwhile, a Java interface defines a contract that a class must adhere to by specifying methods that must be implemented.
- The dissimilarity between an abstract class and an interface in Java lies in their nature and usage within the language.
Java Tutorial – Manipulating Strings
-
- What is the reason behind String being both immutable and final?
-
- Exploring the concept of Java String Pool
-
- Demonstration of Java String subsequence
-
- Example of Java String compareTo
-
- Illustration of Java String substring
-
- Conversion between String and char types
-
- Example of splitting a String in Java
-
- Converting between String and byte array
-
- Converting String to char array
-
- Exploring String concatenation in Java
-
- Comparison of String, StringBuffer, and StringBuilder in Java
- Examples of String programs in Java
Java Tutorial on Arrays – Primitive, Objects, and Multidimensional Arrays.
-
- Java Array Initialization
-
- Java 2D Array
-
- Array of ArrayList in Java
-
- Example of converting String to String Array
-
- Explanation of Java Variable Arguments
-
- Adding elements to a Java Array
-
- Java Array Sorting
-
- Converting Java String Array to String
-
- Converting Java ArrayList to Array
-
- Converting Array to ArrayList in Java
- Methods for copying arrays in Java
Tutorial for Core Java – Annotation and Enum.
-
- Tutorial on Java Annotations
-
- Annotation for overriding in Java
- Tutorial on using enums in Java
Java Core Tutorial – Collections and Generics
-
- Tutorial on the Java Collections Framework which includes the following topics:
– Java List, Java ArrayList, and Java LinkedList
– Java Set, Java HashSet, and Java TreeSet
– Java Map, Java HashMap, and Java TreeMap
– Java Queue, Java Stack, and Java Iterator
– Java ListIterator and Java PriorityQueue Example
– Priority Queue in Java
– Comparison between ArrayList and CopyOnWriteArrayList
– How to prevent ConcurrentModificationException when using an Iterator
– Tutorial on Java Generics Example.
Java Tutorial for Input/Output Operations
-
- Java: Creating a New File
-
- Deleting a File in Java
-
- Using File Separators in Java
-
- Recursively Deleting a Directory in Java
-
- Renaming and Moving a File in Java
-
- Getting the Size of a File in Java
-
- Getting the File Extension in Java
-
- Checking if a File Exists in Java
-
- Checking if a File is a Directory in Java
-
- Getting the Last Modified Date of a File in Java
-
- Listing Specific Files using FileNameFilter in Java
-
- Understanding Java File Path, Absolute Path, and Canonical Path
-
- Setting File Permissions in Java
-
- Copying a File in Java: 4 Methods
-
- Reading a File in Java using BufferedReader, Scanner, and Files
-
- Understanding the Java Scanner Class
-
- Opening a File in Java
-
- Reading a File into a String in Java
-
- Reading a File Line by Line in Java
-
- Writing to a File in Java
-
- Appending Data to a File in Java
-
- Converting an InputStream to a File in Java
-
- Example of Java Random Access File
-
- Downloading a File from a URL in Java
-
- Example of Java GZip Compression
-
- Working with Temporary Files in Java
-
- Reading a CSV File using the Java Scanner Class
- Example of Java Property File
A tutorial on handling exceptions in Core Java.
- Java has a mechanism called exception handling to deal with situations like java.lang.NoSuchMethodError and java.lang.NullPointerException.
Native Paraphrase: MultiThreading and Concurrency in Core Java Tutorial.
-
- Java Threads:
-
- – Java Thread Lifecycle
-
- – Example of Sleeping a Thread
-
- – Example of Joining Threads
-
- – Example of using wait, notify, and notifyAll with Threads
-
- – Ensuring Thread Safety with Synchronization in Java
-
- – Example of using ThreadLocal in Java
-
- – Example of using Timer and TimerTask in Java
-
- – Example of using Thread Pool in Java
-
- – Example of using Callable and Future in Java
-
- – Example of using FutureTask in Java
-
- – Example of using ScheduledThreadPoolExecutor in Java
- – Example of using Lock in Java
Java Tutorial for Regular Expressions
-
- Example tutorial for Regular Expressions in Java
-
- Using Regular Expressions to validate an Email Address in Java
- Using Regular Expressions to validate a Phone Number in Java
The Reflection API in Java that forms its foundation.
- Tutorial on the Java Reflection API
Java 7 refers to the seventh version of the Java programming language.
-
- Switch case with a string
-
- Java ARM – Using try with resources
-
- Using binary literals in Java
-
- Using underscores in numeric literals
-
- Handling multiple exceptions in a single catch block
- Example of using Java PosixFilePermission to set file permissions
One possible paraphrase of “Java 8” could be “Version 8 of the Java programming language.”
-
- Overview of Java 8 Features
-
- Changes in Java 8 Interfaces
-
- Introduction to Lambda Expressions in Java
-
- Exploring the Stream API in Java
-
- Tutorial on Java Date Time API
- Understanding Java Spliterator
Version 9 of the Java programming language.
- Java 9 introduces several new features, including private methods in interfaces, improvements in the try-with-resources statement, enhancements to the Optional class, advancements in the Stream API, a new “var” keyword for local variables, changes to the underscore character, factory methods for creating immutable lists, sets, and maps, the introduction of modules, and guides on developing Java modules using different tools such as Command Prompt, Eclipse, and IntelliJ IDEA.
One possible paraphrase could be:
“The tenth version of Java.”
- Java 10 encompasses characteristics like Local Variable Type Inference.
The eleventh version of Java.
- Java 11 includes several new features, one of which is the addition of six new methods to the String Class.
Advanced Topics in Core Java Tutorial.
-
- Java Heap Memory and Stack Memory
-
- – The memory in Java is divided into the Heap Memory and Stack Memory.
Java is Pass by Value and not Pass by Reference
– In Java, the method of passing values is done by value rather than by reference.
JVM Memory Model and Garbage Collection
– The memory model of the Java Virtual Machine (JVM) and the process of garbage collection.
Serialization in Java
– The process of converting Java objects into a serialized format, which can be stored or transmitted.
Java System Class
– The Java System Class, which provides methods and properties related to the system’s environment.
Internationalization (i18n) in Java
– The concept of internationalization in Java, abbreviated as i18n, that allows software to adapt to various languages and cultures.
Atomic Operations in Java
– Operations in Java that are performed atomically, meaning they are executed as a single, indivisible unit.
Thread Dump in Java
– The collection of information about the threads running in a Java program at a specific point in time.
Deadlocks in Java
– Situations in Java where two or more threads are unable to proceed because each is waiting for a resource held by another.
Sorting Objects in Java
– The process of arranging objects in a specific order based on a specified criterion in Java.
Understanding JDK, JRE and JVM
– Gaining comprehension of the Java Development Kit (JDK), Java Runtime Environment (JRE), and Java Virtual Machine (JVM).
Java Classloader Example Tutorial
– A tutorial that demonstrates an example of how class loading works in Java.
Java clone object
– The process of creating a copy of an object in Java.
If you find that I have overlooked anything in this comprehensive core Java tutorial, please feel free to let me know in the comments. I will gladly write about it and include it in the list. Source: Oracle JavaSE Tutorial.
more tutorials
Tutorials on Java EE(Opens in a new browser tab)
Tutorial on Java Server Faces (JSF)(Opens in a new browser tab)
The Spring Framework(Opens in a new browser tab)
strsplit function in R(Opens in a new browser tab)
convert string to character array in Java.(Opens in a new browser tab)